The bind() Method in JavaScript
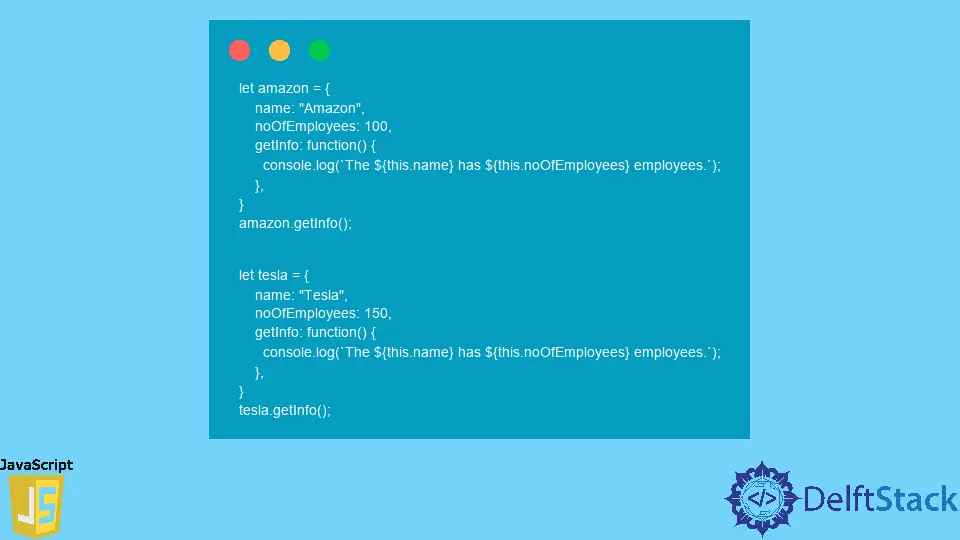
Whenever we call a function in JavaScript the reference of the function will always point to the global object i.e, the window object. This is fine for normal functions, but when it comes to nested functions or the functions present inside an object, this creates a problem. Because here, to call the inner functions, you have to use the object name or the outer function name to call the inner function. So, if you want to use a function that is present inside an object or present inside some other function, you can’t directly do it since it will be pointing to a global context.
To solve this, you can bind the inner function and pass the new context inside the bind function or declare that function outside and then bind the function and pass the new context. The bind()
is a function in JavaScript that will let you change the global context with some other context where the function resides. This is also known as function borrowing. Let’s understand the bind method is used in practice with an example.
Using bind()
Method in JavaScript
In the below code, we have two objects, amazon
and tesla
. Each of these objects contains some properties such as name
, noOfEmployees
, and a function getInfo()
. Here, we call the getInfo()
function, which is present inside the amazon
object with the help of the amazon
object name, and inside the tesla
object with the help of the tesla
object name.
let amazon = {
name: 'Amazon',
noOfEmployees: 100,
getInfo: function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
},
} amazon.getInfo();
let tesla = {
name: 'Tesla',
noOfEmployees: 150,
getInfo: function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
},
} tesla.getInfo();
Output:
The Amazon has 100 employees.
The Tesla has 150 employees.
If you run the above program, you will get the above output. The problem with this approach is that the function getInfo()
does the same thing as printing a line to the console. Also, we have declared the same function in both the objects that leads to code repetition.
Instead, what we can do is we can declare the getInfo()
function outside the object and remove it from the objects as shown below.
let amazon = {
name: 'Amazon',
noOfEmployees: 100,
}
let tesla = {
name: 'Tesla',
noOfEmployees: 150,
}
let getInfo =
function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
}
let amazon_Data = getInfo.bind(amazon);
let tesla_Data = getInfo.bind(tesla);
amazon_Data();
tesla_Data();
Output:
The Amazon has 100 employees.
The Tesla has 150 employees.
Then bind the getInfo()
function with the two objects amazon
and tesla
using bind()
function. And then store the reference inside another various amazon_Data
and tesla_Data
.
Now to call the functions you have stored inside amazon_Data
and tesla_Data
, you have to put a ()
round brackets. This will give you the same output as we got previously. The only difference here is that the approach which we followed.
You can also directly call the method without storing the reference by adding one more ()
at the end of the bind method, as shown below.
getInfo.bind(amazon)();
getInfo.bind(tesla)();
This concept is also known as function borrowing because the same function getInfo()
is being used or borrowed by two different objects, amazon
and tesla
.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn