How to Return Multiple Values in JavaScript
- Return Multiple Values From a Function With Array in JavaScript
- Return Multiple Values From a Function With Objects in JavaScript
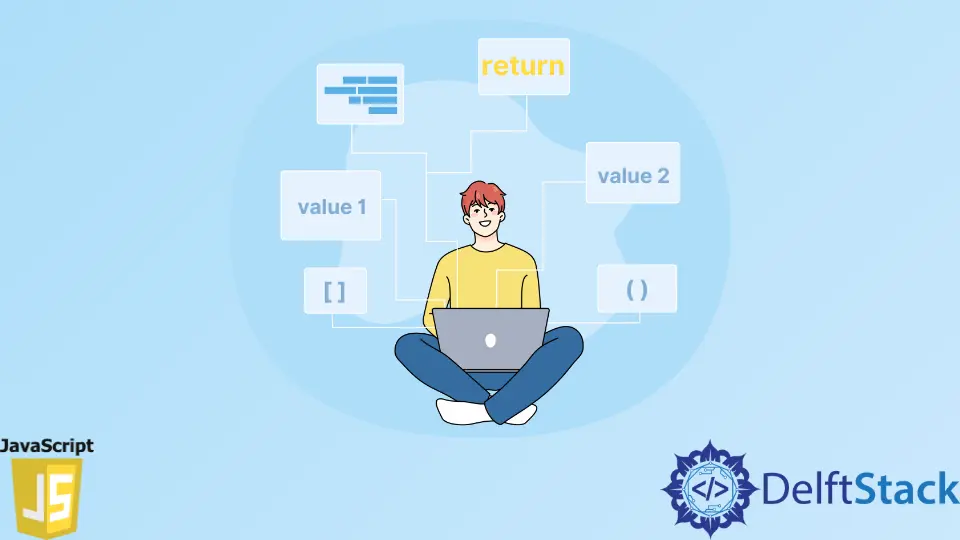
Usually, a function terminates having a return case. The return case can have a value or have nothing to pass.
In JavaScript, a function is not supported to return multiple values. So, to enable packing multiple values and passing them via a function, we take the help of arrays and objects.
Here we will demonstrate using an array to store multiple function values. And later, we will also see how you can use an object to gather the function values.
Besides, we will also figure out how to unpack the function values with a destructing assignment
and general variables declaration.
Return Multiple Values From a Function With Array in JavaScript
In this instance, the getValue
function has two variables, and we will pass them as an array to return.
The new variable feature
declaration will store the function returns in the form of the array. Like any other array traverse, we can access the values with indexes.
More simply, the feature
here is another array that grabs the sync values returned from the getValues
function in the array form.
Code Snippet:
function getValues() {
var hair = 'Short', color = 'Black';
return [hair, color];
}
var feature = getValues();
var x = feature[0];
var y = feature[1];
console.log(x + ' ' + y + ' Hair');
Output:
Use Destructing Assignment
to Unpack the Array
Generally, we can easily declare variables or objects to store the array elements. But it is tedious to define var
every time for large data.
You can also loop through an array to access values. Other than that, ES6 has a new addition to unpacking array elements like the example below.
Code Snippet:
function getValues() {
var hair = 'Short', color = 'Black';
return [hair, color];
}
var [x, y] = getValues();
console.log(x + ' ' + y + ' Hair');
Output:
Return Multiple Values From a Function With Objects in JavaScript
You can easily have a key-value
pair to structure your data with objects. For this example drive, we will again initialize the function getValues
, and this time the return case will be in an object format.
Code Snippet:
function getValues() {
var hair = 'Long', color = 'Brown';
return {hair, color};
}
var feature = getValues();
var x = feature.hair;
var y = feature.color;
console.log(x + ' ' + y + ' Hair');
Output:
The code block here has a variable feature
that works as an object. Later along with the dot(.)
method, we call the keys
to access the values.
This map
requires the key names explicitly, so the corresponding values get the floor.
Use Destructing Assignment
to Unpack Object
An object that has been passed from a function can be unpacked by declaring the ES6 convention: destructing assignment
.
Here we will explicitly declare the keys
, but the declaration will access the value pairs directly from the function. Let’s check the code block.
Code Snippet:
function getValues() {
var hair = 'Long', color = 'Brown';
return {hair, color};
}
var {hair, color} = getValues();
console.log(hair + ' ' + color + ' Hair');
Output:
As you can see, we have just passed the keys
from the function, and when are unpacking them with the key names, the output also shows the corresponding values.