jQuery Local Storage
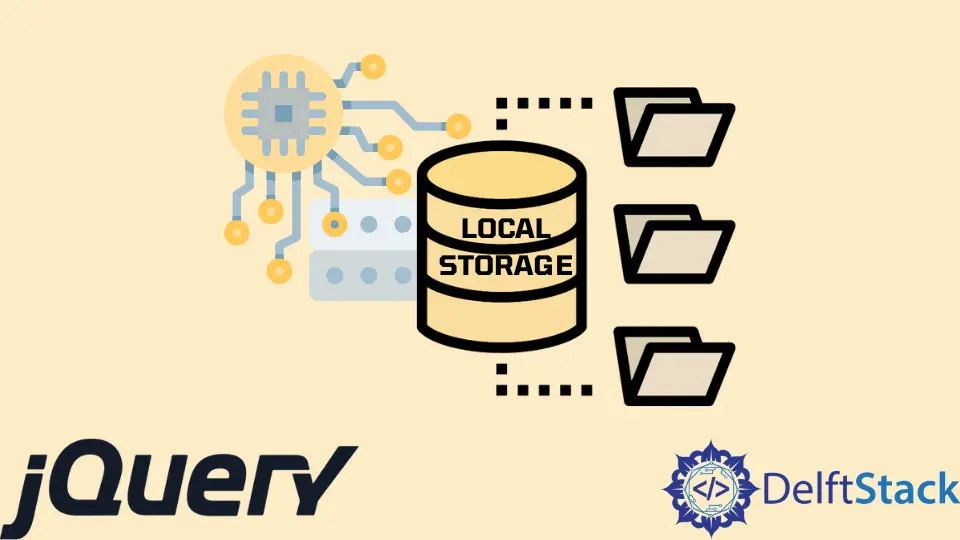
jQuery does not provide any built-in functionality to work with the local storage, but we can use the JavaScript local storage methods with jQuery objects.
This tutorial demonstrates how to use local storage with jQuery.
jQuery Local Storage
The setItem()
and getItem()
methods from JavaScript are used to store and get the data from local storage; we can use these methods to store jQuery objects in local storage and get the objects from local storage. The syntax for these methods using jQuery will be:
var html = $('element')[0].outerHTML;
localStorage.setItem('DemoContent', html);
localStorage.getItem('htmltest')
Where var html
converts the jQuery object to a JavaScript object, stores it to the localStorage
and finally gets it from the localStorage
, the DemoContent
is the key, and the html
variable is the value for the setItem
method.
Let’s try an example to set and get a text from a jQuery to the localStorage
. See example:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Local Storage</title>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Click Here</button>
<script type="text/javascript">
$(document).ready(function () {
$("#DemoButton1").click(function () {
var TextContent = $('#DemoPara1').text();
localStorage.setItem('textcontent', TextContent);
alert(localStorage.getItem('textcontent'));
});
});
</script>
</body>
</html>
The code above will set the paragraph’s content to the local storage and get it in the alert box. See output:
Now let’s try to set and get the whole HTML object to the localStorage
using jQuery. See example:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Local Storage</title>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Click Here</button>
<script type="text/javascript">
$(document).ready(function () {
$("#DemoButton1").click(function () {
var HTMLContent = $('#DemoPara1')[0].outerHTML;
localStorage.setItem('htmlcontent', HTMLContent);
alert(localStorage.getItem('htmlcontent'));
});
});
</script>
</body>
</html>
The code above will convert the jQuery object to JavaScript object using the $('#DemoPara1')[0].outerHTML;
method and store it to the local storage, finally get the object in the alert box. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook