How to Encode and Decode a String to Base64 in JavaScript
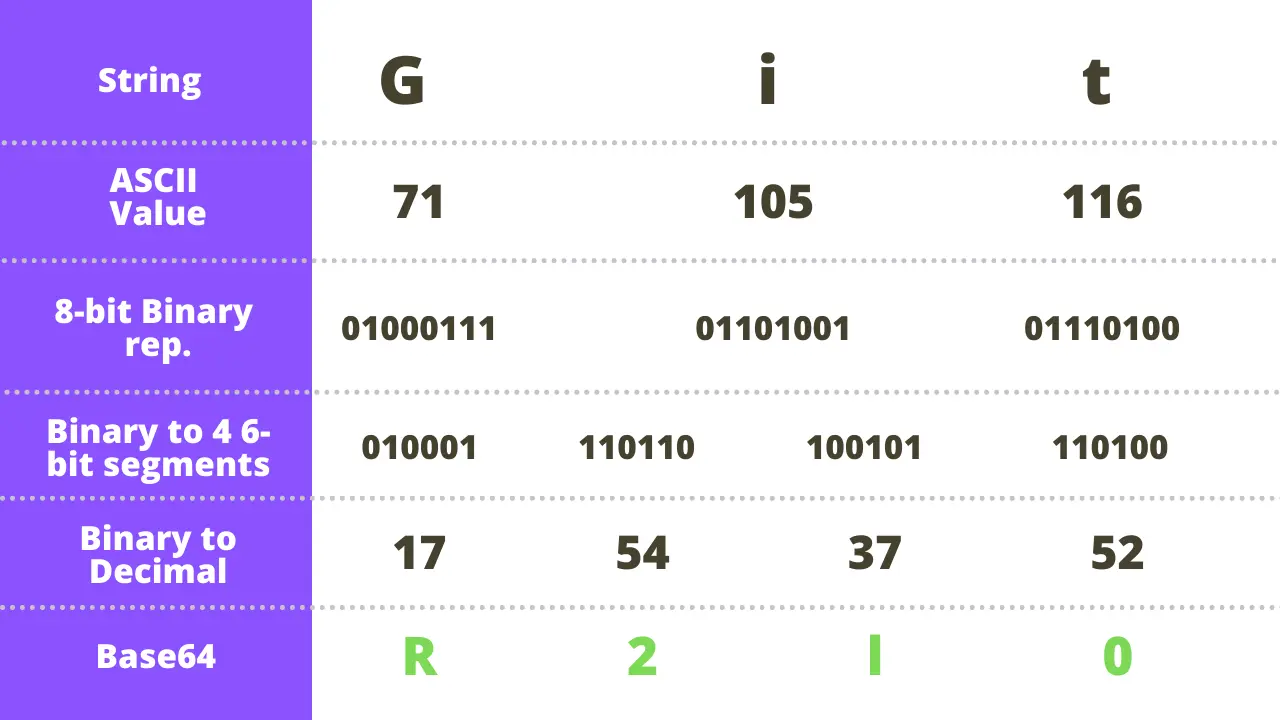
The Base64 encoding character set consist of A-Z
, a-z
, 0-9
, +
and /
. In total, there are 64 characters in this system, and therefore it is known as Base64. The Base64 encoding converts data into ASCII (American Standard Code for Information Interchange) format.
For Example, If you have a string Git, then in Base64 encoding format, this string will be represented as R29vZ2xl
.
What just happened here is that the individual characters of the string are first represented into their ASCII value i.e, for capital G
, its ASCII value is 71
, for small i
, its ASCII value is 105
, and similarly for other characters as well. To get a full list of ASCII values, visit this link.
Then these ASCII values are converted into an 8-bit binary representation. This 8-bit binary representation is then divided into four 6-bit segments. These four binary segments are then converted into decimals.
In the end, these decimal numbers are being converted into Base64 by following the Base64 encoding table.
Now we don’t have to do all of this conversion manually. Till now, what we have seen is just for understanding purposes. Let’s see how to encode and decode a string into Base64.
Encoding and Decoding a String to Base64 in JavaScript
In JavaScript, we have two Base64 helper functions, btoa()
and atob()
, for converting a string into Base64 encoding and vice-versa. The btoa()
takes a string and encodes it to Base64, and atob()
takes an encoded string and decodes it.
In the below code, we have two div
elements inside which we will display the encoded and decoded string. We are storing the references of these two div
inside myDiv_1
and myDiv_2
using getElementById()
method in JavaScript. The string we will encode and decode in this example is Git stored inside the str
variable.
To encode this string, we will use the btoa()
function. For this, just pass the str
variable as a parameter to this function. We will store the result of this inside the encodedStr
variable. Then we will display the value of this variable inside the div_1
using myDiv_1.innerHTML
property.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<div id="div_1"></div>
<div id="div_2"></div>
<script>
let myDiv_1 = document.getElementById('div_1');
let myDiv_2 = document.getElementById('div_2');
let str = "Git";
let encodedStr = btoa(str);
myDiv_1.innerHTML = encodedStr;
</script>
</body>
</html>
Output:
R2l0
This is what you will get as an output. The string is not being converted into Base64.
Now to decode the above string into its original form you can use the atob()
function and pass the encodedStr
inside it. Then display the value of decodedStr
variable inside the div_2
using myDiv_2.innerHTML
property.
let decodedStr = atob(encodedStr);
myDiv_2.innerHTML = decodedStr;
Output:
Git
Make a note that Base64 is used for sending binary data and is not an encryption method. Also, it is not a compression method.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn