How to Set Selected Option in JavaScript
-
Set Select Element Using
document.getElementById()
in JavaScript -
Set Select Element Using
document.querySelector()
in JavaScript
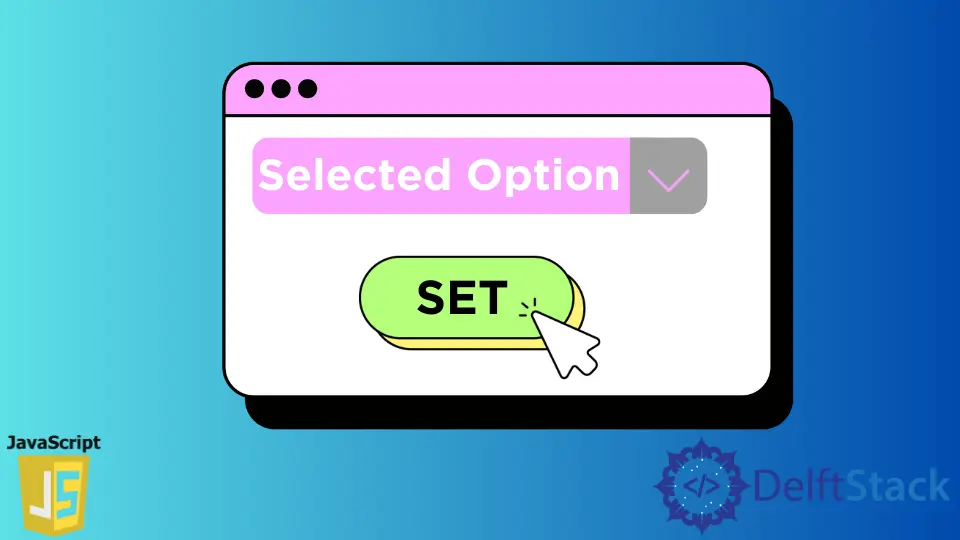
select
is an HTML input element that specifies the list of drop-down options from which the user can choose any option. In today’s post, we will learn how to set the value of this HTML element in JavaScript.
Set Select Element Using document.getElementById()
in JavaScript
This built-in document
method provided by JavaScript returns the element object whose id
matches the specified id
. The actual value is set by the line element.value = 'NEW_VALUE'
.
Syntax
document.getElementById($id);
Paramater
$id
: It is a mandatory parameter that specifies the id
of an HTML attribute that must match.
Return Value
Returns the corresponding DOM element object if the corresponding element is found; otherwise, it returns null
.
Example code:
<select id="osDemo">
<option value="Linux">Linux</option>
<option value="Windows">Windows</option>
<option value="MacOS">MacOS</option>
</select>
<button type="button" onclick="document.getElementById('osDemo').value = 'MacOS'">
Click to Update to MacOS.
</button>
Output:
Set Select Element Using document.querySelector()
in JavaScript
This is a built-in document
method provided by JavaScript and returns the element object whose selector
matches the specified selector. The only difference between querySelector()
and querySelectorAll()
is that the first one is a single matching element object, and then all objects of the matching element are returned. If an invalid selector is passed, a SyntaxError
is issued. The actual value is set by the line element.value = 'NEW_VALUE'
Syntax
document.querySelector($selector);
Paramater
$selector
: It is a mandatory parameter that specifies the matching condition of either class
or id
. This string must be a valid CSS
selection string.
Return Value
Returns the first matching element object that meets the selector condition within the DOM.
Example code:
<select id="osDemo" class="osDemoClass">
<option value="Linux">Linux</option>
<option value="Windows">Windows</option>
<option value="MacOS">MacOS</option>
</select>
<button type="button" onclick="document.querySelector('.osDemoClass').value = 'MacOS'">
Click to Update to MacOS.
</button>
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn