How to Print PDF File in JavaScript
-
Print PDF File Using the
iframe
Tag in JavaScript -
Print PDF File Using the
Print.js
Library in JavaScript
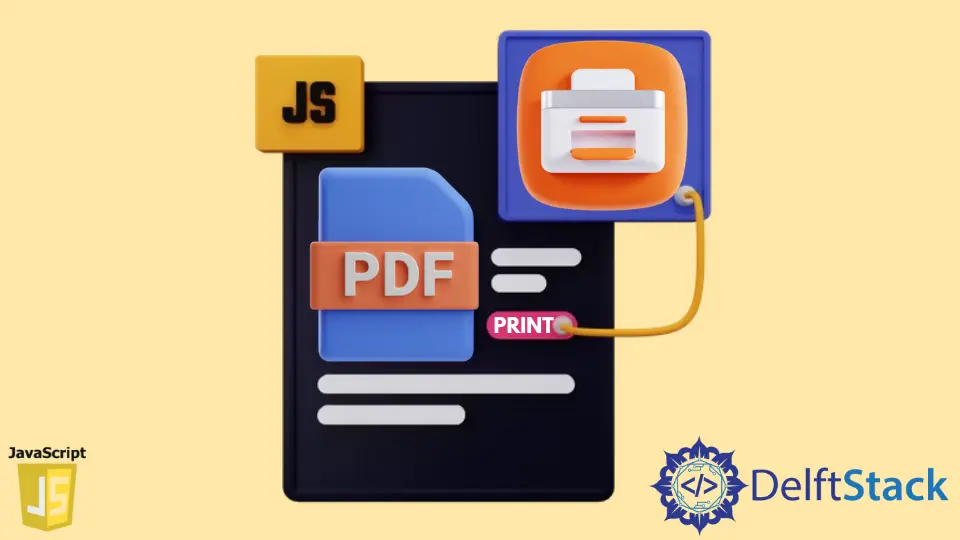
Many websites that are full of educational content are available on the Internet. The contents of these websites can either be in a written format (text) or a form of files mostly in PDF format (like eBooks).
If you directly want to download these PDF files from these websites, you can click on a button and do that. However, scenarios can occur, such as a user only wants to print those files rather than downloading them.
For this, you first have to download the PDF files, open them, and then print them.
However, what if the user can directly go to the print screen after clicking on a button? That would be a much better approach instead of telling the user to download the file, open it, and then print that file.
This process can be achieved in JavaScript by using an iframe
tag or a third-party JavaScript library like Print.js
.
Print PDF File Using the iframe
Tag in JavaScript
The iframe
HTML tag allows us to embed various contents, such as other websites and files inside our webpage.
The iframe
tag provides multiple attributes and methods that you can use in your website to take full advantage of this tag.
Let’s see how we can use this tag to print PDF files in JavaScript.
In our HTML document, we have to create a button inside the body
tag, and then we have to link our JavaScript file using the script
tag.
We will add an onclick
event, and then we will pass a function printPdf()
as a value to this event. We will define the printPdf()
inside JavaScript.
This function takes the PDF file path as a parameter. The path to the PDF file should be given correctly to open inside the print screen area.
<body>
<button onclick="printPdf('./test.pdf')">Print PDF file</button>
<script src="index.js"></script>
</body>
We have a printPdf()
function inside our JavaScript file that takes the PDF file path as an argument. In this function, we will first create an iframe
tag using the DOM API method document.createElement
.
The printPdf()
function will only be invoked after clicking the button. After clicking the button, the iframe
tag will display a rectangular box on the screen.
In the case of websites, it will embed the entire website inside that rectangular box, whereas in the case of a file, it will just show a blank screen that we don’t want.
Thus, we will hide the iframe
by setting the CSS property display
as none
inside our JavaScript file.
We will then set the src
attribute of the iframe
with the pdf
path, which we must send while calling the printPdf
function.
function printPdf(pdf) {
var iframe = document.createElement('iframe');
iframe.style.display = 'none';
iframe.src = pdf;
document.body.appendChild(iframe);
iframe.contentWindow.focus();
iframe.contentWindow.print();
}
Next, we can append the iframe
to the body element. We will then set the contentWindow
of the iframe to focus()
and print()
.
We will directly open the print screen in focus mode due to these methods of the contentWindow
.
Output:
Finally, after you click on the button we have created, you will see the above output.
If you don’t want to write a lot of code like what we have written so far, you can also use a third party like Print.js
to have print PDF functionality in JavaScript. This process is what we will be seeing in the upcoming section.
Print PDF File Using the Print.js
Library in JavaScript
The Print.js
is a tiny PDF library that allows you to open a PDF file in print mode. The main advantage of using a library is that it saves considerable time.
Instead of downloading the library in this tutorial, we will be using its CDN. Various ways can be applied to download and use the Print.js
library.
For more information on this, visit the Print.js documentation. Add the below two CDN links at the bottom of the body
tag.
https: // printjs-4de6.kxcdn.com/print.min.js
https: // printjs-4de6.kxcdn.com/print.min.css
We then have to create a button similar to how we have made it earlier. In this case, inside the onclick
, we have to use the printJS()
function that we obtained from the Print.js
library.
This function can either take a file path as an input or an entire object.
printJS('./test.pdf') // only file path
printJS({printable: './test-large.pdf', type: 'pdf'}) // an entire obj
You can choose any one of these approaches depending on your need. Ensure that you have added a PDF file inside your project folder or on the server.
Otherwise, the code will not work and can give you errors related to PDF.
The PDF files must be served on the same domain where your website is hosted. This is because Print.js
internally uses the iframe
tag and is limited by the Same Origin Policy.
<body>
<button type="button" onclick="printJS('./test.pdf')">
Print PDF
</button>
<script src="https://printjs-4de6.kxcdn.com/print.min.js"></script>
<script src="https://printjs-4de6.kxcdn.com/print.min.css"></script>
</body>
This library will generate the same output as the iframe
tag, as shown below. However, the amount of code that we have written is relatively few.
If the PDF file size is enormous, you can also show a modal so that the user will know that it might take some time to load the file. This process will provide a good user experience.
The modal will be shown after the user clicks the button and will disappear when the print mode appears. For this, just set the showModal
property to true
as follows.
printJS({printable: './test-large.pdf', type: 'pdf', showModal: true})
The modal will look like this.
As soon as the print mode is loaded, it will show you the print screen similar to what we have seen before.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn