How to Override a Function in JavaScript
- Override Custom Functions in JavaScript
- Override Built-In Functions in JavaScript
- Try to Overload a Function in JavaScript
-
Use the
super
Keyword in Inheritance to Get Back Previous Function
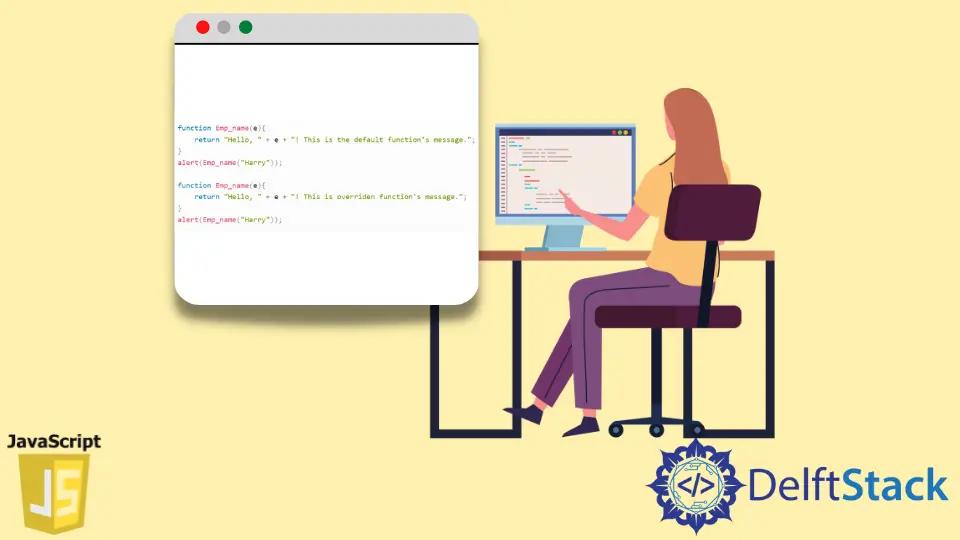
In this tutorial article, we will introduce how to override a function in JavaScript. We will also go through how the JavaScript interpreter behaves when we overload a function or use it with inheritance.
JavaScript does not support overloading. But it allows overriding functions. In the case of declaring two functions with the same name and parameters, JavaScript considers the latest function while interpreting. This is how to override a function in JavaScript.
Override Custom Functions in JavaScript
We will create two custom functions with the same name, Emp_name
, to display the employee’s name with different alert()
messages.
Example code without override function:
function Emp_name(e) {
return 'Hello, ' + e + '! This is the default function\'s message.';
}
alert(Emp_name('Harry'));
Example code with override function:
function Emp_name(e) {
return 'Hello, ' + e + '! This is the default function\'s message.';
}
alert(Emp_name('Harry'));
function Emp_name(e){
return "Hello, " + e + "! This is overriden function's message.";
}
alert(Emp_name("Harry"));
Override Built-In Functions in JavaScript
JavaScript also allows overriding built-in functions in the same way. When we change the code block of a function with the same name as that of a predefined function, it overrides the default function and changes its code to the new one. We will use the Date()
function and override it. By default, the Date()
function displays date, day, and local time. We will override it to display an alert()
message.
Example code:
var d = new Date();
document.write(d);
function Date() {
return 'This is the overriden function.';
}
alert(Date());
Try to Overload a Function in JavaScript
JavaScript does not allow overloading a function. Instead, it will override the function. We will create two functions named sum
to add different numbers of parameters.
function sum(i, j, k) {
return i + j + k;
}
function sum(i, j) {
return i + j;
}
alert('Sum of i+j+k is: ' + sum(4, 5, 8));
alert('Sum of i+j is: ' + sum(4, 5));
The output will show the addition for only the first two parameters for both functions. This is because function overloading is not allowed. Hence, the interpreter will consider the latest function and the parameters it defines. All the additional parameters will be ignored.
Use the super
Keyword in Inheritance to Get Back Previous Function
The only way to get back the previous function once we override it is to use the super
keyword. We will create two functions with the same name and parameters, one in the parent class and one in the child class. We will use the super
keyword to access the parent class function even after overriding it.
class Parent {
msg() {
document.write('This is parent class msg.<br>');
}
}
class Child extends Parent {
msg() {
super.msg();
document.write('This is child class msg.');
}
}
let p = new Parent();
let c = new Child();
p.msg();
c.msg();
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn