How to Convert HTML to PDF in JavaScript
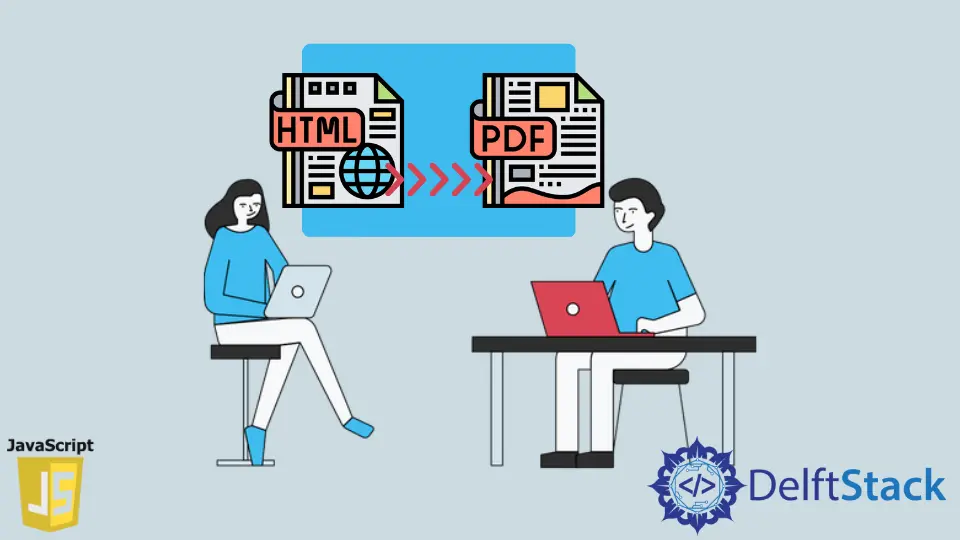
When downloading massive data from a web application, the PDF file format comes in handy. It enables users to download dynamic material as a file for offline consumption. HTML material is transformed to a PDF document and downloaded as a PDF file using the export to PDF capability. A server-side script converts HTML to PDF in the dynamic web application.
In this tutorial, we will perform such conversion using JavaScript.
Use the jsPDF
Library to Convert HTML to PDF
In this method, we will use jsPDF
library to convert HTML to PDF. It is one of the best libraries to use. The jsPDF
library offers several techniques and options for customizing PDF generation.
Check the code below.
- HTML
<!DOCTYPE html>
<html>
<body>
<div>
<p>Convert this text to PDF.</p>
</div>
<div id="hidden-element">This will not be printed</div>
</body>
</html>
- JavaScript
var source = window.document.getElementsByTagName('body')[0];
var specialElementHandlers = {
'#hidden-element': function(element, renderer) {
return true;
}
};
var doc = new jsPDF({orientation: 'landscape'});
doc.setFont('courier');
doc.setFontType('normal');
doc.setFontSize(24);
doc.setTextColor(100);
doc.fromHTML(
elementHTML, 15, 15,
{'width': 170, 'elementHandlers': specialElementHandlers});
The orientation
property sets the orientation of the paper. The setFont()
and setFontType()
option are used to set the font and font-style of the Text. The setFontSize()
and setTextColor()
functions are used to set font size and color of the text.
Use a Window Object to Convert HTML to PDF
In this method, we will create a window object, which we will use to create an associated document and write the HTML text in the file to export it as a PDF.
See the code below.
<html>
<head>
<title></title>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$("#btnPrint").live("click", function () {
var divContents = $("#text").html();
var printWindow = window.open('', '', 'height=400,width=800');
printWindow.document.write('<html><head><title>Html to PDF</title>');
printWindow.document.write('</head><body >');
printWindow.document.write(text);
printWindow.document.write('</body></html>');
printWindow.document.close();
printWindow.print();
});
</script>
</head>
<body>
<form id="form1">
<div id="text">
Convert this text to PDF.
</div>
<input type="button" value="Print Div Contents" id="btnPrint" />
</form>
</body>
</html>
In the above example, the window.open()
function will open a document with the specified dimensions, and document.write()
method is used to write text in the opened document.