How to Redirect Page After Delay in JavaScript
-
Use the
setTimeout()
Method to Redirect Page After Delay in JavaScript -
Use the
preventDefault()
Method to Disable Default Behaviors of HTML Elements in JavaScript
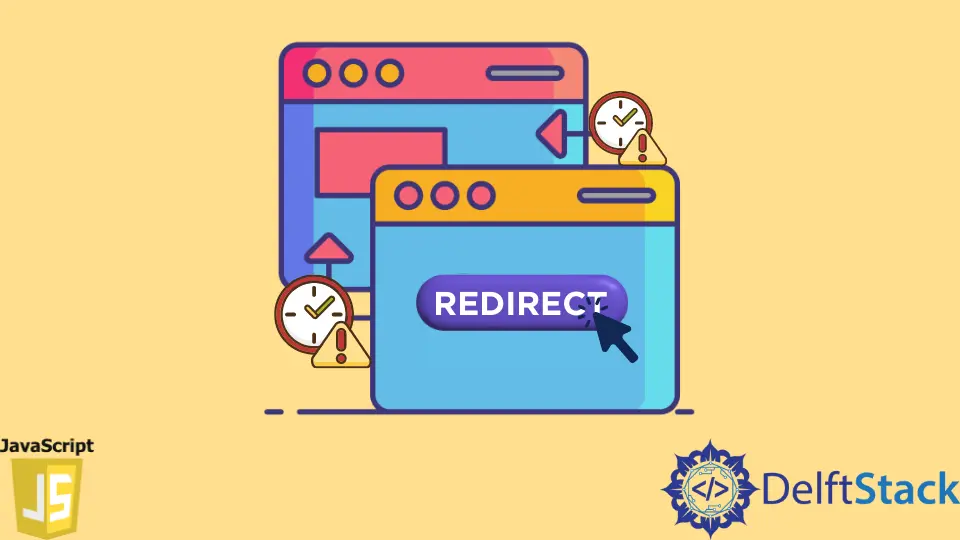
Let’s understand how to redirect to another page after a delay in JavaScript in detail.
Use the setTimeout()
Method to Redirect Page After Delay in JavaScript
You sometimes want to show something on the webpage after the user has clicked on the link, like a text message, before redirecting the user to another page. The anchor tag is not directly possible to delay the redirection for a few seconds and then go to another page.
For this, we have to use the setTimeout
method. This method adds a delay and then redirects the user to another page.
But just using the setTimeout
method will not work. You also have to prevent the default behavior of the anchor tag before using the setTimeout
method.
The setTimeout()
method in JavaScript allows you to execute a piece of code or a function after some time. The setTimeout()
function takes two parameters.
The first parameter is the callback function, and the second parameter is the time for which you want to delay the execution of the code.
To implement this, we will first create two web pages, index.html
and newpage.html
. Inside the index.html
, we have an h1
tag for heading text.
Then we will have an anchor tag a
, which has an id
of link
and href
property.
The href
of the anchor link will be #
and will not point anywhere. We will set the href
link inside the JavaScript.
The anchor tag will also have the text Redirect me
. Finally, we will link the JavaScript file using the script
tag, as shown below.
// index.html
<body>
<h1>Home Page</h1>
<a id="link" href="#">Redirect me</a>
<script src="./script.js">
</script>
</body>
Inside the newpage.html
, we will have an h1
text as shown below. We aim to redirect the user from the index.html
page to newpage.html
after clicking the anchor link.
// newpage.html
<body>
<h1>Welcome to new page...</h1>
</body>
After setting up our HTML code, it’s time to work on the JavaScript code. We will first get the anchor HTML element inside the JavaScript using its id property link
and store this inside the url
variable.
Then we will set the click listener on the url
variable using the addEventListener()
method.
Inside the click event listener, we will first print a message on the console url clicked...
. This will let us know that the user has clicked on the link.
Then we will add a setTimeout()
method.
We will execute the function after 3000 milliseconds, i.e., after 3sec. We will set the URL inside this method using the window.location.href
property.
After successfully redirecting to the new page, we will print a message on the console.
var url = document.getElementById('link');
url.addEventListener('click', () => {
console.log('url clicked...')
setTimeout(() => {
window.location.href = 'http://127.0.0.1:5500/newpage.html';
console.log('timeout executed...')
}, 3000);
});
This entire thing works on an event loop.
Any code before and after the setTimeout()
method will be executed first. After it finishes executing the code, it will then start the execution of the setTimeout
or setInterval
methods.
To understand how the above code is working internally, you must understand the event loop concept in JavaScript.
At this point, if you run the above code, it will now work. As soon as you click the URL, it will instantly redirect you to the next page, which we don’t want.
Even though we have added the setTimeout()
method, it instantly switches to that link without delay. This is because, by default, every HTML element has some default behaviors.
Its default behavior is to go to the next page after clicking anchor links.
Use the preventDefault()
Method to Disable Default Behaviors of HTML Elements in JavaScript
There are times when we have to disable these default behaviors of the HTML elements. We use a method called preventDefault()
to happen.
You must pass an event as a parameter to a function you call. In this case, we pass the event e
to the click event, and then on that event, we set the preventDefault()
method.
var url = document.getElementById('link');
url.addEventListener('click', (e) => {
e.preventDefault();
console.log('url clicked...')
setTimeout(() => {
window.location.href = 'http://127.0.0.1:5500/newpage.html';
console.log('timeout executed...')
}, 5000);
});
If you run the code and click on the link, it will take you to the next page after a time delay mentioned in the setTimeout()
method.
To open the URL in a new tab, you can use the window.open()
method instead of window.location.href()
. In the first parameter of the window.open()
, you can pass the URL, and in the second parameter, you have to specify where you want to open the link.
Since we want to open the delay inside a new tab, we pass _blank
to the second parameter.
window.open('http://127.0.0.1:5500/newpage.html', '_blank');
If you run the code, it will open the URL inside a new tab.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn