How to Implement a Sleep Function in JavaScript
-
Implement a
sleep()
Function WithoutPromise
,async
andawait
in JavaScript -
Implement the
sleep()
Function WithPromise
,async
andawait
in JavaScript
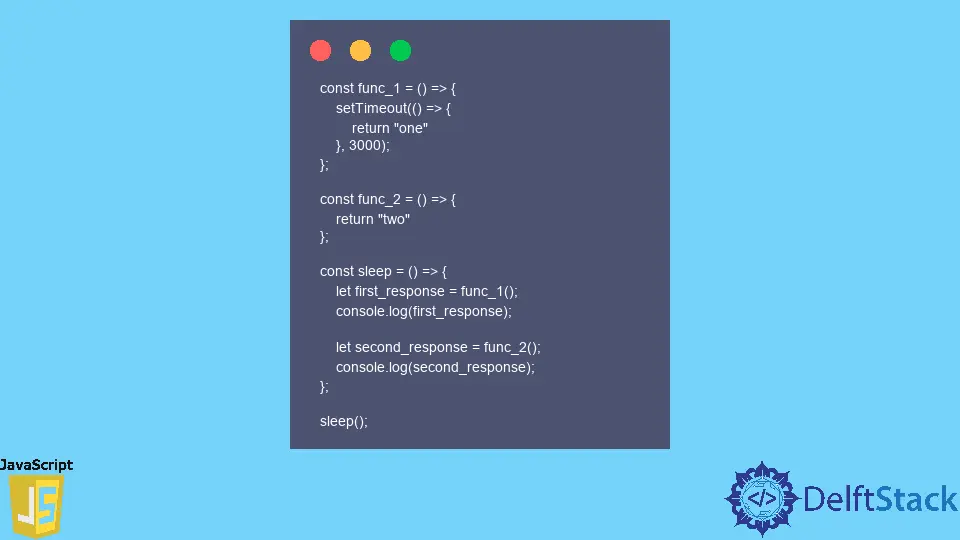
Everyone is aware that the JavaScript programming language is a single-threaded language which means it has only one call stack and one heap stack. Because of this, JavaScript code can only be executed line by line. But there are times when we want to perform some operations that take some time to execute, and during this time, keeping the user waiting for the results is not a good idea.
Let’s say we are performing an API call; then, it might take some time to get the response back from the server. Instead of waiting for the response, we can add the async
keyword before that function that is making the API call which will return a Promise
object. This will make that function asynchronous, and then with the help of await
, we will wait for some time. During that time, we will try to complete other tasks. Later, we can come back and show the API call results as soon as we get the response back from the server.
With this, we can effectively make use of the JavaScript engine. To achieve this, JavaScript provides us with a Promise
object and some keywords like async
and await
. A Promise
is an event that tells us whether a request is resolved or rejected. Whenever we request a resource, then there are two things either our request will be fulfilled or it can get rejected. Using async
, we make that function asynchronous.
Implement a sleep()
Function Without Promise
, async
and await
in JavaScript
Let’s first see what happens when we don’t use promise
, async
, and await
in our program. The below code has 3 function func_1
, func_2
and sleep()
function. The execution will start from the sleep()
function. When the execution starts, the sleep()
function will call the func_1
but notice here that inside the func_1
there is a setTimeOut()
function which has a timeout of 3000ms (3 seconds). Now the func_1
will wait for that amount of time. Till then, other lines of code will be executed.
const func_1 = () => {
setTimeout(() => {return 'one'}, 3000);
};
const func_2 = () => {
return 'two'
};
const sleep = () => {
let first_response = func_1();
console.log(first_response);
let second_response = func_2();
console.log(second_response);
};
sleep();
Output:
undefined
two
When console.log(first_response);
is executed, it will print undefined
. Since the func_1()
is currently under waiting for state and then first_response
doesn’t have any inside it. That’s the reason why it is printing undefined
. In JavaScript, there is a hoisting concept where all the variables in a program that are declared but not initialized will be assigned the value of undefined
. That’s how we get undefined
as an output.
The second function, func_2()
, is straightforward. Here it will simply return the string two
, and then it will be stored inside the variable second_response
and then it will be printed on the output window.
Implement the sleep()
Function With Promise
, async
and await
in JavaScript
Here, we will use the Promise
, async
and await
and making some minor modifications in our program. Notice how we are getting undefined
value of the func_1()
function. So, let’s fix this by making the sleep()
function asynchronous with async
and then wait or sleep for some time with the help of await
and then get the response as Promise()
object, which is returned by async
.
const func_1 = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('The request is successful.');
}, 3000);
});
};
const func_2 = () => {
return 'two'
};
const sleep = async () => {
let first_response = await func_1();
console.log(first_response);
let second_response = func_2();
console.log(second_response);
};
sleep();
Output:
The request is successful.
two
Just adding the keyword async
in front of the function doesn’t make that function asynchronous. For that, wherever you want to make an asynchronous call or wait, we have to use await
on that function, in this case before func_1()
.
The await
keyword is written before a function that allows the function to wait for some time and then execute it. The time until it will wait until it executes the function depends on the time specified inside the setTimeOut()
function.
Inside the function, func_1
, we are returning a Promise
object which takes two parameters, the first is the resolve
and the second is the reject
. If the promise
is successfully fulfilled, we can use the resolve
method; otherwise, we can use the reject
method.
If you run this program, the func_1()
will be called first, and then the program will wait for 3 seconds since we have used setTimeOut()
of 3000ms. After that, it will return the string The request is successful.
as the result of the func_1
, it will be stored in the first_response
variable. The func_2()
will be called and will directly return two
as a result, which will then be stored inside the second_response
variable. This is how we achieve sleep()
functionality in JavaScript.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn