How to Get the Timestamp in JavaScript
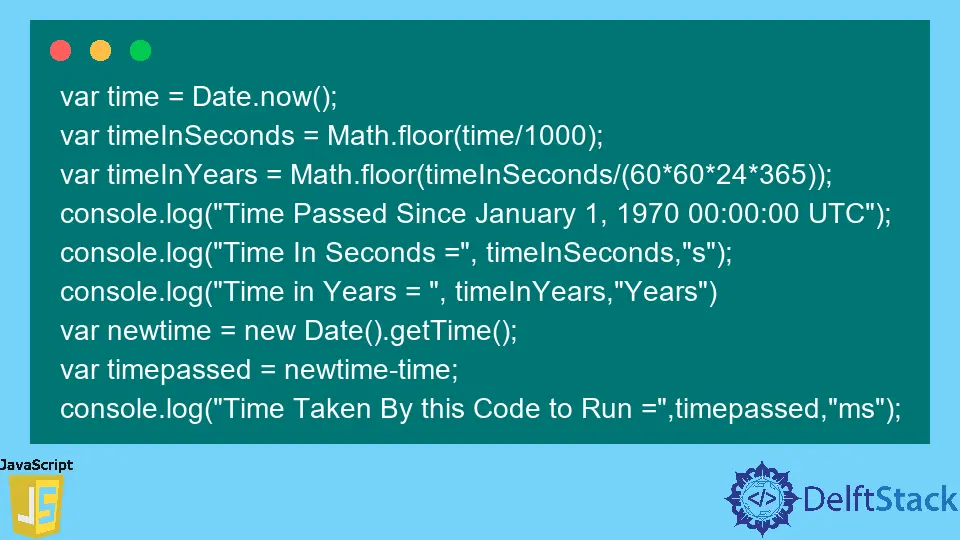
You can use the Date.now()
function in JavaScript to get the timestamp.
This tutorial demonstrates the process of using the Date.now()
function, which you can refer to as your guide.
Get the Timestamp Using the Date.now()
Function in JavaScript
We can use the Date.now()
function to get the timestamp in milliseconds in JavaScript. The Date.now()
function returns the number of milliseconds passed since 01-01-1970. For example, let’s find the number of milliseconds passed using the Date.now()
function in JavaScript. See the code below.
var t = Date.now();
console.log(t);
Output:
1622872385158
The output shows the number of milliseconds passed since January 1, 1970, 00:00:00 UTC. Let’s convert the time to seconds and years and show it on the console using the console.log()
function. See the code below.
var t = Date.now();
console.log(t);
var time = Date.now();
var timeInSeconds = Math.floor(time / 1000);
var timeInYears = Math.floor(timeInSeconds / (60 * 60 * 24 * 365));
console.log('Time Passed Since January 1, 1970 00:00:00 UTC');
console.log('Time In Seconds =', timeInSeconds, 's');
console.log('Time in Years = ', timeInYears, 'Years')
Output:
Time Passed Since January 1, 1970 00:00:00 UTC
Time In Seconds = 1622872385 s
Time in Years = 51 Years
As you can see in the output, 51 years have passed since 1970; this means we’re currently living in the year 2021. Similarly, we can also find the current month, day, and time using conversion formulas. The Date.now()
function is commonly used to find the time taken by a program or piece of code to run. You can find the time at the start and end of the code and evaluate the time difference. For example, let’s find the time taken by the code above to run. See the below code.
var time = Date.now();
var timeInSeconds = Math.floor(time / 1000);
var timeInYears = Math.floor(timeInSeconds / (60 * 60 * 24 * 365));
console.log('Time Passed Since January 1, 1970 00:00:00 UTC');
console.log('Time In Seconds =', timeInSeconds, 's');
console.log('Time in Years = ', timeInYears, 'Years')
var newtime = new Date().getTime();
var timepassed = newtime - time;
console.log('Time Taken By this Code to Run =', timepassed, 'ms');
Output:
Time Passed Since January 1, 1970 00:00:00 UTC
Time In Seconds = 1622872385 s
Time in Years = 51 Years
Time Taken By this Code to Run = 1 ms
In the output, the time taken by this code is 1 millisecond. Here, you can use the Date.now()
function to check the performance of different functions. In the program above, we use the Math.floor()
function to convert a floating-point number into an integer. You can also use the bitwise operators like the bitwise NOT ~~
to convert the floating-point number into an integer. The bitwise operators are slightly faster than the Math.floor()
function, but they may not work for long numbers.