How to Print to Console in JavaScript
-
Print to Console With the
console.log()
Method in JavaScript -
Print to Console With the
console.warn()
Method in JavaScript -
Print to Console With the
console.error()
Method in JavaScript -
Print to Console With the
console.info()
Method in JavaScript
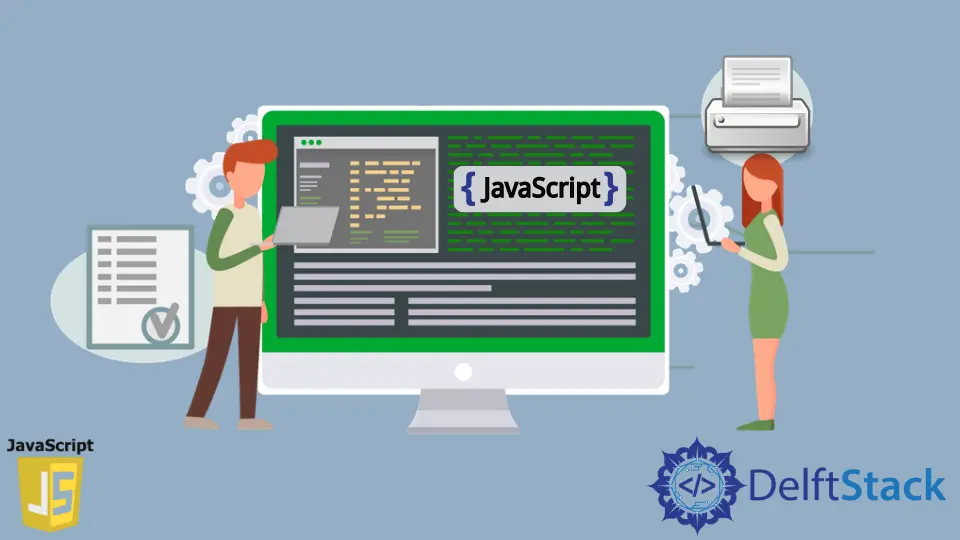
As a programmer, you always have to write a code that works that has fewer bugs in it. But the process of finding bugs and understanding how the code is executing is complicated when you have to work with n
lines of codes. So, to make this process easier, we can use something called a console. A console is a window used to print or show the output of the program and also used for testing purposes. All modern browsers support the console. You can open the browser console window by heading over to the Developer tools
section.
There are various methods provided by the JavaScript programming language which can be used for printing an output message on the console. All these methods can be accessed with the help of the console
object. Each method has there own purpose and can be used based on your requirements. Below are some of the console methods which many developers widely use.
console.log()
console.warn()
console.error()
console.info()
Let’s understand how to use each of these methods to print the message to the console in detail. Make sure that you have the console window open (if not, then press F12 or go to Developer Tools
) so that you can see the output for the below programs.
Print to Console With the console.log()
Method in JavaScript
It is the most popular and widely used console method in JavaScript. This method is commonly used to print various messages or calculations results on the console or even while debugging the code.
You have written a code that adds two numbers, and now you want to display the result of this operation on the console window; in this case, you can use the console.log()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<script>
var num_1 = 2, num_2 = 3;
console.log(num_1 + num_2);
</script>
</body>
</html>
This is how it will look like on the console window.
Print to Console With the console.warn()
Method in JavaScript
If you want to print warnings to the console, then that you can use this method. There can be cases where you want the user from doing a particular thing; then, you can use the console.warn()
method. For example, you create an application where tracks the user’s weight. If the weight of the user increases beyond 100, then you will show a warning to the console displaying that Do exercise and be healthy
using the console.warn()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<script>
var weight = Math.random() * (110-95) + 95;
if(Math.floor(weight) < 100){
console.warn("Do exercise and be healthy");
}
</script>
</body>
</html>
The output of the above code:
Print to Console With the console.error()
Method in JavaScript
Whenever you want to throw any error to the console, you can use the console.error()
method. For example, you write a code that generates a random number between 0
to 5
. Now here you want to throw an error message to the console when the random number generated is equal to zero, then you can use the console.error()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<script>
var min =0, max = 5;
var num = Math.random() * (max - min) + min;
if(Math.floor(num) === 0){
console.error("We don't accept zeros...");
}
</script>
</body>
</html>
If you run the above code, you will see an output in the console window.
Print to Console With the console.info()
Method in JavaScript
It is used to display the informational message to the console window. If you are creating a web application that provides weather updates to the user and gives some useful suggestions or information based on the current weather. These kinds of suggestions can be displayed into the console window as an informational message using the console.info()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<script>
console.info("The weather looks rainy today, you should take an umbrella with you.");
</script>
</body>
</html>
The above code will display the informational message The weather looks rainy today, you should take an umbrella with you.
as an output to the console.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn