How to Init Functions in JavaScript
- Understanding Function Initialization
- Function Declaration
- Function Expression
- Arrow Functions
- Immediately Invoked Function Expressions (IIFE)
- Conclusion
- FAQ
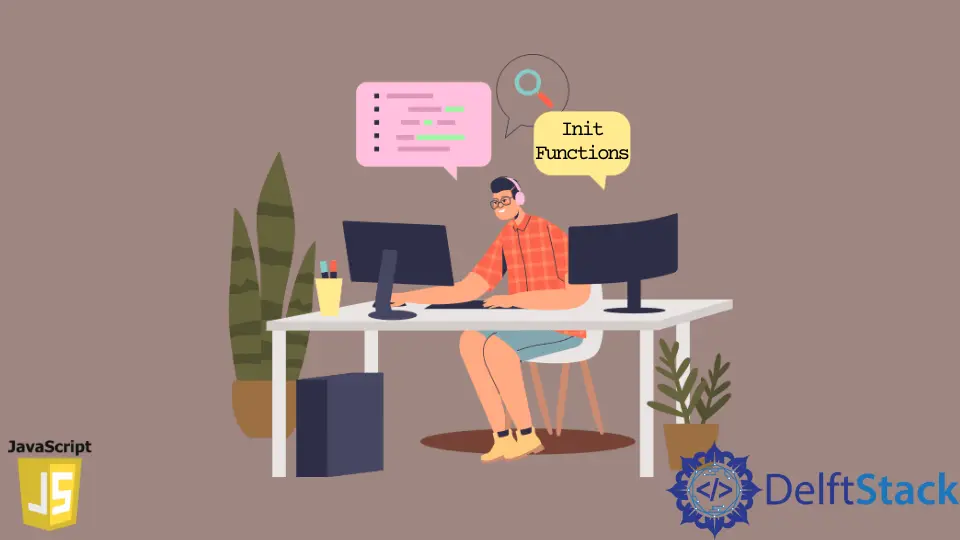
In today’s post, we’ll learn how to init functions in JavaScript. JavaScript is a versatile programming language that enables developers to create dynamic and interactive web applications. One of the essential building blocks of JavaScript is the function. Functions allow you to encapsulate code for reuse, making your scripts cleaner and more efficient. Whether you’re just starting with JavaScript or looking to refine your skills, understanding how to initialize functions is crucial.
In this article, we’ll explore various methods to define and invoke functions in JavaScript, along with practical examples and explanations. Let’s dive in!
Understanding Function Initialization
Before we jump into different methods of initializing functions, let’s clarify what it means to “init” a function in JavaScript. Function initialization refers to the process of defining a function and making it ready for execution. This can be done in several ways, each with its own syntax and use cases. Here are some common methods to initialize functions in JavaScript.
Function Declaration
The function declaration is one of the most straightforward ways to define a function in JavaScript. It starts with the function
keyword, followed by the function name, parentheses, and curly braces. This method is hoisted, meaning you can call the function before its declaration in the code.
Here’s how you can declare a function:
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice"));
Output:
Hello, Alice!
In this example, we defined a function named greet
that takes one parameter, name
. When we call greet("Alice")
, it returns a greeting string. The flexibility of function declarations allows you to organize your code efficiently, making it easy to read and maintain.
Function Expression
Another way to initialize a function in JavaScript is through a function expression. Unlike function declarations, function expressions are not hoisted. This means you cannot call them before they are defined. A function expression can be anonymous or named, and it’s often assigned to a variable.
Here’s an example of a function expression:
const add = function(x, y) {
return x + y;
};
console.log(add(5, 3));
Output:
8
In this code snippet, we assigned an anonymous function to the variable add
. When we invoke add(5, 3)
, it returns the sum of the two numbers. Function expressions are particularly useful when you want to pass functions as arguments or return them from other functions.
Arrow Functions
Introduced in ES6, arrow functions provide a concise syntax for writing function expressions. They are especially popular for their brevity and the fact that they do not have their own this
context, making them suitable for certain use cases like callbacks.
Here’s how you can create an arrow function:
const multiply = (a, b) => a * b;
console.log(multiply(4, 7));
Output:
28
In this example, we defined an arrow function named multiply
that takes two parameters and returns their product. Arrow functions can make your code cleaner and more readable, especially when dealing with short functions.
Immediately Invoked Function Expressions (IIFE)
An Immediately Invoked Function Expression (IIFE) is a function that runs as soon as it is defined. This pattern is useful for encapsulating code and avoiding polluting the global scope. An IIFE is defined as a function expression that is immediately invoked.
Here’s how to create an IIFE:
(function() {
console.log("This function runs immediately!");
})();
Output:
This function runs immediately!
In this example, the function is defined and then executed right away. IIFEs are particularly useful for creating private variables or functions that you don’t want to expose to the global scope, thus maintaining a clean environment.
Conclusion
Understanding how to initialize functions in JavaScript is fundamental for any developer looking to write efficient and maintainable code. From function declarations to arrow functions and IIFEs, each method serves a specific purpose and can be used based on the needs of your application. As you continue to explore JavaScript, mastering these techniques will enhance your coding proficiency and allow you to create more dynamic web applications. So, get out there, experiment with these function initialization methods, and elevate your JavaScript skills!
FAQ
-
What is a function declaration in JavaScript?
A function declaration is a way to define a function using thefunction
keyword, followed by the function name and parameters. -
What are arrow functions?
Arrow functions are a concise way to write function expressions in JavaScript, introduced in ES6, and they do not have their ownthis
context. -
What is an IIFE?
An Immediately Invoked Function Expression (IIFE) is a function that runs as soon as it is defined, helping to avoid polluting the global scope. -
Are function declarations hoisted in JavaScript?
Yes, function declarations are hoisted, meaning you can call them before their actual definition in the code. -
Can you assign a function to a variable in JavaScript?
Yes, you can assign a function to a variable using a function expression, allowing you to invoke it later through that variable.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn