How to Run jQuery After Page Load
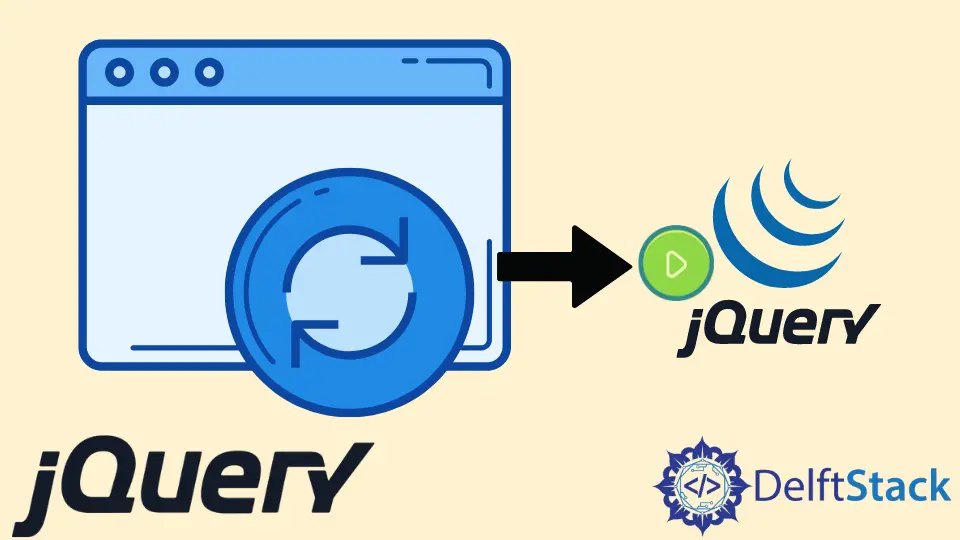
This tutorial demonstrates how to run jQuery after the page load.
Running the jQuery code after loading the page can be achieved using two methods. One is the ready()
method, and the other is the on()
method with a load event.
Use ready()
to Run jQuery After Page Load
The ready()
method in jQuery will run the jQuery code only after the fully loaded DOM. The ready()
is a built-in jQuery method that invokes the jQuery code after the DOM is fully loaded; it doesn’t wait for heavy files like images and videos to load completely.
The ready()
method is not used with the <body onload = "">
event. The syntax for this method is:
$(document).ready(function)
The ready()
method can be used only for the current document, where the function is the jQuery code you want to run after the page is loaded. Let’s try an example of this method:
<!DOCTYPE html>
<html>
<head>
<title> jQuery ready() function </title>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#h1").css({"font-size": "80px", "color": "lightblue", "background": "darkblue", "fontWeight": "bold"});
$("#h2").css({"fontSize": "50px", "fontWeight": "bold", "color": "lightblue"});
$("#para").css({"color": "blue"});
});
});
</script>
</head>
<body>
<h1 id = "h1"> Delftstack.com </h1>
<h2 id = "h2"> Example of $(document).ready() from Delftstack. </h2>
<p id = "para"> </p>
<p> This is an example for ready() method in jQuery </p>
<button> After Page Load </button>
</body>
</html>
As we can see, we use the click
event here. This click
will only work after the DOM is loaded.
See the output:
Use on()
to Run jQuery After Page Load
The on()
method can be used with the load
event to run the jQuery code after loading the page. The on()
method with load
will work when the full page is loaded, which includes heavy objects like images and videos.
The on()
method is a built-in jQuery method for different operations. The syntax for this method is:
$(selector).on(event, childSelector, data, function, map)
Where:
- The
event
is a mandatory parameter; in our case, it will beload
. - The
childSelector
is an optional parameter for specifying the child elements to which the event will be attached. - The
data
is also an optional parameter used for the data to be passed when the event is triggered. - The
function
is also an optional parameter invoked when the event is triggered. - The
map
is the event map.
Now let’s try an example with the on()
method to run the jQuery code after the page is loaded:
<!DOCTYPE html>
<html>
<head>
<title>jQuery on(load) method</title>
<script src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
</head>
<body>
<h1 id = "h1"> Delftstack.com </h1>
<h2 id = "h2"> Example of $(document).ready() from Delftstack. </h2>
<p id = "para"> </p>
<p> This is an example for ready() method in jQuery </p>
<button> After Page Load </button>
<script type="text/javascript">
$(window).on('load', DemoFunction());
function DemoFunction() {
$("#h1").css({"font-size": "80px", "color": "lightblue", "background": "darkblue", "fontWeight": "bold"});
$("#h2").css({"fontSize": "50px", "fontWeight": "bold", "color": "lightblue"});
$("#para").css({"color": "blue"});
}
</script>
</body>
</html>
The code above will make CSS changes when the page is fully loaded. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook