The resize() Method in jQuery
-
Understanding the
resize()
Method -
Using
resize()
for Responsive Design - Performance Considerations with resize()
- Advanced Usage of resize()
- Conclusion
- FAQ
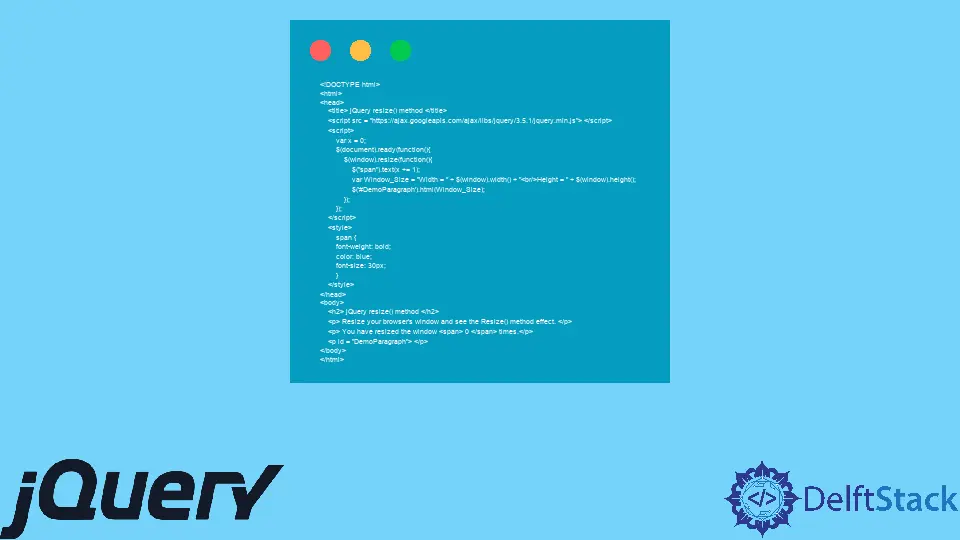
In the world of web development, jQuery has made it easier for developers to manipulate HTML elements and handle events seamlessly. One of the lesser-known yet powerful methods in jQuery is the resize()
method. This method is particularly useful for responding to changes in the size of the browser window or any other elements on the page.
In this tutorial, we will explore how to effectively use the resize()
method in jQuery, providing you with practical examples and detailed explanations. Whether you are looking to create responsive designs or enhance user experience, understanding the resize()
method can significantly elevate your web development skills.
Understanding the resize()
Method
The resize()
method in jQuery is designed to execute a function whenever the window is resized. This can be particularly useful for creating responsive layouts or dynamically adjusting elements based on the viewport size. The method can be attached to the window object or any specific element that you want to monitor for size changes.
Here’s a simple example of how to use the resize()
method in jQuery:
$(window).resize(function() {
console.log("Window resized!");
});
Output:
Window resized!
In this example, every time the window is resized, a message will be logged to the console. This basic usage can be expanded to include more complex functionalities, such as adjusting the layout or hiding/showing elements based on the new size.
Using resize()
for Responsive Design
Responsive design is a crucial aspect of modern web development. The resize()
method can be a key player in achieving a fluid and adaptable layout. By listening for resize events, you can adjust CSS properties, reposition elements, or even load different content based on the viewport size.
Here’s how you can use the resize()
method to change the background color of an element based on the window size:
$(window).resize(function() {
if ($(window).width() < 600) {
$('#myElement').css('background-color', 'blue');
} else {
$('#myElement').css('background-color', 'green');
}
});
In this example, the background color of the element with the ID myElement
changes to blue when the window width is less than 600 pixels. If the width is greater than or equal to 600 pixels, the background color changes to green. This kind of dynamic styling enhances the user experience by making the interface more intuitive and visually appealing.
Performance Considerations with resize()
While the resize()
method is powerful, it can also lead to performance issues if not used wisely. The resize event can fire multiple times during a single resize action, which may cause performance degradation, especially if the function being executed is resource-intensive. To mitigate this, you can use throttling or debouncing techniques.
Here’s an example of how to implement a debounce function with the resize()
method:
let timeout;
$(window).resize(function() {
clearTimeout(timeout);
timeout = setTimeout(function() {
console.log("Resized with debounce!");
}, 200);
});
Output:
Resized with debounce!
In this code snippet, we clear the previous timeout whenever the resize event fires. The actual function to be executed is only called after the user has stopped resizing for 200 milliseconds. This significantly reduces the number of times the function is executed, improving performance and responsiveness.
Advanced Usage of resize()
The resize()
method can also be combined with other jQuery features to create more advanced functionalities. For instance, you can use it alongside animations or to trigger AJAX calls based on the window size.
Here’s an example where we load different content based on the window size:
$(window).resize(function() {
if ($(window).width() < 600) {
$('#content').load('small-screen.html');
} else {
$('#content').load('large-screen.html');
}
});
In this example, the content of the #content
element is dynamically loaded from different HTML files depending on the window width. This allows you to serve optimized content for different devices, enhancing user experience and performance.
Conclusion
The resize()
method in jQuery is a powerful tool for creating responsive web applications. By understanding its functionality and best practices, you can significantly improve the user experience on your website. Whether you are adjusting styles, loading content, or optimizing performance, the resize()
method can help you achieve a more dynamic and engaging interface. As you continue to explore jQuery, remember that mastering such methods will elevate your web development skills and make your projects stand out.
FAQ
-
What is the purpose of the
resize()
method in jQuery?
Theresize()
method is used to execute a function whenever the window is resized, allowing developers to create responsive designs. -
How can I improve performance when using the
resize()
method?
You can improve performance by implementing throttling or debouncing techniques to limit how often the resize event handler is executed. -
Can the
resize()
method be used on elements other than the window?
Yes, theresize()
method can be used on any DOM element to monitor its size changes. -
Is the
resize()
method compatible with all browsers?
Theresize()
method is widely supported across modern browsers, but it’s always good to test for compatibility. -
How can I trigger a function after a resize event?
You can attach a function to the resize event using jQuery’s .resize() method to trigger any desired action when the event occurs.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook