How to Exit JavaScript Function
- Understanding Function Exits in JavaScript
- Using the Return Statement
- Leveraging Exceptions
- Utilizing Control Flow Statements
- Conclusion
- FAQ
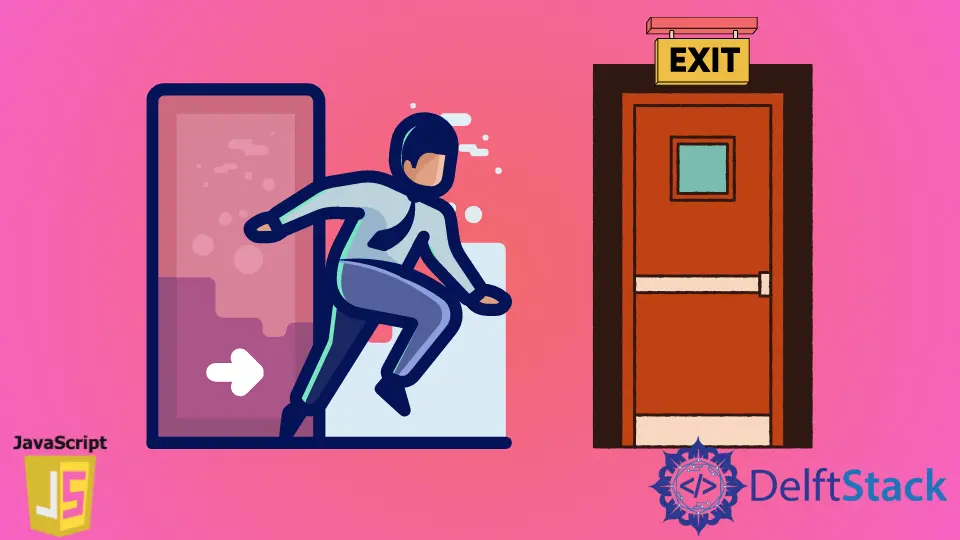
In the world of JavaScript programming, knowing how to exit a function early is a crucial skill. Whether you’re dealing with complex logic or simply want to streamline your code, understanding how to exit functions can lead to cleaner and more efficient code.
This tutorial will guide you through various methods to exit a JavaScript function early, ensuring that your code runs smoothly and as intended. We will cover different scenarios where exiting a function can be beneficial, and provide clear examples to illustrate each method. By the end of this article, you’ll have a solid grasp of how to manage function exits effectively in your JavaScript projects.
Understanding Function Exits in JavaScript
When you’re writing functions in JavaScript, there may be instances where you want to terminate the function’s execution before it reaches the end. This could be due to a specific condition not being met, or perhaps an error has occurred that makes further execution unnecessary. Exiting a function early can help avoid unnecessary computations and improve the overall performance of your code.
There are several ways to exit a function in JavaScript:
- Using the return statement
- Leveraging exceptions
- Utilizing control flow statements
Let’s delve into each method in detail.
Using the Return Statement
The most straightforward way to exit a function in JavaScript is by using the return statement. When JavaScript encounters a return statement, it immediately stops executing the function and returns control to the caller. This method is particularly useful when you want to return a value or simply terminate the function based on certain conditions.
Here’s a simple example:
function checkAge(age) {
if (age < 18) {
return "Access denied";
}
return "Access granted";
}
const result = checkAge(16);
console.log(result);
Output:
Access denied
In this example, the checkAge
function checks if the provided age is less than 18. If it is, the function immediately exits and returns “Access denied”. If the age is 18 or older, it continues and returns “Access granted”. This showcases how the return statement can effectively control the flow of execution within a function.
Leveraging Exceptions
Another method to exit a function in JavaScript is by throwing an exception. This approach is particularly useful when dealing with error handling. When an exception is thrown, the function’s execution stops, and control is passed to the nearest catch block in the call stack. This can be a powerful way to manage unexpected situations in your code.
Here’s an example:
function validateInput(input) {
if (input === "") {
throw new Error("Input cannot be empty");
}
return "Valid input";
}
try {
const result = validateInput("");
console.log(result);
} catch (error) {
console.error(error.message);
}
Output:
Input cannot be empty
In this code, the validateInput
function checks if the input is an empty string. If it is, it throws an error, effectively terminating the function. The error is then caught in the try-catch block, allowing you to handle it gracefully. This method not only exits the function but also provides a way to manage errors effectively.
Utilizing Control Flow Statements
Control flow statements like if-else or switch-case can also be used to exit a function under specific conditions. By structuring your code with these statements, you can dictate when a function should terminate its execution. This method is particularly effective when dealing with multiple conditions.
Here’s an example:
function processOrder(order) {
if (order.status === "cancelled") {
return "Order has been cancelled";
} else if (order.status === "pending") {
return "Order is still pending";
}
return "Order processed successfully";
}
const orderResult = processOrder({ status: "cancelled" });
console.log(orderResult);
Output:
Order has been cancelled
In this example, the processOrder
function checks the status of an order. If the status is “cancelled”, it exits early with a specific message. If the order is still pending, it exits with a different message. Only if the order is processed successfully does it reach the end of the function. This method allows for clear and concise handling of different scenarios within a single function.
Conclusion
Exiting a JavaScript function early is a fundamental concept that can significantly enhance the efficiency and readability of your code. Whether you utilize the return statement, throw exceptions, or employ control flow statements, understanding how to manage function exits allows you to create more robust applications. By mastering these techniques, you can ensure that your functions execute optimally and handle various conditions gracefully. Armed with this knowledge, you are now better equipped to write cleaner, more effective JavaScript code.
FAQ
-
What is the return statement in JavaScript?
The return statement is used to stop the execution of a function and return a value to the caller. -
How can I handle errors in JavaScript functions?
You can handle errors by throwing exceptions and using try-catch blocks to manage them gracefully. -
Can I exit a function without returning a value?
Yes, you can exit a function without returning a value by simply using the return statement without any value. -
What are control flow statements in JavaScript?
Control flow statements, such as if-else and switch-case, are used to dictate the flow of execution in your code based on specific conditions. -
Why is it important to exit functions early?
Exiting functions early can improve code performance, reduce unnecessary computations, and enhance overall readability.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn