JavaScript Default Function Parameters
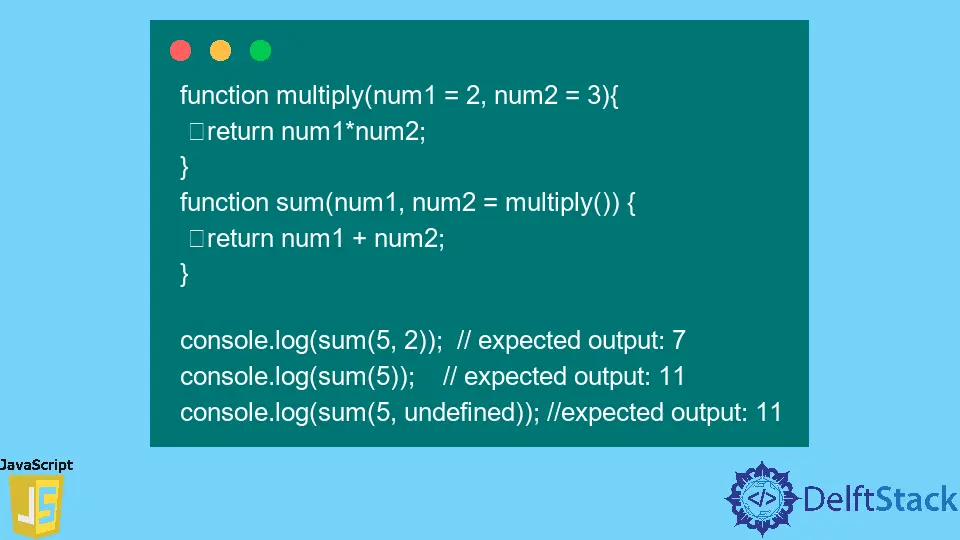
This article enlightens JavaScript default function parameters that allow the named parameters to be initialized with default values (if actual parameters are not passed or undefined
is passed). We will learn the difference between named parameters & actual parameters and their use.
Actual parameters are the actual values passed to the function by its caller. Named parameters use names for the actual parameters. These names are associated with the formal parameters in the function definition.
The actual parameters are labeled within the function body. The order does not matter for named parameters as long as they are correctly labeled.
JavaScript Default Function Parameters
In the following code, the value of num2
would be two (2) if no value is passed (or undefined
is passed) while calling the sum()
function.
function sum(num1, num2 = 2) {
return num1 + num2;
}
console.log(sum(10, 3)); // expected output: 13
console.log(sum(3)); // expected output: 5
console.log(sum(3, undefined)); // expected output: 5
Now, what if we don’t use JavaScript default function parameters. See the example given below.
function sum(num1, num2) {
return num1 + num2;
}
console.log(sum(10, 3)); // expected output: 13
console.log(sum(3)); // expected output: NaN
console.log(sum(3, undefined)); // expected output: NaN
We can use the following technique to avoid NaN
(Not a Number). The typeof
checks if the value or type of num2
is undefined
, then the value of num2
would be 1
. typeof
allows you to pass anything including null
and false
.
function sum(num1, num2) {
num2 = (typeof num2 !== 'undefined') ? num2 : 1
return num1 + num2;
}
console.log(sum(5, 2)); // expected output: 7
console.log(sum(5)); // expected output: 6
Using ES2015, it is not necessary to tell the default parameters in the function body. Now, we can assign default values within the function definition. Check the following sample code.
function sum(num1, num2 = 1) {
return num1 + num2;
}
console.log(sum(5, 2)); // expected output: 7
console.log(sum(5)); // expected output: 6
console.log(sum(5, undefined)); // expected output: 6
We can also call another function as the default value. Have a look at the following example.
function multiply(num1 = 2, num2 = 3) {
return num1 * num2;
}
function sum(num1, num2 = multiply()) {
return num1 + num2;
}
console.log(sum(5, 2)); // expected output: 7
console.log(sum(5)); // expected output: 11
console.log(sum(5, undefined)); // expected output: 11
Let’s practice by using the string type data.
function greetings(
name, greeting, message = greeting + ' ' + name + ', How are you?') {
return message;
}
console.log(greetings('Mehvish', 'Hello'));
// expected output: "Hello Mehvish, How are you?"
console.log(greetings('Mehvish', 'Hello', 'What\'s up'));
// expected output: "What's up"
We have studied default function parameters using numbers, strings, and functions. Let’s understand how to set default values using the destructuring assignment. But before that, have a look at what destructuring assignment exactly is?
const person = {
firstname: 'Mehvish',
lastname: 'Ashiq',
age: 30,
email: 'delfstack@example.com'
};
const {firstname, lastname, age, email} = person
console.log(firstname);
console.log(lastname);
console.log(email);
Output:
"Mehvish"
"Ashiq"
"delfstack@example.com"
You may have noticed that we are directly using the object’s properties in console.log
rather than objectname.propertyname
. If you comment the line const {firstname,lastname,age,email} = person
, you have to use person.firstname
, person.lastname
, person.email
for all console.log
statements.
So, what the following line of code is doing? It is a destructuring assignment which means unpacking the object. Right now, we have minimal properties in the person
object.
Just think, we have hundreds of properties in an object and use objectname.propertyname
every time. It will look messy. Here, unpacking the object is useful.
With that, we get rid of objectname.
part and use propertyname
only.
const {firstname, lastname, age, email} = person;
Let’s use this destructuring assignment for JavaScript default function parameters.
function displayPerson(lastname, firstname, email = 'mehvish.ashiq@gmail.com') {
if (typeof email !== 'undefined') {
console.log('not using default value of email');
console.log(firstname, lastname, email);
} else {
console.log('using default value of email');
console.log(firstname, lastname, email);
}
}
const person = {
firstname: 'Mehvish',
lastname: 'Ashiq',
age: 30,
email: 'delfstack@example.com'
};
const {firstname, lastname, age, email} =
person // destructure assignment (unpack the object)
displayPerson(age, firstname, email);
displayPerson(
age,
firstname); // default value of email will be used in this function call
Output:
"not using default value of email"
"Mehvish", 30, "delfstack@example.com"
"not using default value of email"
"Mehvish", 30, "mehvish.ashiq@gmail.com"
In this example, the default value of email
will be used if the caller does not pass it.