How to Wait for 5 Seconds in JavaScript
-
Use the
setTimeout()
Function to Wait for 5 Seconds in JavaScript -
Use
promises
andasync/await
to Wait for 5 Seconds in JavaScript -
Use the
for
Loop to Implement a Synchronousdelay
Function in JavaScript - Conclusion
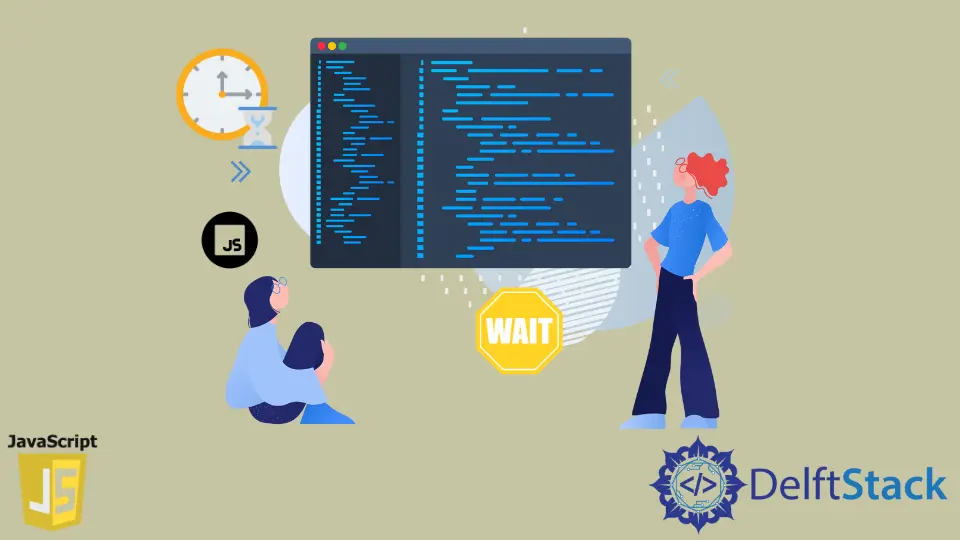
In JavaScript, there are scenarios where you might have to introduce a delay in the execution of your code, such as when you want to display a certain message for a specific duration or add a simple pause between certain actions. One common requirement is to wait for a specific period, for example, 5 seconds, before proceeding to the next steps.
This article will explore different approaches to introducing a 5-second delay in JavaScript.
Methods for implementing a 5-second delay in JavaScript include using setTimeout()
, a for
loop, async/await
, and Promises. We will cover each method, providing options based on your use case.
Additionally, we will discuss scenarios where delaying code execution is useful, such as creating smooth transitions, implementing timeouts, or introducing delays in asynchronous operations.
Use the setTimeout()
Function to Wait for 5 Seconds in JavaScript
The asynchronous setTimeout()
method can be referred to as one of the higher-order functions that can take a callback function as its argument and is capable of executing that function even after the input time gets elapsed. The time given in the parameter is in the unit of ms
.
What it means is that this global method does not block or pause the execution of other functions existing within the function stack.
The setTimeout
method mainly takes two main parameters: code
and delay
. The code
parameter is essential to specify, while the delay
parameter is optional, whose default value amounts to 0.
We can have other additional parameters passed through to the function we passed.
console.log('I am the first log');
setTimeout(function() {
console.log('I am the third log after 5 seconds');
}, 5000);
console.log('I am the second log');
Output:
Use promises
and async/await
to Wait for 5 Seconds in JavaScript
One method to implement the delay
function in the asynchronous context is to combine the async/await
concept and promises
concept. A delay
function can be created to return a new promise
inside, which we’ll call the setTimeout()
method and set it to the waiting time we desire.
After that, we can call that delay
function inside any asynchronous context as we like.
function delay(n) {
return new Promise(function(resolve) {
setTimeout(resolve, n * 1000);
});
}
async function myAsyncFunction() {
// Do what you want here
console.log('Before the delay')
await delay(5);
console.log('After the delay')
// Do what you want here too
}
myAsyncFunction();
Output:
The delay
function can also be optimized using the arrow syntax =>
in ECMAScript 6.
const delay = (n) => new Promise(r => setTimeout(r, n * 1000));
Use the for
Loop to Implement a Synchronous delay
Function in JavaScript
Suppose we don’t have any asynchronous context to use the above delay()
function or don’t want to use the setTimeout()
function. In that case, we can implement a synchronous delay
function with a normal for
loop and Date()
class to calculate time.
The implementation of this function is pretty simple. It’s all about keeping the CPU busy for the desired time. This is by calculating the elapsed time and comparing it to the waiting time so we can continue the execution after it.
function syncDelay(milliseconds) {
var start = new Date().getTime();
var end = 0;
while ((end - start) < milliseconds) {
end = new Date().getTime();
}
}
console.log('Before the delay')
syncDelay(5000);
console.log('After the delay')
Output:
Conclusion
Introducing a 5-second delay in JavaScript can be achieved using various methods, such as setTimeout()
, a for
loop, async/await
, or Promises. Whether you need to pause code execution, add delays between actions, or create smooth transitions, understanding these techniques is essential.
You can effectively introduce delays without blocking the main thread by leveraging the setTimeout()
function, using a for
loop, or utilizing async/await
with Promises. Consider the specific requirements of your project and select the appropriate method accordingly.
Remember that waiting for 5 seconds can be useful for implementing timeouts, managing asynchronous operations, or creating user-friendly interactions. By incorporating these delay techniques into your JavaScript code, you can achieve better control over the timing of your application’s execution flow.