jQuery remove() Method
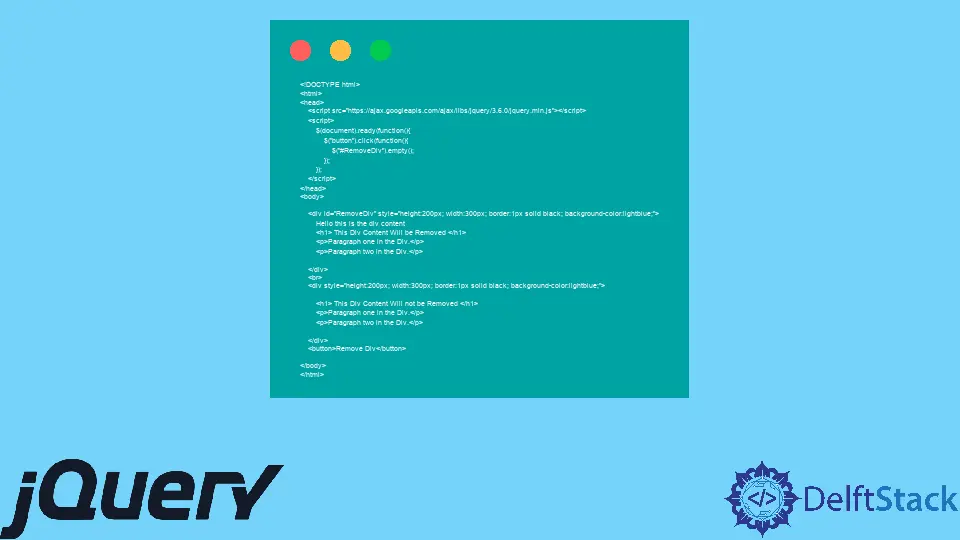
The remove()
method removes elements from DOM in jQuery. Similarly, the empty()
method removes only the selected element’s child elements in the DOM.
This tutorial demonstrates how to use the remove
and empty
methods in jQuery.
jQuery remove()
Method
The remove()
method can remove selected elements from the DOM. The method will remove the selected elements and everything inside the element.
The syntax for this method is below.
$(selector).remove(selector)
Where selector
is an optional parameter that specifies whether to remove one or more elements, if there is more than one element to remove, we can separate them by a comma. Let’s try an example for the remove()
method.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#RemoveDiv").remove();
});
});
</script>
</head>
<body>
<div id="RemoveDiv" style="height:200px; width:300px; border:1px solid black; background-color:lightblue;">
<h1> This Div Will be Removed </h1>
<p>Paragraph one in the Div.</p>
<p>Paragraph two in the Div.</p>
</div>
<br>
<div style="height:200px; width:300px; border:1px solid black; background-color:lightblue;">
<h1> This Div Will not be Removed </h1>
<p>Paragraph one in the Div.</p>
<p>Paragraph two in the Div.</p>
</div>
<button>Remove Div</button>
</body>
</html>
The code above will remove the selected div
and its child elements. See output:
As we can see, the remove()
method deletes all the elements inside the selected elements. Another method, empty()
, removes only the child elements.
jQuery empty()
Method
The empty()
method can remove all the child elements from the selected element. It also removes the content inside the child elements.
The syntax for empty()
method is below.
$('selector').empty();
Where selector
can be the id, class, or the element name, let’s try an example for the empty
method.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#RemoveDiv").empty();
});
});
</script>
</head>
<body>
<div id="RemoveDiv" style="height:200px; width:300px; border:1px solid black; background-color:lightblue;">
Hello this is the div content
<h1> This Div Content Will be Removed </h1>
<p>Paragraph one in the Div.</p>
<p>Paragraph two in the Div.</p>
</div>
<br>
<div style="height:200px; width:300px; border:1px solid black; background-color:lightblue;">
<h1> This Div Content Will not be Removed </h1>
<p>Paragraph one in the Div.</p>
<p>Paragraph two in the Div.</p>
</div>
<button>Remove Div</button>
</body>
</html>
The code above will remove only the child content of the div
, not the div
itself. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook