JavaScript it() Function
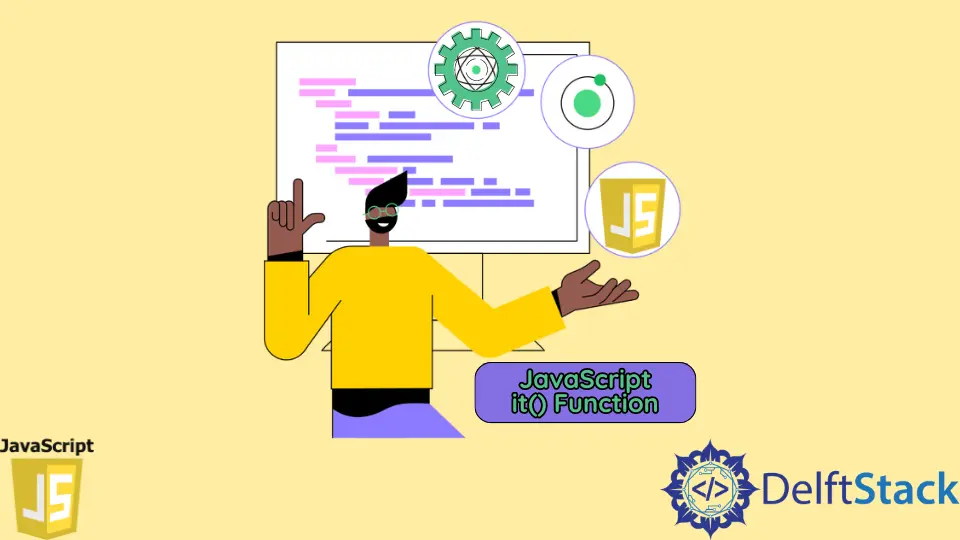
In JavaScript, we have many frameworks dealing with individual concerns, and Jasmine
is one of those many. It is a unit testing framework, and it has its documentation already packed up.
One of the functions of this framework is the it()
function that enables the user/admin to get the total outcome of the code lines.
Generally, we have to tackle piles of codes, and it is near impossible to check up on each line for debugging. However, we know the output regardless of how large the code segments are.
So, the target is to use this it()
function to declare the expected output and have a fair check if it matches the coded conditions. We will cover the basic setup and its work in the next section.
Use the JavaScript it()
Function
We will have to set up some configurations in our local PC to enable the Jasmine
framework here. We will check a number and expect
to receive the number as a number
type.
But if the number input is a string, it will cause an error. Initially, we will consider okay.html
and okay.jss
.
We will set directory jasmine
, and inside, we will keep okay.html
and another folder src
containing the okay.jss
.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jasmine/4.1.0/jasmine.min.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jasmine/4.1.0/jasmine.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jasmine/4.1.0/boot0.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jasmine/4.1.0/jasmine-html.min.js"></script>
<title>JS Bin</title>
</head>
<body>
<script src="./src/okay.js">
</script>
</body>
</html>
let year = '2022';
describe('What\'s the year?', () => {
it('Should be a number', () => {
expect(year).toBe(2022);
});
});
We will open Visual Studio Code
for the jasmine
directory and directly go to the terminal. From the documentation for Jasmine
, we will follow this thread and grab the necessary command lines.
We will set jasmine
for the node.js
and the server.
We will start typing in the terminal npm install
. By doing so, you will have a package.json
and a package-lock.json
file.
However, if you do not find the package.json
file, then visit this unpacker and paste the contents of package-lock.json
in the editor of the unpacker, and press unpack
. Next, create a package.json
file in the root directory and paste the contents you received from the unpacker.
In the further step, we will use these commands in the terminal.
npm install --save-dev jasmine
npm install --save-dev jasmine-browser-runner jasmine-core
npx jasmine init
npx jasmine-browser-runner init
After executing these, you will have additional dependencies in your pacakge.json
file. Now, add this object to your package.json
file and save it.
'scripts': {'test': 'jasmine-browser-runner runSpecs'}
So, now overall, your file tree will look something like this, and the package.json
will look like the below.
File Tree:
node_modules
spec
src
okay.jss
okay.html
package.json
package-lock.json
package.json
:
{
"name": "converted",
"version": "1.0.0",
"description": "",
"author": "",
"license": "ISC",
"dependencies": {
"concat-map": "0.0.1",
"jasmine": "4.1.0"
},
"devDependencies": {
"jasmine-browser-runner": "^1.0.0",
"jasmine-core": "^4.1.0"
},
"scripts": {
"test": "jasmine-browser-runner runSpecs"
}
}
Now, type npx jasmine-browser-runner serve
in the terminal, and port 8888
will showcase the test cases. Let’s check the output.
Output:
It can be seen that the expected output was a number type, but we received a string type. Thus, it showed a failure
, and now we will see a correct version and examine what happens.
Code Snippet:
let year = 2022;
describe('What\'s the year?', () => {
it('Should be a number', () => {
expect(year).toBe(2022);
});
});
Output:
So, we can infer how the it()
function works and draws out the expected output.