JavaScript 中的 bind() 方法
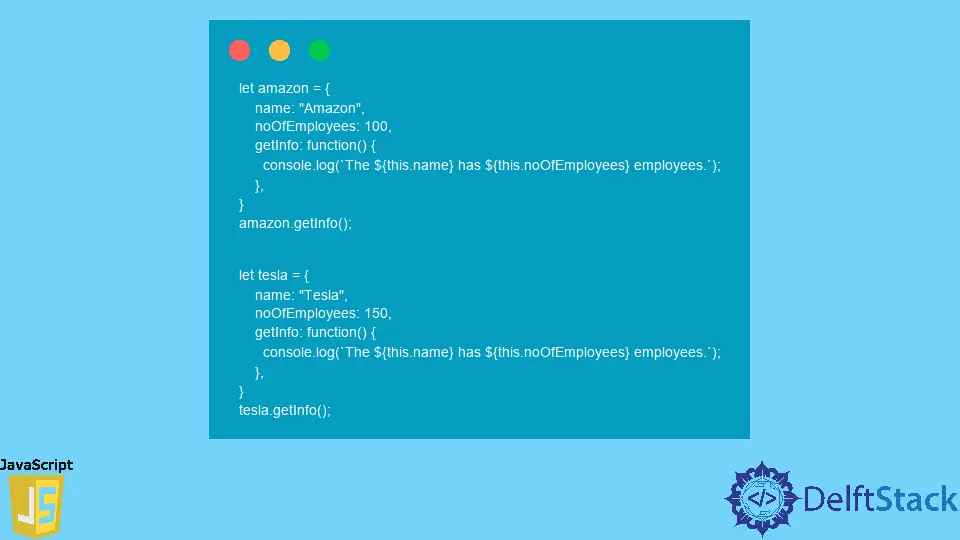
每当我们在 JavaScript 中调用一个函数时,该函数的引用将始终指向全局对象,即 window 对象。这对于普通函数来说很好,但是当涉及嵌套函数或对象中存在的函数时,这会产生问题。因为在这里,要调用内部函数,必须使用对象名称或外部函数名称来调用内部函数。因此,如果你想使用存在于对象中或存在于其他函数中的函数,则不能直接使用,因为它将指向全局上下文。
为了解决这个问题,你可以绑定内部函数并在绑定函数内部传递新的上下文,或者在外部声明该函数然后绑定函数并传递新的上下文。bind()
是 JavaScript 中的一个函数,它可以让你使用函数所在的其他上下文更改全局上下文。这也称为函数借用。让我们通过一个例子来了解 bind 方法在实践中的使用。
在 JavaScript 中使用 bind()
方法
在下面的代码中,我们有两个对象,amazon
和 tesla
。这些对象中的每一个都包含一些属性,例如 name
、noOfEmployees
和一个函数 getInfo()
。在这里,我们调用 getInfo()
函数,该函数在 amazon
对象名称的帮助下出现在 amazon
对象内部,在 tesla
对象名称的帮助下出现在 tesla
对象内部。
let amazon = {
name: 'Amazon',
noOfEmployees: 100,
getInfo: function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
},
} amazon.getInfo();
let tesla = {
name: 'Tesla',
noOfEmployees: 150,
getInfo: function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
},
} tesla.getInfo();
输出:
The Amazon has 100 employees.
The Tesla has 150 employees.
如果你运行上面的程序,你会得到上面的输出。这种方法的问题在于函数 getInfo()
的作用与将一行打印到控制台的作用相同。此外,我们在导致代码重复的两个对象中声明了相同的函数。
相反,我们可以做的是我们可以在对象外声明 getInfo()
函数,并将其从对象中删除,如下所示。
let amazon = {
name: 'Amazon',
noOfEmployees: 100,
}
let tesla = {
name: 'Tesla',
noOfEmployees: 150,
}
let getInfo =
function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
}
let amazon_Data = getInfo.bind(amazon);
let tesla_Data = getInfo.bind(tesla);
amazon_Data();
tesla_Data();
输出:
The Amazon has 100 employees.
The Tesla has 150 employees.
然后使用 bind()
函数将 getInfo()
函数与两个对象 amazon
和 tesla
绑定。然后将引用存储在另一个不同的 amazon_Data
和 tesla_Data
中。
现在要调用你存储在 amazon_Data
和 tesla_Data
中的函数,你必须放置一个 ()
圆括号。这将为你提供与我们之前得到的相同的输出。这里唯一的区别是我们遵循的方法。
也可以通过在 bind 方法的末尾多加一个 ()
直接调用该方法而不存储引用,如下所示。
getInfo.bind(amazon)();
getInfo.bind(tesla)();
这个概念也被称为函数借用,因为相同的函数 getInfo()
正在被两个不同的对象使用或借用,amazon
和 tesla
。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn