How to Select by Name in jQuery
- Understanding jQuery Selectors
- Selecting Elements by Name
- Retrieving Values from Selected Elements
- Manipulating Selected Elements
- Adding Event Listeners to Selected Elements
- Conclusion
- FAQ
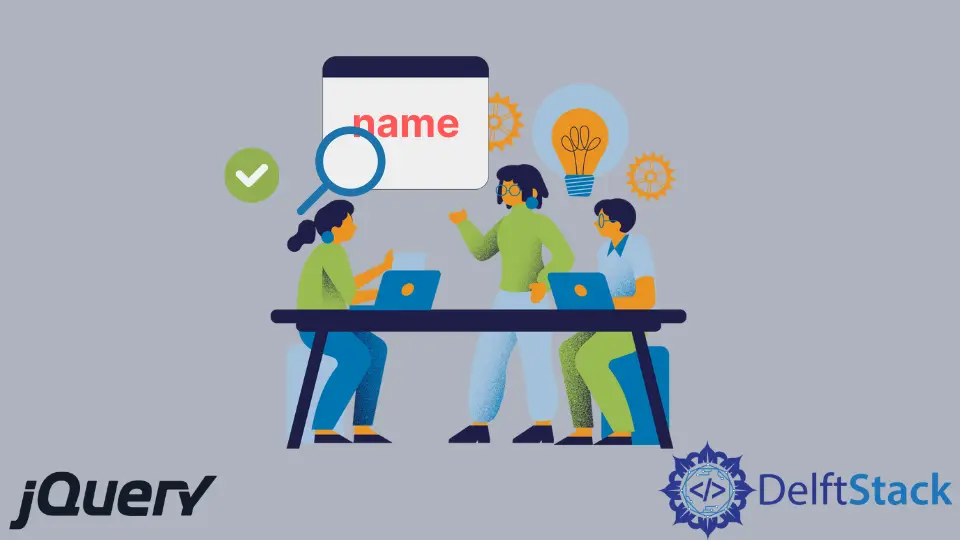
In today’s post, we’ll learn about selecting elements using name attributes in jQuery. If you’re a web developer or just getting started with jQuery, understanding how to select elements by their name can significantly streamline your coding process. This technique is particularly useful when dealing with forms, as it allows you to easily access and manipulate input fields based on their name attributes. Whether you want to retrieve values, change styles, or manage events, selecting by name can enhance your workflow. Let’s dive into the different methods to achieve this and see how you can effectively implement them in your projects.
Understanding jQuery Selectors
Before we delve into the specifics of selecting elements by name, it’s essential to grasp the concept of jQuery selectors. jQuery provides a powerful way to select HTML elements using various attributes, including class, ID, and name. The name attribute is especially useful for form elements like input fields, checkboxes, and radio buttons. By selecting elements based on their name, you can easily manipulate multiple elements at once, making your code cleaner and more efficient.
Selecting Elements by Name
To select elements by their name attribute in jQuery, you can use the following syntax:
$("[name='elementName']")
This selector allows you to target any HTML element with the specified name. For example, if you have an input field named “username,” you can select it like this:
let username = $("[name='username']");
Output:
Selected element: <input type="text" name="username">
This code snippet retrieves the input element with the name “username” and stores it in the variable username
. You can then use this variable to manipulate the element as needed, whether it’s changing its value, adding event listeners, or modifying its CSS properties.
Selecting by name is particularly beneficial when working with forms that have multiple fields sharing the same name. For instance, if you have multiple checkboxes with the same name, you can easily select all of them and perform actions like toggling their checked state or retrieving their values collectively.
Retrieving Values from Selected Elements
Once you’ve selected elements by their name, the next logical step is to retrieve their values. This is especially useful in forms where you want to gather user input. Here’s how you can do it:
let values = $("[name='options']:checked").map(function() {
return this.value;
}).get();
Output:
Selected values: ["option1", "option2"]
In this example, we are selecting all checked checkboxes with the name “options.” The map
function iterates over each checked checkbox, returning its value. Finally, the get
method converts the jQuery object into a standard JavaScript array. This approach allows you to gather all selected values efficiently.
Using this method, you can easily handle user inputs in forms, ensuring that you capture exactly what the user has selected. This is particularly useful in scenarios where users can select multiple options, such as in surveys or preference settings.
Manipulating Selected Elements
In addition to retrieving values, you can also manipulate the selected elements in various ways. For instance, you might want to change the style of all elements with a certain name or reset their values. Here’s an example of how to change the background color of all input fields named “colorChoice”:
$("[name='colorChoice']").css("background-color", "yellow");
Output:
All colorChoice inputs have a yellow background.
This simple line of code changes the background color of all input fields with the name “colorChoice” to yellow. This can be particularly useful for highlighting fields that require user attention or indicating selections in a user-friendly way.
Moreover, you can reset the values of all input fields by using:
$("[name='resetField']").val('');
Output:
All resetField inputs are now empty.
This command sets the value of each input field with the name “resetField” to an empty string, effectively clearing them. Manipulating selected elements in this way can enhance user experience and streamline interactions within your web applications.
Adding Event Listeners to Selected Elements
Another powerful feature of selecting elements by name in jQuery is the ability to add event listeners. This allows you to respond to user actions, such as clicks or changes, in real-time. Here’s how you can add a click event listener to all buttons with the name “submit”:
$("[name='submit']").on("click", function() {
alert("Form submitted!");
});
Output:
Alert: Form submitted!
In this example, when a button with the name “submit” is clicked, an alert pops up, confirming that the form has been submitted. This method can be expanded to include more complex interactions, such as form validation or AJAX submissions.
Adding event listeners to multiple elements at once not only saves time but also keeps your code organized and efficient. You can easily manage user interactions across various components of your application, leading to a more dynamic and responsive user experience.
Conclusion
Selecting elements by name in jQuery is a straightforward yet powerful technique that can greatly enhance your web development process. By mastering this method, you can efficiently manipulate forms, retrieve user inputs, and add interactivity to your web applications. Whether you’re changing styles, gathering values, or responding to events, understanding how to select by name is an essential skill for any developer. As you continue to explore jQuery, keep these techniques in mind to create more engaging and user-friendly experiences.
FAQ
-
What is the purpose of selecting elements by name in jQuery?
Selecting elements by name allows you to easily manipulate multiple HTML elements, especially in forms. -
Can I select multiple elements with the same name using jQuery?
Yes, jQuery allows you to select all elements with the same name attribute, making it convenient to manage groups of inputs. -
How do I retrieve values from selected elements by name?
You can use themap
function in jQuery to extract values from selected elements. -
Is it possible to change the styles of elements selected by name?
Absolutely! You can use jQuery’scss
method to change styles of selected elements. -
How can I add event listeners to elements selected by name?
You can use theon
method to attach event listeners to elements selected by their name attribute.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn