Difference Between i++ and ++i in JavaScript
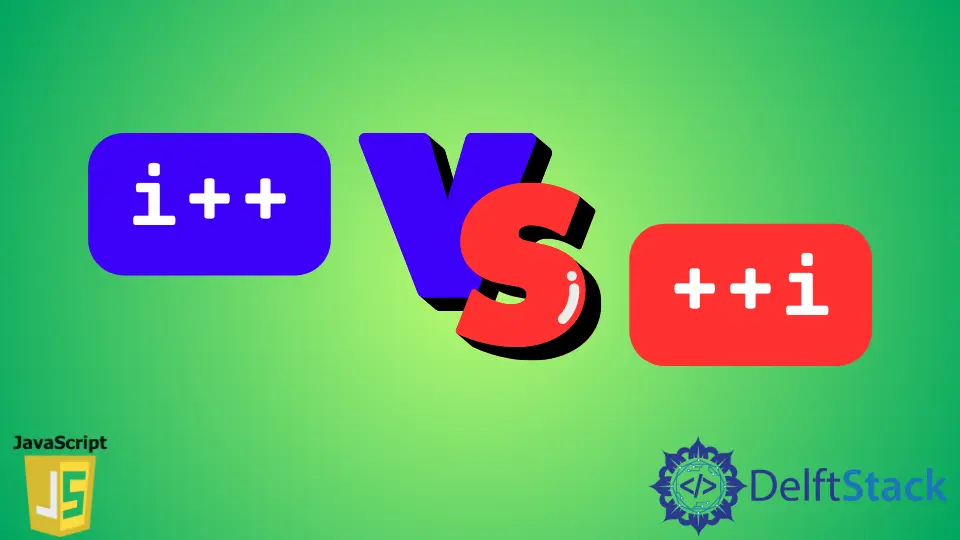
This tutorial article explains the increment and decrement operators in detail.
Increment and Decrement Operators in JavaScript
The increment and decrement operators are utilized to increase or decrease the value of a variable by 1. These increment and decrement operators can be written in multiple ways, and each way has its properties, meaning they are not the same. We usually see these operators in loops and are much familiar with their use in loops.
The following are the two most common ways of using increment and decrement operators:
++variable
(Prefix)variable++
(Postfix)--variable
(Prefix)variable--
(Postfix)
These two methods of increment/decrement operators are the same in that they update the value of the variable by 1. However, it has its differences if looked closely.
In the following example, we will use the Prefix method of using the increment operator and understand its working.
var i = 5;
alert(++i);
alert(i);
In the code mentioned above, the initial value of the variable i
is 5, and in the next line, we used an alert message to show the working of prefix increment. Now the alert will get the value of i
after the increment. So the alert will generate the value of i
as 6. And the next line, which has an alert for i
, will return the same value as the previous alert, 6.
Let’s have a look at the postfix method of using increment operators.
var i = 5;
alert(i++);
alert(i);
In this code segment, we have a postfix increment operator, which is used in an alert. The i
variable’s initial value is 5, and the increment operator will make it 6.
However, if you use the postfix increment operator in an alert, you will notice that the alert will have the value of i
as before, which is 5; no increment can be seen here.
But in the next line, where the alert is used to get the value of i
, it will have a new value incremented by 1. So the new value will be 6.
Here, the prefix method increments the variable by 1 first and then is fetched by the alert. However, in the postfix, the i
variable’s value is fetched before the increment, so the first alert shows the same value as before.
And once the first alert is executed, the value of the i
variable is updated and is fetched by the next alert message.
Another way of writing the increment operator is
> i = i + 1;
This method is the same as the prefix method and is used if we want to change the number of increments. Let’s have a look at the following example.
var i = 5;
i = i + 1
alert(i);
In this example, the variable i
is incremented by 1. So at the time where the alert tries to fetch the value of the variable i
, the variable will send the new incremented value, and the first alert will have an output of value 6. This method is the same as the prefix method, and before the value is fetched, the increment operation takes place.
Postfix vs Prefix Increment Operator in JavaScript
The following is a complex example using different ways of using the increment operators:
var i = 5;
var j = 10;
alert(i++); // shows 5
alert(j++); // shows 10
alert(++i); // shows 7
alert(++j); // shows 12
alert(i = i + 1); // shows 8
alert(i); // shows 8
We have used multiple increment operators in this code segment, and each line has its output written as the comment. The postfix increment is used in the first and second alerts, so the variable’s value is incremented after the alert has fetched it.
Similarly, the alerts show the value after incrementing in the third and fourth alert messages as the prefix increment operator.
In some languages, the postfix and prefix have no difference, but in JavasScript, they serve the same purpose if you are not concerned with the immediate result. However, if you have a JavaScript program that needs the immediate results, then the difference between the postfix and prefix is that :
Postfix (i++
): returns the value of variable i
first and then increments the variable
Prefix(i--
): return the value of variable i
after the increment is done to the variable.