jQuery Sortable Table
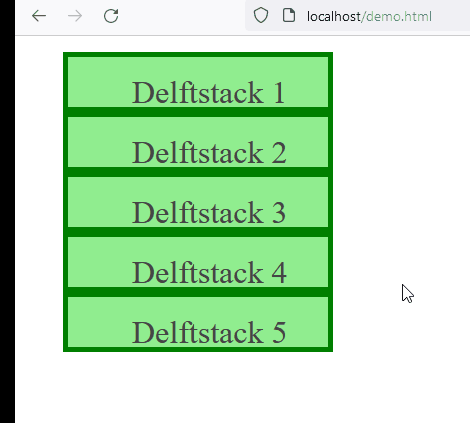
This tutorial demonstrates how to create a sortable table in jQuery.
Create a Sortable Table in jQuery
The jQuery UI
package has the method sortable()
, which implements the sort by dragging functionality for DOM objects. The syntax for this method is below.
$(selector).sortable();
The sortable
method can be implemented with lists, tables, etc. Let’s try a simple example with the default functionality of the sortable()
method.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Sortable</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<style>
#DemoList {
list-style-type: none;
width: 20%;
}
#DemoList li {
border: 5px solid green;
background-color : lightgreen;
height: 18px;
padding: 0.5em;
padding-left: 2em;
font-size: 2em;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.2/jquery-ui.js"></script>
<script>
$( function() {
$( "#DemoList" ).sortable();
});
</script>
</head>
<body>
<ul id="DemoList">
<li class="ui-state-default">Delftstack 1</li>
<li class="ui-state-default">Delftstack 2</li>
<li class="ui-state-default">Delftstack 3</li>
<li class="ui-state-default">Delftstack 4</li>
<li class="ui-state-default">Delftstack 5</li>
</ul>
</body>
</html>
The code above implements the default sortable
function for a list. See the output:
Now let’s try to create a sortable table using the jQuery UI method sortable()
method. To create a sortable table, we can also customize the sortable()
method using the options, methods, and event provided on jQuery UI documentation; let’s try an example.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Sortable Table</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<style type="text/css">
table th, table td
{
width: 150px;
padding: 10px;
border: 3px solid #ccc;
}
.Selected_Row
{
background-color: lightgreen;
color: darkgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.2/jquery-ui.js"></script>
<script type="text/javascript">
$(function () {
$("#DemoTable").sortable({
items: 'tr:not(tr:first-child)',
cursor: 'move',
axis: 'y',
dropOnEmpty: true,
scroll: true,
tolerance: "pointer",
start: function (e, ui) {
ui.item.addClass("Selected_Row");
},
stop: function (e, ui) {
ui.item.removeClass("Selected_Row");
$(this).find("tr").each(function (index) {
if (index > 0) {
$(this).find("td").eq(3).html(index);
}
});
}
});
});
</script>
</head>
<body>
<table id="DemoTable">
<tr>
<th>ID </th>
<th>First Name</th>
<th>Last Name</th>
<th>Preference</th>
</tr>
<tr>
<td>1</td>
<td>James</td>
<td>Smith</td>
<td>1</td>
</tr>
<tr>
<td>2</td>
<td>Robert</td>
<td>Smith</td>
<td>2</td>
</tr>
<tr>
<td>3</td>
<td>Maria</td>
<td>Rodriguez</td>
<td>3</td>
</tr>
<tr>
<td>4</td>
<td>James</td>
<td>Johnson</td>
<td>4</td>
</tr>
<tr>
<td>5</td>
<td>John</td>
<td>Trovolta</td>
<td>5</td>
</tr>
<tr>
<td>6</td>
<td>Jack</td>
<td>Danials</td>
<td>6</td>
</tr>
<tr>
<td>7</td>
<td>Dana</td>
<td>White</td>
<td>7</td>
</tr>
<tr>
<td>8</td>
<td>Josh</td>
<td>Thompson</td>
<td>8</td>
</tr>
<tr>
<td>9</td>
<td>Connor</td>
<td>Rollins</td>
<td>9</td>
</tr>
</table>
</body>
</html>
The code above customize the sortable()
method and uses the cursor
, axis
, dropOnEmpty
, scroll
, tolerance
options and start
, and stop
methods. The code creates a sortable table with draggable functionality.
See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook