How to Check if the Input Field Is Empty Using jQuery
-
Use the
val()
Method to Check if the Given Input Field Is Empty Using jQuery -
Use the
val()
Method With the Length Property to Check if the Given Input Field Is Empty Using jQuery
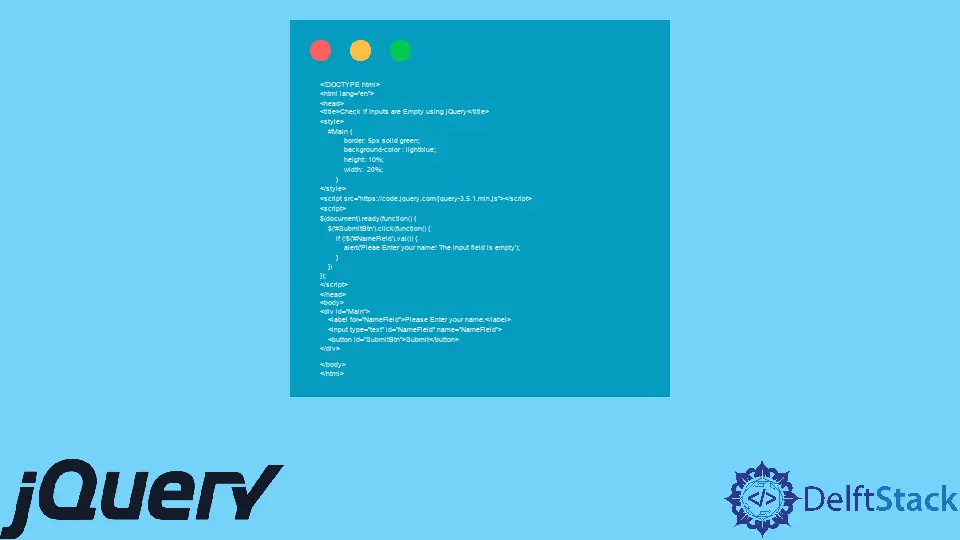
This tutorial demonstrates how to check if an input is empty in jQuery.
Use the val()
Method to Check if the Given Input Field Is Empty Using jQuery
jQuery doesn’t provide a built-in method to check if an input field is empty, but we can use the val()
method to check if the input is empty.
The val()
method can be used in two ways in jQuery. First, it can get the current value of an element, and other, it can set the current element’s value.
To check if the input is empty or not, we get its value, and if it doesn’t get a value, it means the input is empty.
We use the get functionality of the val()
method, where we get the value and check if the empty is empty or not. This can be done in two ways:
if (!$('#NameField').val())
- Put the value of input directly into theif
condition. If it is false, that means the input is empty.if ($('#NameField').val().length === 0)
- Check for the length of the input value, if it is zero that means the input is empty.
Let’s try an example to check if an input field is empty using the val()
method in the if
condition:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Check If Inputs are Empty using jQuery</title>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function() {
$('#SubmitBtn').click(function() {
if (!$('#NameField').val()) {
alert('Pleae Enter your name! The input field is empty');
}
})
});
</script>
</head>
<body>
<div id="Main">
<label for="NameField">Please Enter your name:</label>
<input type="text" id="NameField" name="NameField">
<button id="SubmitBtn">Submit</button>
</div>
</body>
</html>
The code above will display an alert if the submit button is pressed when the input field Name
is empty. See the output:
Use the val()
Method With the Length Property to Check if the Given Input Field Is Empty Using jQuery
Similarly, we can use the val()
method with length property to check if the given input field is empty or not. If the length is equal to zero, the field is empty.
Let’s try an example:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Check If Input is Empty using jQuery</title>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function() {
$('#SubmitBtn').click(function() {
if ($('#NameField').val().length === 0) {
alert('Pleae Enter your name! The input field is empty');
}
})
});
</script>
</head>
<body>
<div id="Main">
<label for="NameField">Please Enter your name:</label>
<input type="text" id="NameField" name="NameField">
<button id="SubmitBtn">Submit</button>
</div>
</body>
</html>
The code above uses the length property to check if the given field is empty or not, where we get the input value by the val()
method and then check its length. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook