How to Set Input Value in jQuery
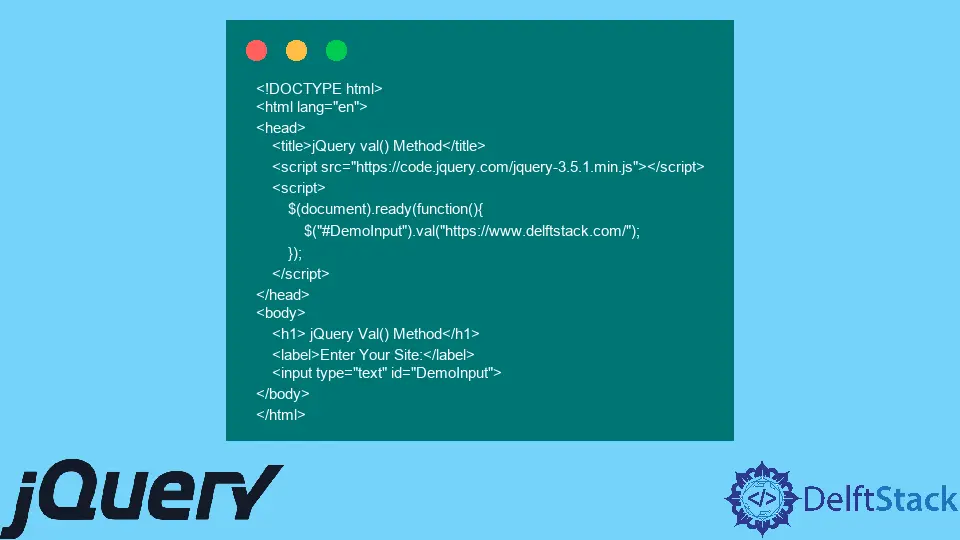
The val()
method can be used in jQuery to set the value of an input field in jQuery.
This tutorial demonstrates how to use the val()
method to set the value of an input field in jQuery.
Set Input Value in jQuery
The val()
is a built-in method in jQuery used to return or set the value of given elements. If this method returns the value, that will be the value of the first selected element.
If this method is used to set the value of an element, that value can be set for one or more elements. This method can be used in three different ways.
-
First, to return the value of the element.
Syntax:
$(selector).val()
-
To set the value of the element.
Syntax:
$(selector).val("value")
-
Set the value using a function.
Syntax:
$(selector).val(function(index, CurrentValue))
Where The selector can be the id, class, or name of the element, the
value
is the value to be used as the new value.Index
andCurrentValue
return the position of the element’s index in the set and the current value of the selected element.
Let’s have a simple example to return the value of an input field.
Example Code:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title> jQuery Val() Method</title>
<style>
p {
color: green;
margin: 10px;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<input type="text" placeholder="Type Something">
<p></p>
<script>
$( "input" ).keyup(function() {
var Input_Value = $( this ).val();
$( "p" ).text( Input_Value );
}).keyup();
</script>
</body>
</html>
The code above will return the input field’s value and write it into the given paragraph.
Output:
Now let’s try to set the value of an input field with the val()
method.
Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<title>jQuery val() Method</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("#DemoInput").val("https://www.delftstack.com/");
});
</script>
</head>
<body>
<h1> jQuery Val() Method</h1>
<label>Enter Your Site:</label>
<input type="text" id="DemoInput">
</body>
</html>
The code above will set the value of the given input to https://www.delftstack.com/
.
Output:
Let’s use the val()
method with a function.
Example Code:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery val() Method</title>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<h1> jQuery Val() Method</h1>
<p>Type anything and it will be converted into Uppercase.</p>
<p>Type and click outside.</p>
<input type="text" placeholder="type anything">
<script>
$( "input" ).on( "blur", function() {
$( this ).val(function( x, Input_Value ) {
return Input_Value.toUpperCase();
});
});
</script>
</body>
</html>
The code above will convert the input field’s value into the Uppercase.
Output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook