JavaScript 中的 bind() 方法
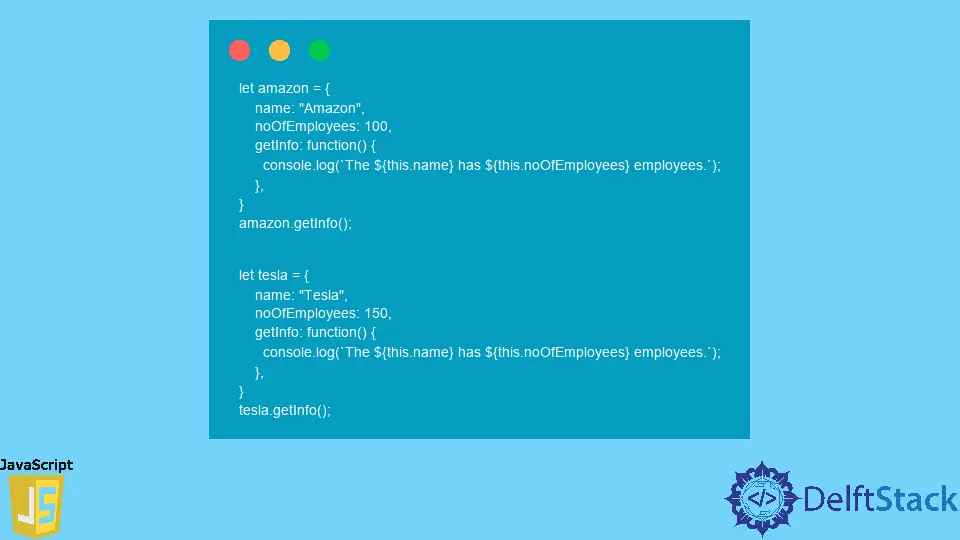
每當我們在 JavaScript 中呼叫一個函式時,該函式的引用將始終指向全域性物件,即 window 物件。這對於普通函式來說很好,但是當涉及巢狀函式或物件中存在的函式時,這會產生問題。因為在這裡,要呼叫內部函式,必須使用物件名稱或外部函式名稱來呼叫內部函式。因此,如果你想使用存在於物件中或存在於其他函式中的函式,則不能直接使用,因為它將指向全域性上下文。
為了解決這個問題,你可以繫結內部函式並在繫結函式內部傳遞新的上下文,或者在外部宣告該函式然後繫結函式並傳遞新的上下文。bind()
是 JavaScript 中的一個函式,它可以讓你使用函式所在的其他上下文更改全域性上下文。這也稱為函式借用。讓我們通過一個例子來了解 bind 方法在實踐中的使用。
在 JavaScript 中使用 bind()
方法
在下面的程式碼中,我們有兩個物件,amazon
和 tesla
。這些物件中的每一個都包含一些屬性,例如 name
、noOfEmployees
和一個函式 getInfo()
。在這裡,我們呼叫 getInfo()
函式,該函式在 amazon
物件名稱的幫助下出現在 amazon
物件內部,在 tesla
物件名稱的幫助下出現在 tesla
物件內部。
let amazon = {
name: 'Amazon',
noOfEmployees: 100,
getInfo: function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
},
} amazon.getInfo();
let tesla = {
name: 'Tesla',
noOfEmployees: 150,
getInfo: function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
},
} tesla.getInfo();
輸出:
The Amazon has 100 employees.
The Tesla has 150 employees.
如果你執行上面的程式,你會得到上面的輸出。這種方法的問題在於函式 getInfo()
的作用與將一行列印到控制檯的作用相同。此外,我們在導致程式碼重複的兩個物件中宣告瞭相同的函式。
相反,我們可以做的是我們可以在物件外宣告 getInfo()
函式,並將其從物件中刪除,如下所示。
let amazon = {
name: 'Amazon',
noOfEmployees: 100,
}
let tesla = {
name: 'Tesla',
noOfEmployees: 150,
}
let getInfo =
function() {
console.log(`The ${this.name} has ${this.noOfEmployees} employees.`);
}
let amazon_Data = getInfo.bind(amazon);
let tesla_Data = getInfo.bind(tesla);
amazon_Data();
tesla_Data();
輸出:
The Amazon has 100 employees.
The Tesla has 150 employees.
然後使用 bind()
函式將 getInfo()
函式與兩個物件 amazon
和 tesla
繫結。然後將引用儲存在另一個不同的 amazon_Data
和 tesla_Data
中。
現在要呼叫你儲存在 amazon_Data
和 tesla_Data
中的函式,你必須放置一個 ()
圓括號。這將為你提供與我們之前得到的相同的輸出。這裡唯一的區別是我們遵循的方法。
也可以通過在 bind 方法的末尾多加一個 ()
直接呼叫該方法而不儲存引用,如下所示。
getInfo.bind(amazon)();
getInfo.bind(tesla)();
這個概念也被稱為函式借用,因為相同的函式 getInfo()
正在被兩個不同的物件使用或借用,amazon
和 tesla
。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn