How to Return Values of a Function in JavaScript
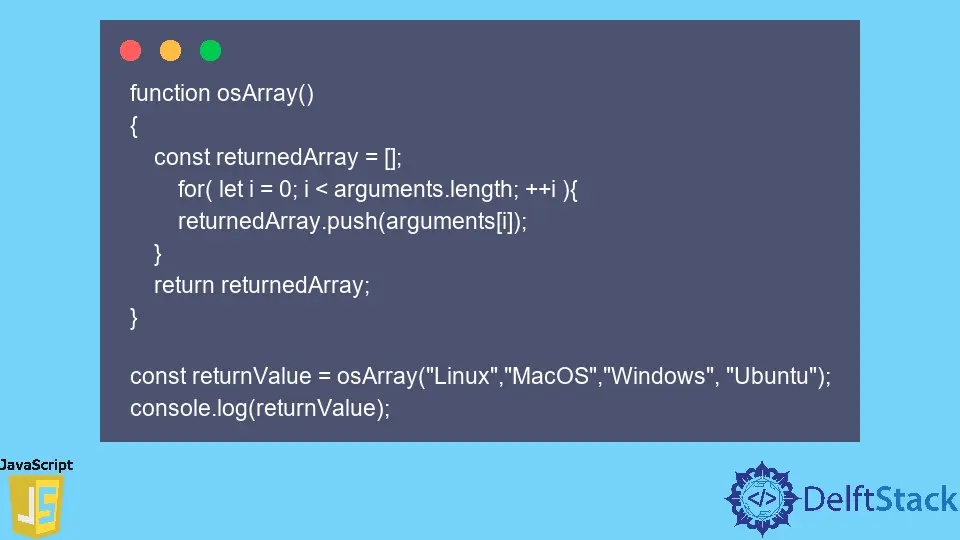
JavaScript functions are one of the essential building blocks which execute the series of steps. These functions are like procedures.
A set of instructions are provided inside the function that executes the task. The only difference is that the function needs the input parameter and must return something to the caller function. To use a function, you need to define it somewhere in the scope you want to call it.
This article is going to show how to return values from a JavaScript function.
Return Values From the JavaScript Function
A function definition consists of the function
keyword. A function definition is also called a function statement or function declaration. It is followed by:
- The name of the function. This function name should be unique for the file.
- A list of function input parameters. These parameters are enclosed in parentheses and separated by commas.
- The content of the function is enclosed in curly brackets, {…}.
Syntax:
Before ES6
function fnName(params) {
/* perform actions on params */
return value;
}
After ES6
param => expression
ES6
standard supports the arrow function, which does not require defining the function keyword before defining the function, returning the value, and enclosing the code inside curly brackets. For more information about the ES6 function, read the documentation of arrow functions method.
The function can return anything like an array, literal object, string, integer, a boolean value, or an object of a custom type you create that encapsulates the return values. You can pass anything to the function like an array, literal object, or custom object to a method to cast the values.
Functions can be declared as expressions. This function is called an anonymous function.
Anonymous functions do not have any function name. Function needs to be called to execute the steps inside the function.
For more information, read the documentation of Functions
method.
Object example:
function osObject() {
const returnedObject = {};
for (let i = 0; i < arguments.length; ++i) {
returnedObject['os' + i] = arguments[i];
}
return returnedObject;
}
const returnValue = osObject('Linux', 'MacOS', 'Windows', 'Ubuntu');
console.log(returnValue);
In the above code example, we created the function osObject
, which takes the input parameters and returns the object with the input values. The return
keyword indicates that the function is returning something to the caller.
In our example, we are returning osObject
. The above code’s output will look something like this:
Output:
{
os0: "Linux",
os1: "MacOS",
os2: "Windows",
os3: "Ubuntu"
}
Array Example:
function osArray() {
const returnedArray = [];
for (let i = 0; i < arguments.length; ++i) {
returnedArray.push(arguments[i]);
}
return returnedArray;
}
const returnValue = osArray('Linux', 'MacOS', 'Windows', 'Ubuntu');
console.log(returnValue);
In the above code example, we created the function osArray
, which takes the input parameters in arguments
and returns the Array with the input values. The return
keyword indicates that the function is returning something to the caller.
In our example, we are returning osArray
. The output of the above code’s output will look something like this:
Output:
["Linux", "MacOS", "Windows", "Ubuntu"]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn