在 JavaScript 中將 HTML 轉換為 PDF
Kushank Singh
2023年10月12日
JavaScript
JavaScript PDF
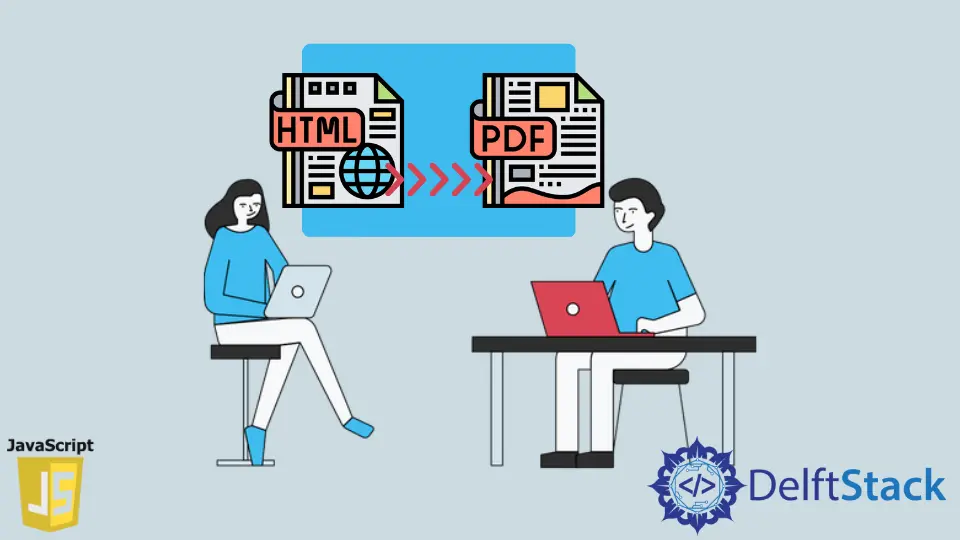
從 Web 應用程式下載大量資料時,PDF 檔案格式會派上用場。它使使用者能夠將動態素材下載為檔案以供離線使用。HTML 材料轉換為 PDF 文件,並使用匯出為 PDF 功能下載為 PDF 檔案。伺服器端指令碼在動態 Web 應用程式中將 HTML 轉換為 PDF。
在本教程中,我們將使用 JavaScript 執行此類轉換。
使用 jsPDF
庫將 HTML 轉換為 PDF
在此方法中,我們將使用 jsPDF
庫將 HTML 轉換為 PDF。它是最好的庫之一。jsPDF
庫提供了多種自定義 PDF 生成的技術和選項。
參考下面的程式碼。
- HTML
<!DOCTYPE html>
<html>
<body>
<div>
<p>Convert this text to PDF.</p>
</div>
<div id="hidden-element">This will not be printed</div>
</body>
</html>
- JavaScript
var source = window.document.getElementsByTagName('body')[0];
var specialElementHandlers = {
'#hidden-element': function(element, renderer) {
return true;
}
};
var doc = new jsPDF({orientation: 'landscape'});
doc.setFont('courier');
doc.setFontType('normal');
doc.setFontSize(24);
doc.setTextColor(100);
doc.fromHTML(
elementHTML, 15, 15,
{'width': 170, 'elementHandlers': specialElementHandlers});
orientation
屬性設定紙張的方向。setFont()
和 setFontType()
選項用於設定文字的字型和字型樣式。setFontSize()
和 setTextColor()
函式用於設定文字的字型大小和顏色。
使用 window 物件將 HTML 轉換為 PDF
在此方法中,我們將建立一個視窗物件,我們將使用該物件建立關聯文件並在檔案中寫入 HTML 文字以將其匯出為 PDF。
看下面的程式碼
<html>
<head>
<title></title>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$("#btnPrint").live("click", function () {
var divContents = $("#text").html();
var printWindow = window.open('', '', 'height=400,width=800');
printWindow.document.write('<html><head><title>Html to PDF</title>');
printWindow.document.write('</head><body >');
printWindow.document.write(text);
printWindow.document.write('</body></html>');
printWindow.document.close();
printWindow.print();
});
</script>
</head>
<body>
<form id="form1">
<div id="text">
Convert this text to PDF.
</div>
<input type="button" value="Print Div Contents" id="btnPrint" />
</form>
</body>
</html>
在上面的例子中,window.open()
函式將開啟一個指定尺寸的文件,document.write()
方法用於在開啟的文件中寫入文字。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe