How to Sort by Date in JavaScript
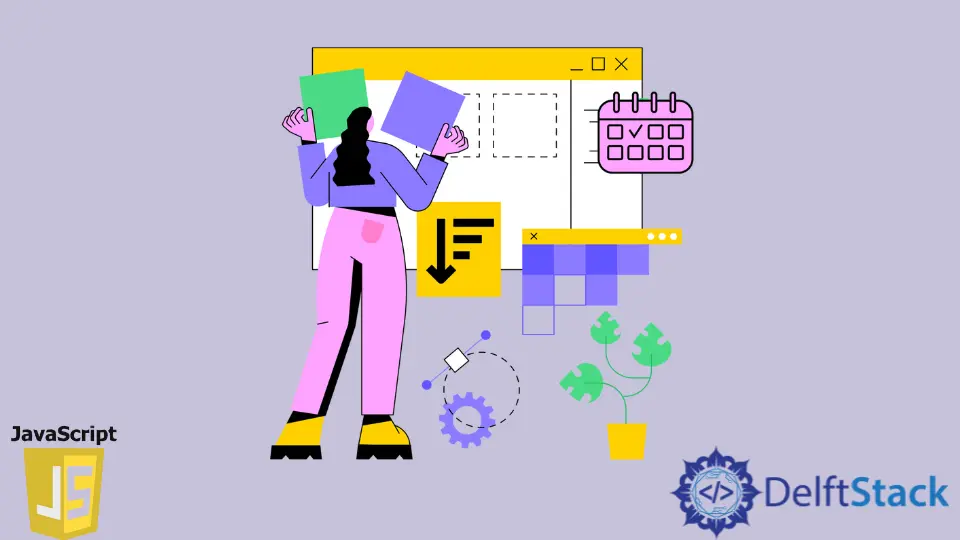
Sorting means arranging data in ascending or descending order according to a linear relationship between data items. Sorting can be done by name, number, date, and entry type.
In today’s post, we’ll learn to sort by date in ascending or descending order in JavaScript.
Sort by Date in JavaScript
There are several types of sorting algorithms in the data structure. Some of these algorithms are:
- Insertion sort
- Merge sort
- Quicksort
- Radix sort
- Heap sort
- Selection sort
- Bubble sort
JavaScript provides an in-built sort()
method that sorts the elements of an array and returns the sorted array. The default sorting order is ascending.
You can write the function if you want to sort in descending order. This method converts elements to strings and compares their UTF16 code unit values sequences.
Syntax:
sort()
sort((a, b) => {/* write logic here */})
sort(compareFunction)
sort(function compareFunction(a, b) { /* write logic here */ })
The sort()
method takes 2 arguments: a
and b
.
CompareFunction
is an optional parameter. This specifies a function that defines the sort order.
If not passed, the array elements are converted to strings and sorted based on each character’s Unicode point value. If this function is specified, by default, it takes 2 input array element, which needs to be compared against each other.
This function mutates the original array, and no copy is made.
You can learn more about the sort()
method here.
Let’s create an example comparing dates in ascending and descending order.
Example:
const datesArray1 = ['2022-03-14', '2022-04-14', '2022-01-14'];
const datesArray2 = ['2022-03-14', '2022-04-14', '2022-01-14'];
const ascDates = datesArray1.sort((a, b) => {
return new Date(a).getTime() - new Date(b).getTime();
});
const descDates = datesArray2.sort((a, b) => {
return new Date(b).getTime() - new Date(a).getTime();
});
console.log(ascDates);
console.log(descDates);
In the example, we have defined three dates. In the comparison function, we first converted the string date into a date object and then calculated the number of milliseconds since the epoch.
Try to run the above example in any browser. It will print the below result.
Output:
["2022-01-14", "2022-03-14", "2022-04-14"]
["2022-04-14", "2022-03-14", "2022-01-14"]
You may also access the complete code in this example here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn