Selection Sort in JavaScript
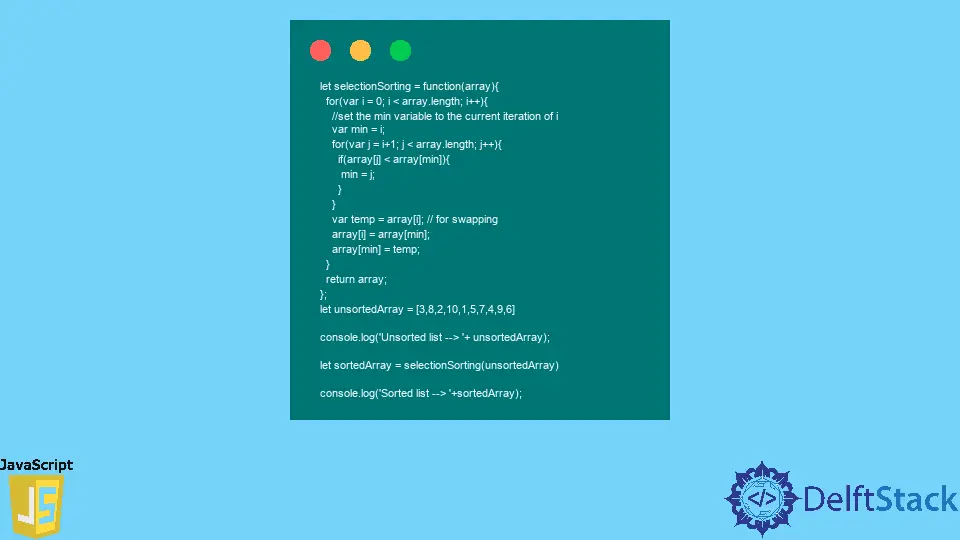
This article will teach the selection sort and how it works in JavaScript source code. We will look at an example to sort an array element using the selection sort algorithm in JavaScript.
Selection Sort in JavaScript
Selection sort is considered a simple sorting algorithm in programming languages. In this sorting algorithm, the list is divided into two parts: a sorted part on the left and an unsorted part on the right.
The sorted part will be empty at the initial stage since, at that stage, we only have the unsorted data.
In selection sort, the smallest element is selected from the list and swapped with the left-most element, and that smallest element will be the part of the sorted list. This process continues.
Time Complexity of the Selection Sort
This algorithm is not feasible for a large amount of data because of its average and worst-case complexities. The time complexity of this algorithm will be Ο(n^2)
, where n
is the number of list items.
Algorithm of the Selection Sort
The selection sort algorithm can be broken into 5 steps:
-
Set the
min
element to index 0. -
Search for the minimum element in the list.
-
Swap with value at location
min
element. -
Increment
min
element to point to the next element. -
Repeat the process until the list is sorted.
In the example below, we will use JavaScript to perform the selection sort algorithm. We will use a for
loop iteration on the list items.
let selectionSorting = function(array) {
for (var i = 0; i < array.length; i++) {
// set the min variable to the current iteration of i
var min = i;
for (var j = i + 1; j < array.length; j++) {
if (array[j] < array[min]) {
min = j;
}
}
var temp = array[i]; // for swapping
array[i] = array[min];
array[min] = temp;
}
return array;
};
let unsortedArray = [3, 8, 2, 10, 1, 5, 7, 4, 9, 6]
console.log('Unsorted list --> ' + unsortedArray);
let sortedArray = selectionSorting(unsortedArray)
console.log('Sorted list --> ' + sortedArray);
Output:
"Unsorted list --> 3,8,2,10,1,5,7,4,9,6"
"Sorted list --> 1,2,3,4,5,6,7,8,9,10"
As shown above, we declared a let
type function selectionSorting()
in which we will take an array as a parameter. Inside that function, we have used a for
loop on the passed array.
Inside the loop body, we got the first element as min
used another loop for the rest of the elements. We have checked the rest of the elements with min
using the conditional statement if()
.
If the min
element is greater than the first element of the rest of the data, we only need to update the min
variable. Then, we need to close the first loop and perform swapping, and the same process continues. When the array is sorted, the function will return the final sorted array.
We initialized the unsorted array, passed it to the selectionSort()
function as an argument, and stored the return value in the sortedArray
variable. Finally, we used the console.log()
method to display the result in logs.
Copy and save the above source code and use the JavaScript compiler to see the result.