How to Generate PDF in JavaScript
- Generate PDF in JavaScript
-
Use the
jsPDF
Library to Generate PDF in JavaScript -
Use the
html2pdf
Library to Generate PDF in JavaScript
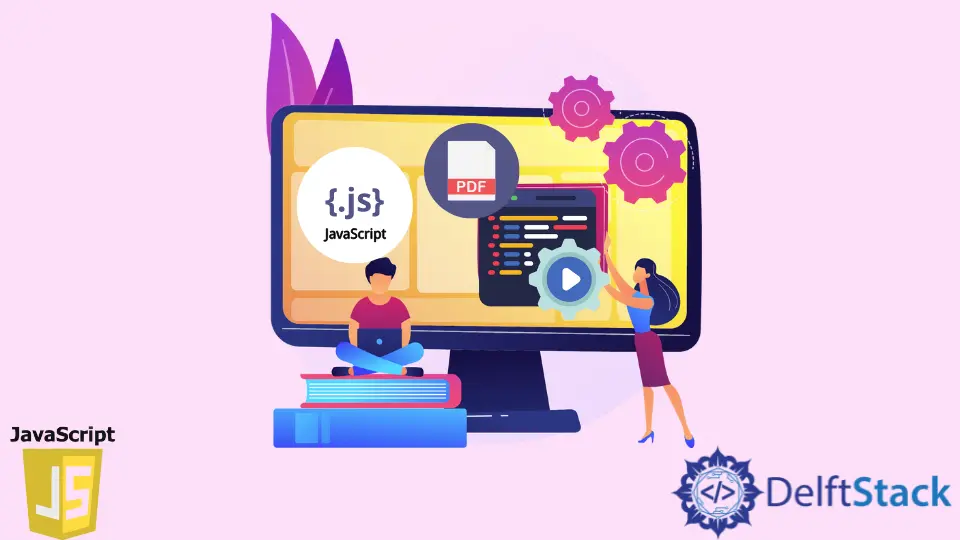
In this article, we will learn the best library for PDF creation in JavaScript source code and how we can use that library in our JavaScript code.
Generate PDF in JavaScript
We have often seen file download options on web pages such as PDFs. The developer must generate a PDF file using program code to provide this functionality to the user.
We can use multiple libraries in JavaScript to create a PDF file. For example, many websites provide the functionality to generate and download files like invoices, tickets, CVs etc.
These websites use different libraries to perform this functionality. We will discuss and learn the two most commonly used libraries with the help of examples.
Use the jsPDF
Library to Generate PDF in JavaScript
For JavaScript only, we have npm
library package jsPDF
to create PDF. There is no server-side scripting needed while using this library.
We can generate a PDF file by processing the dynamic content. It’s a quick and simple way to generate a PDF file in 3 lines of code, as shown below.
Basic Syntax:
var myDoc = new jsPDF(); // object
myDoc.text(10, 10, 'DelftStack Website!'); // content
myDoc.save('dummyFile.pdf'); // saving
We need to create an object and store it in a variable using the new
keyword and jsPDF()
. Then, we must set the content as a string with an object’s text
property.
Finally, we save the PDF file with the save
property.
Use the html2pdf
Library to Generate PDF in JavaScript
The html2pdf
library allows us to embed it in our website with JavaScript source and convert web page content into PDF document. The PDF document will be downloadable as PDF.
Just download the library and import it into our source before using it.
Basic Syntax:
const docElement = document.getElementById('ticket');
html2pdf().from(docElement).save();
As shown above we get the element content by document.getElementById()
and using html2pdf().from(docElement).save()
we can convert the content to PDF and download file.
Example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>HTML to PDF conversion</title>
<script src="html2pdf.bundle.min.js"></script>
<script>
function createPDF() {
// get the element of ticket content.
const docElement = document.getElementById('ticket');
// select the element and save as the PDF.
html2pdf().from(docElement).save();
}
</script>
</head>
<body>
<h1 style="color:blueviolet">DelftStack Learning</h1>
<h3>JavaScript create and download pdf</h3>
<div id="ticket">
<h1>Our Ticket</h1>
<p>Ticket content here</p>
</div>
<button onclick="createPDF()">Download as PDF</button>
</body>
</html>
Output:
In the above HTML source example, we downloaded html2pdf
library already, and imported in <head>
tags. We created a div
element for the ticket, defined the id ticket
to it, and provided dummy content to that div
element of HTML.
Then, there is a button element Download as PDF
on the click event of that button; we have called the function createPDF()
. Inside that function, first of all, we have the element by using document.getElementById('ticket')
and storing it in a variable.
Finally we used html2pdf
library method from()
and save()
. We just need to pass the element in from()
method as an argument and call save()
method.
Download this library, save the above source with the extension of HTML and open it in the browser to see the results.