How to Parse HTML in JavaScript
- Method 1: Using the DOMParser
- Method 2: Using jQuery
- Method 3: Using Element.innerHTML
- Conclusion
- FAQ
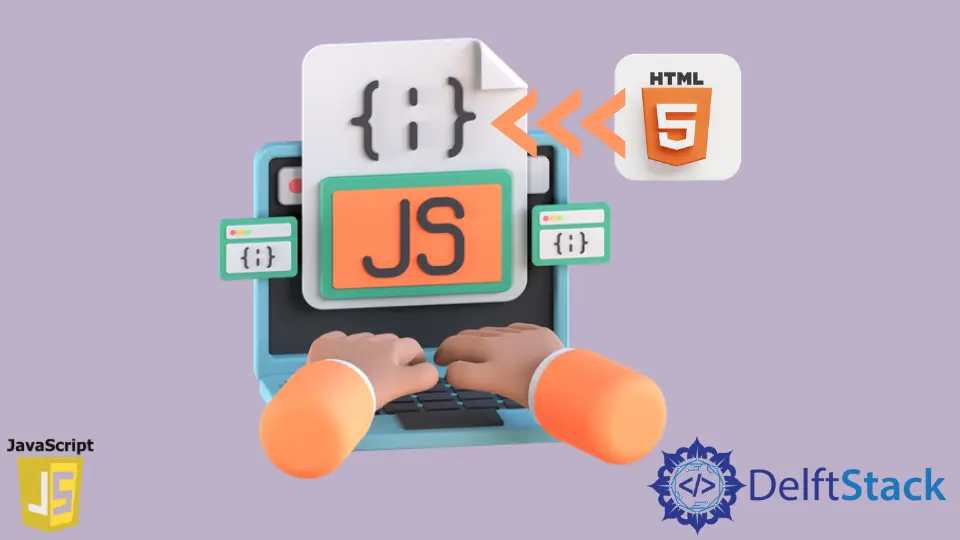
In today’s post, we’ll learn how to parse HTML in JavaScript. Whether you’re developing a dynamic web application or simply want to manipulate HTML content, understanding how to parse HTML effectively is crucial. Parsing HTML allows you to extract, modify, and utilize data from HTML documents, making it an essential skill for any web developer.
In this article, we will explore various methods to parse HTML in JavaScript, including using the browser’s built-in DOMParser, leveraging jQuery, and employing the Element.innerHTML property. By the end of this post, you’ll have a solid grasp of how to parse HTML and integrate it into your projects seamlessly.
Method 1: Using the DOMParser
The DOMParser is a built-in JavaScript object that allows you to parse XML or HTML source code from a string into a DOM Document. This method is straightforward and works in most modern browsers.
const htmlString = '<div><h1>Hello, World!</h1><p>This is a paragraph.</p></div>';
const parser = new DOMParser();
const doc = parser.parseFromString(htmlString, 'text/html');
const heading = doc.querySelector('h1').textContent;
const paragraph = doc.querySelector('p').textContent;
console.log(heading);
console.log(paragraph);
Output:
Hello, World!
This is a paragraph.
The code above starts with a simple HTML string that contains a heading and a paragraph. We then create a new instance of DOMParser and use the parseFromString
method to convert the HTML string into a document. After parsing, we can use standard DOM methods like querySelector
to extract the desired elements. This method is particularly useful when you need to parse HTML dynamically, such as when fetching data from an API.
Method 2: Using jQuery
If you are already using jQuery in your project, you can take advantage of its powerful HTML manipulation capabilities. jQuery simplifies the process of parsing HTML and provides a more concise syntax.
const htmlString = '<div><h1>Hello, jQuery!</h1><p>This is a paragraph.</p></div>';
const $parsedHTML = $(htmlString);
const heading = $parsedHTML.find('h1').text();
const paragraph = $parsedHTML.find('p').text();
console.log(heading);
console.log(paragraph);
Output:
Hello, jQuery!
This is a paragraph.
In this example, we start with the same HTML string. By wrapping the string with the jQuery $()
function, we can create a jQuery object that allows us to use jQuery methods directly. The find
method is used to locate and extract the heading and paragraph text. This method is particularly useful for developers already familiar with jQuery, as it provides a more intuitive way to manipulate HTML elements.
Method 3: Using Element.innerHTML
Another straightforward method to parse HTML in JavaScript is by using the innerHTML
property of a DOM element. This approach is efficient for small snippets of HTML and is easy to implement.
const htmlString = '<div><h1>Hello, innerHTML!</h1><p>This is a paragraph.</p></div>';
const tempDiv = document.createElement('div');
tempDiv.innerHTML = htmlString;
const heading = tempDiv.querySelector('h1').textContent;
const paragraph = tempDiv.querySelector('p').textContent;
console.log(heading);
console.log(paragraph);
Output:
Hello, innerHTML!
This is a paragraph.
In this code snippet, we create a temporary <div>
element and assign the HTML string to its innerHTML
property. This effectively parses the HTML, allowing us to use DOM methods to retrieve the heading and paragraph text. This method is quick and works well for small amounts of HTML, but be cautious when dealing with larger documents, as it might not be as efficient as other methods.
Conclusion
Parsing HTML in JavaScript is a vital skill for web developers, enabling dynamic content manipulation and data extraction. Whether you choose to use the DOMParser, jQuery, or the innerHTML property, each method has its advantages and use cases. Understanding these techniques will empower you to handle HTML more effectively in your projects. As you continue to explore JavaScript, keep these methods in your toolkit to enhance your web development skills.
FAQ
- What is the DOMParser in JavaScript?
The DOMParser is a built-in object in JavaScript that allows you to parse XML or HTML strings into a DOM Document, enabling easy manipulation of the parsed content.
-
Can I use jQuery to parse HTML?
Yes, jQuery simplifies HTML parsing and manipulation, allowing you to use its powerful methods to work with HTML strings efficiently. -
What is the advantage of using innerHTML?
Using innerHTML is a quick and straightforward way to parse small snippets of HTML. It allows you to create a temporary DOM structure without the overhead of a full parser. -
Are there any performance concerns with these methods?
While all methods are efficient for small to moderate amounts of HTML, for larger documents, using DOMParser or jQuery may provide better performance and flexibility compared to innerHTML. -
Can I parse HTML from an API response?
Yes, you can parse HTML strings received from API responses using any of the methods discussed, making it easy to work with dynamic content in your applications.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn