Nested Functions in JavaScript
- What are Nested Functions?
- Advantages of Using Nested Functions
- When to Use Nested Functions
- Best Practices for Nested Functions
- Conclusion
- FAQ
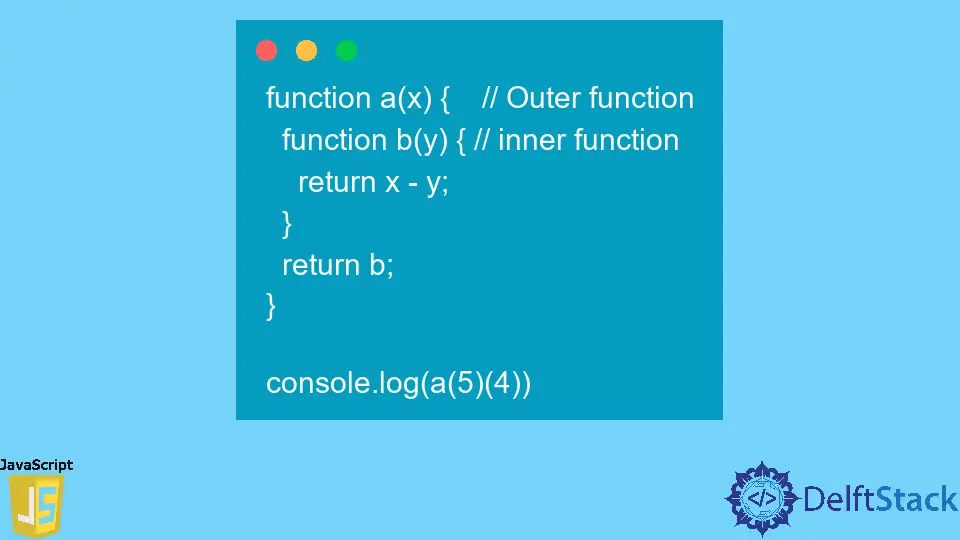
JavaScript is a versatile language that allows developers to create complex functionalities with ease. One of the powerful features it offers is the ability to use nested functions.
This tutorial demonstrates nested functions in JavaScript, shedding light on how they work and how you can leverage them to write cleaner, more efficient code. Whether you’re a beginner or an experienced developer, understanding nested functions can enhance your coding skills and improve your JavaScript applications. Let’s dive into the world of nested functions and explore their syntax, use cases, and benefits.
What are Nested Functions?
Nested functions are simply functions defined within another function. This structure allows the inner function to access variables and parameters of the outer function, creating a closure. Closures are a fundamental concept in JavaScript that enable data encapsulation and provide a way to maintain state in an elegant manner.
Here’s a simple example to illustrate nested functions:
function outerFunction(outerVariable) {
function innerFunction(innerVariable) {
return outerVariable + innerVariable;
}
return innerFunction;
}
const addFive = outerFunction(5);
const result = addFive(10);
Output:
15
In this example, outerFunction
takes a parameter called outerVariable
. Inside it, we define innerFunction
, which takes another parameter called innerVariable
. The inner function can access the outerVariable
because of the closure created by the outer function. When we call outerFunction(5)
, it returns the innerFunction
, which we assign to addFive
. Calling addFive(10)
returns 15
, demonstrating how nested functions can work together.
Advantages of Using Nested Functions
There are several advantages to using nested functions in your JavaScript code. First, they help in organizing code logically. By encapsulating functionality, you can keep related code together, making it easier to read and maintain. Secondly, nested functions allow for data privacy. The inner function can access variables from the outer function without exposing them to the global scope, reducing the risk of variable collisions. Lastly, nested functions can improve performance by minimizing the number of global variables and functions, leading to cleaner and more efficient code execution.
Consider this example:
function createCounter() {
let count = 0;
return function() {
count += 1;
return count;
};
}
const counter = createCounter();
console.log(counter());
console.log(counter());
Output:
1
2
Here, createCounter
defines a local variable count
and returns an inner function that increments and returns count
. Each time we call counter()
, it maintains its own state, demonstrating how nested functions can encapsulate data effectively.
When to Use Nested Functions
Knowing when to use nested functions can significantly enhance your JavaScript programming. You might consider using them when you need to group related functionality that doesn’t need to be exposed globally. They are also useful when you want to maintain state across function calls without relying on global variables. For instance, if you’re building a complex application with multiple components, nested functions can help you manage scope and state effectively.
Here’s another practical example:
function multiplier(factor) {
return function(number) {
return number * factor;
};
}
const double = multiplier(2);
console.log(double(5));
Output:
10
In this case, the multiplier
function takes a factor
and returns a new function that multiplies its input by that factor. This pattern is particularly useful in scenarios where you want to create specific functions based on a general one, such as creating different multipliers.
Best Practices for Nested Functions
While nested functions are powerful, there are best practices to keep in mind. First, avoid excessive nesting, as it can make your code harder to read. Aim to keep functions concise and focused on a single task. Additionally, ensure that your inner functions are only used where necessary. If a function can stand alone, consider defining it at a higher scope. Lastly, always be mindful of memory usage, especially in environments with limited resources, as closures can lead to increased memory consumption.
Here’s a refined example that adheres to these best practices:
function fetchData(apiUrl) {
return function(callback) {
fetch(apiUrl)
.then(response => response.json())
.then(data => callback(data))
.catch(error => console.error('Error:', error));
};
}
const getUserData = fetchData('https://api.example.com/user');
getUserData(data => console.log(data));
Output:
{ id: 1, name: "John Doe" }
In this refined example, fetchData
is a higher-order function that returns a function responsible for fetching data from a given API URL. This keeps the code modular and reusable, while still demonstrating the power of nested functions effectively.
Conclusion
Nested functions in JavaScript are a powerful tool that can help you manage complexity, encapsulate data, and improve code organization. By understanding how to use them effectively, you can write cleaner, more efficient code that is easier to maintain. As you continue your journey in JavaScript, remember to consider the scope and structure of your functions. Embracing nested functions can elevate your coding skills and lead to better coding practices in your projects.
FAQ
-
What are nested functions in JavaScript?
Nested functions are functions defined within another function, allowing access to the outer function’s variables. -
Why should I use nested functions?
They help organize code logically, maintain data privacy, and improve performance by reducing global scope usage. -
Can nested functions access outer function variables?
Yes, inner functions can access variables and parameters of their outer functions due to closures. -
Are there any downsides to using nested functions?
Excessive nesting can lead to code that is difficult to read and maintain, so it’s essential to use them judiciously.
- How can I improve my use of nested functions?
Focus on keeping them concise, avoid unnecessary nesting, and ensure inner functions are only used where necessary.