jQuery를 사용하여 입력 필드가 비어 있는지 확인
-
val()
메서드를 사용하여 jQuery를 사용하여 주어진 입력 필드가 비어 있는지 확인 -
jQuery를 사용하여 지정된 입력 필드가 비어 있는지 확인하려면 Length 속성과 함께
val()
메서드를 사용하십시오.
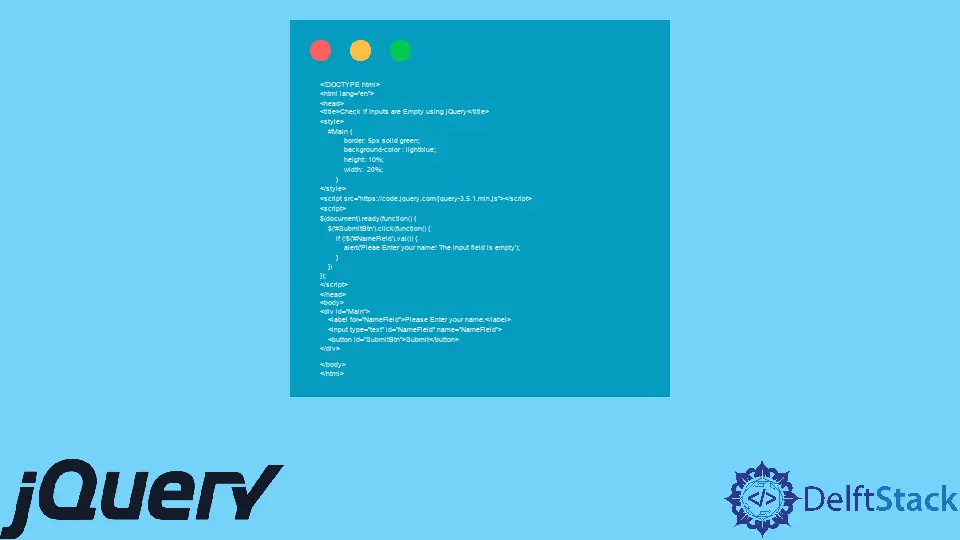
이 튜토리얼은 jQuery에서 입력이 비어 있는지 확인하는 방법을 보여줍니다.
val()
메서드를 사용하여 jQuery를 사용하여 주어진 입력 필드가 비어 있는지 확인
jQuery는 입력 필드가 비어 있는지 확인하는 내장 메서드를 제공하지 않지만 val()
메서드를 사용하여 입력이 비어 있는지 확인할 수 있습니다.
val()
메서드는 jQuery에서 두 가지 방법으로 사용할 수 있습니다. 첫째, 요소의 현재 값을 가져올 수 있고, 다른 하나는 현재 요소의 값을 설정할 수 있습니다.
입력이 비어 있는지 확인하기 위해 값을 가져오고 값을 얻지 못하면 입력이 비어 있음을 의미합니다.
val()
메서드의 get 기능을 사용하여 값을 가져오고 빈 항목이 비어 있는지 확인합니다. 이는 두 가지 방법으로 수행할 수 있습니다.
if (!$('#NameField').val())
- 입력 값을if
조건에 직접 넣습니다. false이면 입력이 비어 있음을 의미합니다.if ($('#NameField').val().length === 0)
- 입력 값의 길이를 확인합니다. 0이면 입력이 비어 있음을 의미합니다.
if
조건에서 val()
메서드를 사용하여 입력 필드가 비어 있는지 확인하는 예제를 시도해 보겠습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Check If Inputs are Empty using jQuery</title>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function() {
$('#SubmitBtn').click(function() {
if (!$('#NameField').val()) {
alert('Pleae Enter your name! The input field is empty');
}
})
});
</script>
</head>
<body>
<div id="Main">
<label for="NameField">Please Enter your name:</label>
<input type="text" id="NameField" name="NameField">
<button id="SubmitBtn">Submit</button>
</div>
</body>
</html>
위의 코드는 입력 필드 이름
이 비어 있을 때 제출 버튼을 누르면 경고를 표시합니다. 출력을 참조하십시오.
jQuery를 사용하여 지정된 입력 필드가 비어 있는지 확인하려면 Length 속성과 함께 val()
메서드를 사용하십시오.
마찬가지로 주어진 입력 필드가 비어 있는지 확인하기 위해 길이 속성과 함께 val()
메서드를 사용할 수 있습니다. 길이가 0이면 필드가 비어 있습니다.
예를 들어 보겠습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Check If Input is Empty using jQuery</title>
<style>
#Main {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function() {
$('#SubmitBtn').click(function() {
if ($('#NameField').val().length === 0) {
alert('Pleae Enter your name! The input field is empty');
}
})
});
</script>
</head>
<body>
<div id="Main">
<label for="NameField">Please Enter your name:</label>
<input type="text" id="NameField" name="NameField">
<button id="SubmitBtn">Submit</button>
</div>
</body>
</html>
위의 코드는 length 속성을 사용하여 주어진 필드가 비어 있는지 여부를 확인합니다. 여기서 val()
메서드로 입력 값을 얻은 다음 길이를 확인합니다. 출력을 참조하십시오.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook