Global and Private Functions in JavaScript
- Implement Global and Private Functions in JavaScript
-
Implement
Global
andPrivate
Functions in JavaScript Classes
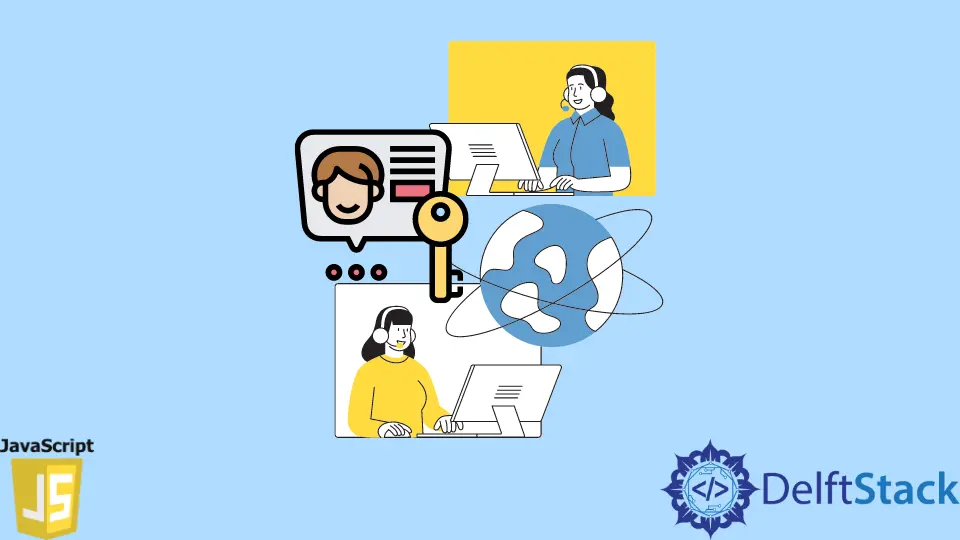
The functions that can be called globally are known as global functions, while we can’t invoke the private functions directly outside the class/module.
Throughout this article, we’ll use three files: index.html
, fileOne.js
, and fileTwo.js
.
The startup code for the index.html
is given below because it will remain the same throughout this article.
index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="./fileOne.js"></script>
<script src="./fileTwo.js"></script>
<title>Document</title>
</head>
<body>
<button id="greet" onclick="greetings()">Display Greetings</button>
<p id="displayGreet"></p>
</body>
</html>
Implement Global and Private Functions in JavaScript
If we write a function directly in a JavaScript file, it would be restricted to the window
object, global. It means the function will also be global.
fileOne.js
:
function displayName() {
let firstName = 'Mehvish';
let lastName = 'Ashiq';
return firstName + ' ' + lastName;
}
fileTwo.js
:
function greetings() {
document.getElementById('displayGreet').innerHTML = 'Hi! ' + displayName();
}
The greetings()
function would be called as soon as we click on the Display Greetings
button, and it calls the displayName()
directly, which is defined in the fileOne.js
file because it is a global function.
It gets the value returned by the displayName()
and change the innerHTML
of an element whose id is displayGreet
.
Think of private functions and make the displayName()
function private. To do that, we can create an object that will contain this function as follows.
fileOne.js
:
myfunctions = {
displayName: function displayName() {
let firstName = 'Mehvish';
let lastName = 'Ashiq';
return firstName + ' ' + lastName;
}
// you can add more functions here
}
Now, the question is how to call it in fileTwo.js
because displayName()
is a private function now? We can invoke it as myfunctions.displayName()
.
fileTwo.js
:
function greetings() {
document.getElementById('displayGreet').innerHTML =
'Hi! ' + myfunctions.displayName();
}
Implement Global
and Private
Functions in JavaScript Classes
Suppose we have a class named oneClass
in fileOne.js
. How can we make displayName()
private and global now?
fileOne.js
:
class oneClass {
constructor() {}
displayName = function displayName() {
let firstName = 'Mehvish';
let lastName = 'Ashiq';
return firstName + ' ' + lastName;
}
// you can write more functions
}
fileTwo.js
:
function greetings() {
const one = new oneClass();
document.getElementById('displayGreet').innerHTML =
'Hi! ' + one.displayName();
}
Here, we create an instance of class oneClass
and save its reference in one
.
We can call displayName()
directly via using one
instance class object because the displayName()
is a public function also known as global function.
fileOne.js
:
class oneClass {
constructor() {}
#displayName = function displayName() {
let firstName = 'Mehvish';
let lastName = 'Ashiq';
return firstName + ' ' + lastName;
} getDisplayName() {
return this.#displayName();
}
// you can write more functions
}
fileTwo.js
:
function greetings() {
const one = new oneClass();
document.getElementById('displayGreet').innerHTML =
'Hi! ' + one.getDisplayName();
}
Here, we use the #
symbol to denote that this particular function is private, and we know we can’t access it directly.
We will write another function getDisplayName()
inside the class that can access #displayName
.
In this way, we get the value of #displayName
via getDisplayName()
in fileTwo.js
. You can find more here in detail.