How to Use of Inline Functions in Kotlin
-
Kotlin
inline
Functions -
Use Kotlin
inline
Functions With Reified Type Parameters -
Use
noinline
in Kotlininline
Functions
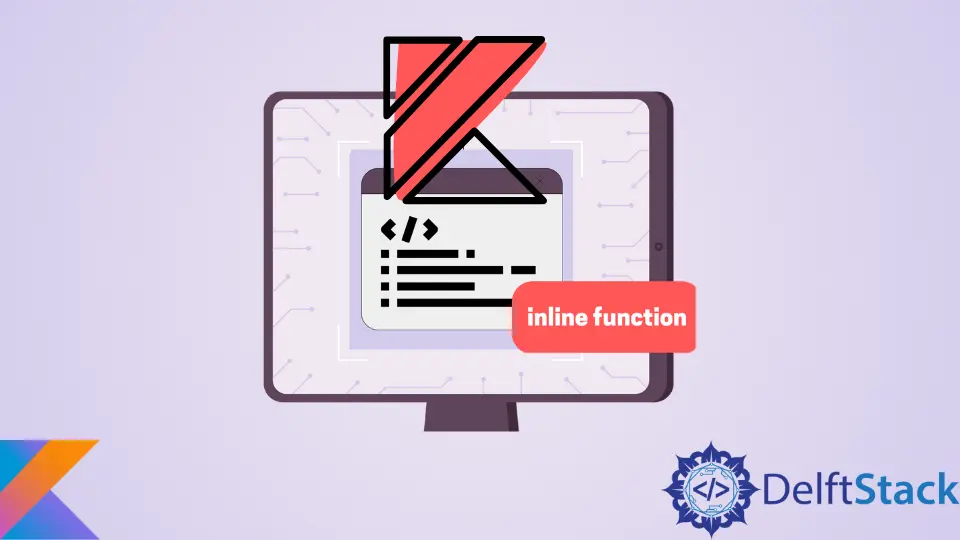
Most Kotlin projects use high-order functions, which can be problematic for your Kotlin projects. Each high-order function is an object of a class and hence, needs closure.
It means that high-order functions require memory allocations every time they are called. Hence, every call of a high-order function leads to a runtime overhead.
Kotlin provides inline
functions to overcome this issue. This article introduces the concept of Kotlin inline
functions and when we can use them for better memory efficiency.
Kotlin inline
Functions
Kotlin inline
functions are declared using the keyword inline
. We need to write the keyword right before declaring the function.
Using Kotlin inline
functions enhances the memory efficiency of high-order functions. When we use inline
functions, they tell the compiler to copy the function code to the call site, preventing memory allocation.
Here’s a basic example of the Kotlin inline
function.
fun main(args: Array<String>) {
exampleFunc({ println("This function will call our inline function")})
}
inline fun exampleFunc(Func: () -> Unit ) {
Func()
print("This is an inline function call and doesn't require memory allocation")
}
Output:
When Not to Use inline
Functions
While inline
functions offer an excellent way to make higher functions more efficient, there are some declarations and scenarios that do not support their use, including:
- Local class declaration
- Inner nested class declaration
- Function expressions
- Local function declarations
- Optional parameters’ default value
Best Scenarios to Use inline
Functions
The inline
functions are not useful in every situation. Here are the best scenarios to use Kotlin inline
functions:
- While accessing higher-order functions
- To enhance memory allocation efficiency
- When passing a functional type parameter
- To get better control flow
- When a function accepts another function as a parameter
- When a function accepts lambda as a parameter
Use Kotlin inline
Functions With Reified Type Parameters
We can use Kotlin inline
functions to access and retrieve the data type of the parameter passed during the call. We can do that using the reified
modifier.
fun main(args: Array<String>) {
exampleFunction<String>()
}
inline fun <reified S> exampleFunction() {
print(S::class)
}
Output:
Use noinline
in Kotlin inline
Functions
When we declare a function inline
, all its parameters become inline
. However, if we want only some parameters to be inline
, we can use the noinline
modifier.
All the expressions in front of which we use the noinline
modifier will be non-inlined.
Consider this example to understand the use of noinline
in Kotlin inline
functions.
fun main(args: Array<String>){
println("Start")
inlineFuncExample({ println("This is the first expression/parameter")
return }, // This will not throw an error as it is an inline expression
{ println("This is the first expression/parameter")
return } ) // This will throw an error as it is not an inline expression
println("End")
}
inline fun inlineFuncExample( para1: () -> Unit, noinline para2: () -> Unit ) {
para1()
para2()
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn