How to Fix the Warning: Uses or Overrides a Deprecated API in Java
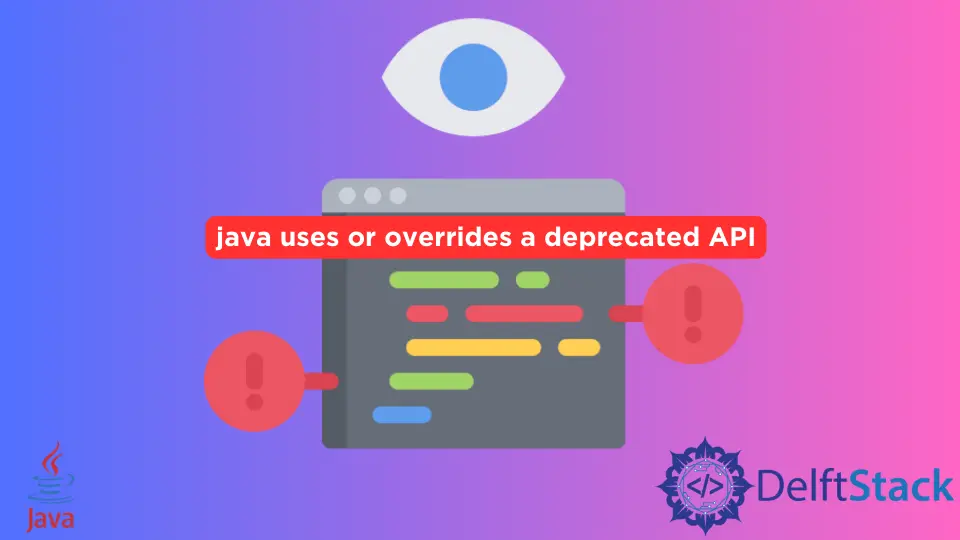
Today, we will see why a warning says uses or overrides a deprecated API
and demonstrate how to fix this to accomplish the task.
Fix Warning Saying uses or overrides a deprecated API
in Java
Example Code (that contains a warning):
// import libraries
import java.io.BufferedInputStream;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
// Main class
public class Main {
// main method
public static void main(String[] args) {
// path of a text file
File filePath = new File("Files/TestFile.txt");
try {
// obtain input bytes from a file
FileInputStream fileInputStream = new FileInputStream(filePath);
// adds the functionality to another input stream
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream);
// lets an app read primitive Java data types from the specified input stream
DataInputStream dataInputStream = new DataInputStream(bufferedInputStream);
if (dataInputStream.available() != 0) {
// Get a line.
String line = dataInputStream.readLine();
// Place words to an array which are split by a "space".
String[] stringParts = line.split(" ");
// Initialize the word's maximum length.
int maximumLength = 1;
// iterate over each stingPart, the next one is addressed as "stringX"
for (String stringX : stringParts) {
// If a document contains the word longer than.
if (maximumLength < stringX.length())
// Set the new value for the maximum length.
maximumLength = stringX.length();
} // end for-loop
// +1 because array index starts from "0".
int[] counter = new int[maximumLength + 1];
for (String str : stringParts) {
// Add one to the number of words that length has
counter[str.length()]++;
}
// We are using this kind of loop because we require the "length".
for (int i = 1; i < counter.length; i++) {
System.out.println(i + " letter words: " + counter[i]);
} // end for-loop
} // end if statement
} // end try
catch (IOException ex) {
ex.printStackTrace();
} // end catch
} // end main method
} // end Main class
In this code, we access a .txt
file, read that file line by line and place the words in an array which are split based on a single space
. Then, we count the number of characters in each word and display all of them in the program output.
Though this program generates the output, it also highlights that we are using or overriding a deprecated API at line String line = dataInputStream.readLine();
. See the following.
This warning is being generated using the readLine()
method of the DataInputStream
class. According to the documentation, this method has been deprecated since JDK 1.1
because it does not convert bytes to characters properly.
Although, the method is deprecated and likely will perform as expected in some cases. But we cannot guarantee that it will fulfill its job anymore.
Therefore, it is good to use a similar but consistent method.
As of JDK 1.1
, the method preferred for reading the lines of text is the readLine()
function from the BufferedReader
class. We don’t have to change all the code from scratch but only need to convert the DataInputStream
to the BufferedReader
class.
Replace this line of code:
DataInputStream dataInputStream = new DataInputStream(in);
With this line of code:
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(in));
Now, the complete working program will look as follows.
// import libraries
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
// Main class
public class Main {
// main method
public static void main(String[] args) {
// path of a text file
File filePath = new File("Files/TestFile.txt");
try {
// obtain input bytes from a file
FileInputStream fileInputStream = new FileInputStream(filePath);
// adds the functionality to another input stream
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream);
// lets an app read primitive Java data types from the specified input stream
// DataInputStream dataInputStream = new DataInputStream(bufferedInputStream);
BufferedReader bufferedReader =
new BufferedReader(new InputStreamReader(bufferedInputStream));
String line = "";
// get a line and check if it is not null
if ((line = bufferedReader.readLine()) != null) {
// Place words to an array which are split by a "space".
String[] stringParts = line.split(" ");
// Initialize the word's maximum length.
int maximumLength = 1;
// iterate over each stingPart, the next one is addressed as "stringX"
for (String stringX : stringParts) {
// If a document contains the word longer than.
if (maximumLength < stringX.length())
// Set the new value for the maximum length.
maximumLength = stringX.length();
} // end for-loop
// +1 because array index starts from "0".
int[] counter = new int[maximumLength + 1];
for (String str : stringParts) {
// Add one to the number of words that length has
counter[str.length()]++;
}
// We are using this kind of loop because we require the "length".
for (int i = 1; i < counter.length; i++) {
System.out.println(i + " letter words: " + counter[i]);
} // end for-loop
} // end if statement
} // end try
catch (IOException ex) {
ex.printStackTrace();
} // end catch
} // end main method
} // end Main class
Additionally, if you also see something similar to the following.
Recompile with -Xlint: deprecation for details
Don’t worry; it just tells you an option to use while compiling to have more details about where you are using the deprecated stuff.