How to Pause an Interval in JavaScript
-
Use the
setInterval()
Method to Pause an Interval in JavaScript -
Use jQuery and
setInterval()
to Pause an Interval in JavaScript
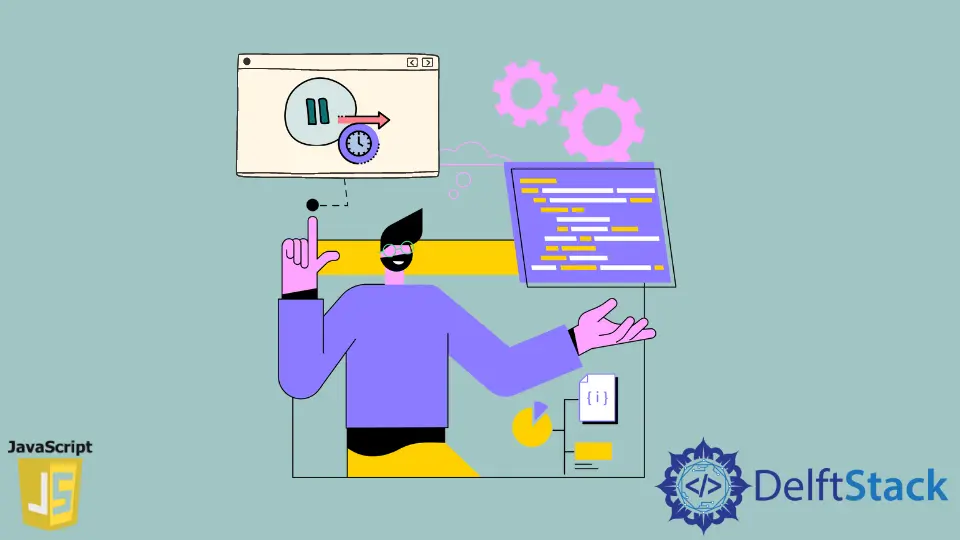
The setInterval()
method in JavaScript allows repeating a task after a certain period. There are no such parameters that will enable to pause a task amidst that time frame.
In this case, we can let the setInterval()
method have other functions as parameters. Those functions will trigger the normal flow of the timer to halt or resume.
The following sections will explain two ways of implementing this task.
Our first motive is to use the current JavaScript syntax to solve the problem. And we will also see how we can use jQuery to develop a solution.
Use the setInterval()
Method to Pause an Interval in JavaScript
Here, we will have an h1
tag that will have a predefined text in it. We will ensure it has an id as we will need to grab the DOM element to apply the setInterval()
method.
The next step is to use two button elements with individual functions to fire on click.
The JavaScript section will fetch the h1
element via the id and set a variable time
to increment every second (we will assign the millisecond
parameter to 1000).
Let’s check the code for a good view of the solution.
Code snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<h1 id="h1">Seconds: 0</h1>
<button class="play" onclick="play()">Play</button>
<button class="pause" onclick="pause()">Pause</button>
</body>
</html>
var output = document.getElementById('h1');
var isPaused = false;
var time = 0;
var t = setInterval(function() {
if (!isPaused) {
time++;
output.innerText = 'Seconds: ' + time;
}
}, 1000);
function play() {
isPaused = false;
}
function pause() {
isPaused = true;
}
Output:
As seen, the play()
and pause()
functions will set the flag variable isPaused
to the boolean values as per the condition, and thus the output.innerText()
will have a pause action in the middle of the time flow.
It is more efficient to use the JavaScript getElementById()
method over jQuery DOM element grabber. It is faster and does not require another wrapper layer.
Use jQuery and setInterval()
to Pause an Interval in JavaScript
The jQuery way of setting time intervals is also popular, and the overall drive is the same other than a slight change in syntax and agility.
Here, we will not take the onclick
attribute for the play and pause button, but the buttons will have a jQuery function in the JavaScript section to define the activity.
Look at the code lines below for a clear portrayal.
Code snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<h1>Seconds: 0</h1>
<button class="play">Play</button>
<button class="pause">Pause</button>
</body>
</html>
var output = $('h1');
var isPaused = false;
var time = 0;
var t = window.setInterval(function() {
if (!isPaused) {
time++;
output.text('Seconds: ' + time);
}
}, 1000);
$('.pause').on('click', function(e) {
e.preventDefault();
isPaused = true;
});
$('.play').on('click', function(e) {
e.preventDefault();
isPaused = false;
});
Output:
We have explicitly mentioned the window
object before the setInterval()
method. It works similarly without mentioning the window
object as well.
The overall task is based on the variable isPause
condition, and its change toggles the action of pausing the time stream.