How to Stop setInterval Call in JavaScript
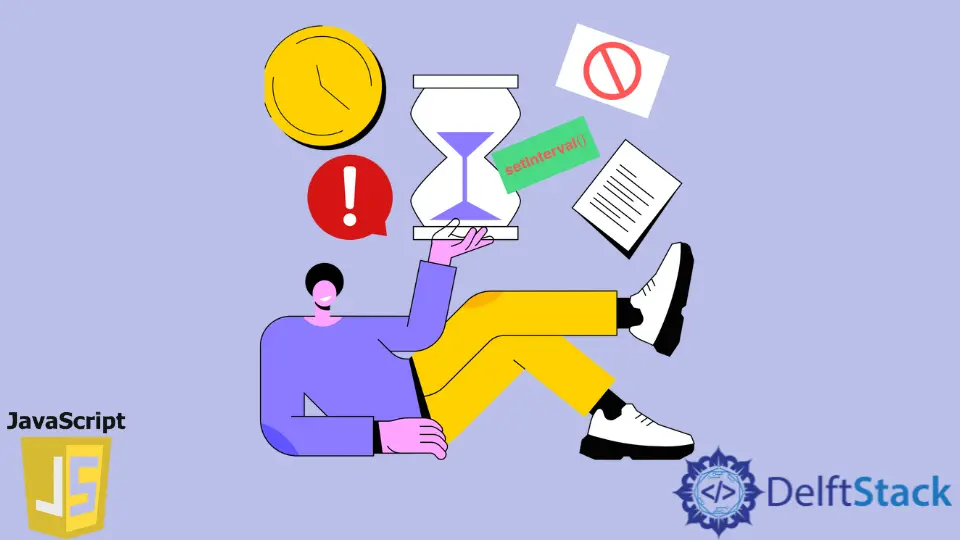
We sometimes want to execute a part of code or a function repeatedly in JavaScript. For example, if you want to show a popup to the user every few seconds, you can use the setInterval
function.
However, showing the same popup to the user again and again can be irritating and may increase your website’s bounce rate, affecting your SEO.
This condition is just one use case. However, there are many instances where you need to stop the setInterval
after some time.
This can be done with the help of clearInterval()
function.
This function takes the interval ID generated by the setInterval()
function as an input and then stops that interval function from repeating. This method is really handy and can be used in various situations.
Let’s now see how to use this function to stop the setInterval
function in JavaScript.
Stop setInterval()
Using clearInterval()
Function in JavaScript
The setInterval()
function is used to call a function or execute a piece of code multiple times after a fixed time delay. This method returns an id that can be used later to clear or stop the interval using clearInterval()
.
Syntax:
var intervalID = setInterval(func, [delay, arg1, arg2, ...]);
Below are the parameters that the setInterval()
function takes:
func
: A function you want to keep executing after some time.delay
: A delay is a time in milliseconds (this argument is optional). The function will keep on repeating after every delay.args1, ..., argN
: You can also pass various arguments to thesetInterval
function, which will then be taken as an input by the function that you have passed to thesetInterval()
. This argument is also optional.
First, let’s create a function called showText()
, which will print the word Hello
on the screen every 1 second. Our goal is to stop the interval whenever the user clicks the Stop Printing
button.
We have created a button with an onClick
attribute inside the HTML document. This attribute will execute a stopInterval()
function when a user clicks this button.
Our entire JavaScript code will go inside the index.js
file, and we will link that file to our HTML document using the script
tag as follows.
<body>
<button onclick="stopInterval()">Stop Printing</button>
<script src="index.js"></script>
</body>
We then have to create a variable intervalID
and two functions showText()
and stopInterval()
inside the JavaScript file.
Inside the showText()
function, we will first create an h1
tag and then store it inside the text
variable. Next, we will add text Hello
to the text
variable using the innerHTML
property.
Finally, we will append the text
to the body
of the HTML document.
var intervalID;
function showText() {
var text = document.createElement('h1');
text.innerHTML = 'Hello';
document.body.appendChild(text);
}
function stopInterval() {
console.log('stopping the interval...')
clearInterval(intervalID);
}
intervalID = setInterval(showText, 1000);
In the stopInterval()
function, we will first log a statement stopping the interval...
, which will tell that the stop button is pressed.
We will then use a built-in function in JavaScript called the clearInterval()
and pass the intervalID
variable that we have created.
Finally, we will call the setInterval()
function and then pass the showText
function that we have created and a time delay of 1000
milliseconds, which is 1 second.
An ID will be created whenever we call the setInterval()
function, and we will store that ID inside the intervalID
variable. This ID will come in handy when we need to stop the interval.
Here, the setInterval()
function will be called as soon as the page is loaded, and you will see the text Hello
printed on the screen. You can press the stop button whenever you want to stop the interval.
Please see the image above to see the output of the code that we have written.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn