경고 수정: Java에서 더 이상 사용되지 않는 API 사용 또는 재정의
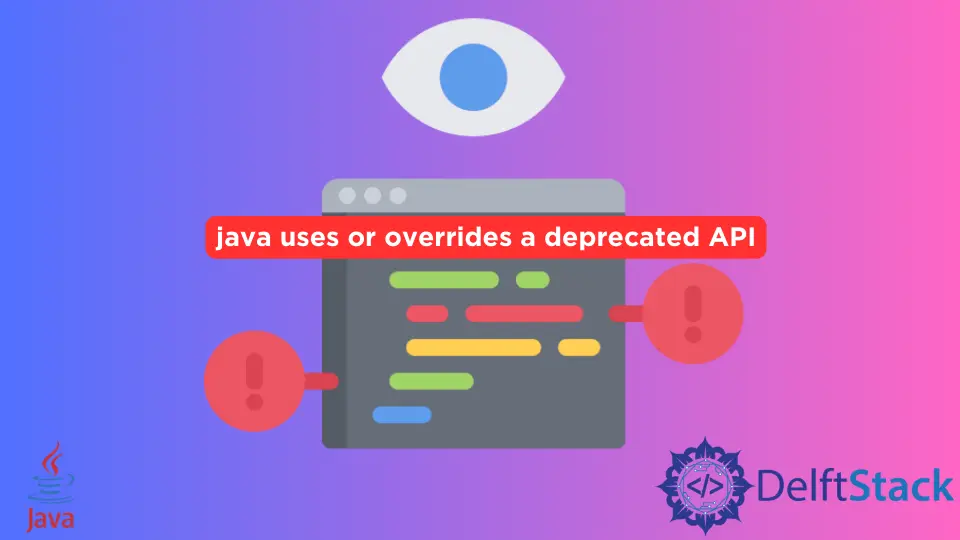
오늘, 우리는 경고가 더 이상 사용되지 않는 API를 사용하거나 재정의합니다
라고 말하는 이유를 알아보고 작업을 수행하기 위해 이를 수정하는 방법을 보여줍니다.
Java에서 더 이상 사용되지 않는 API를 사용하거나 재정의합니다
라는 경고 메시지 수정
예제 코드(경고 포함):
// import libraries
import java.io.BufferedInputStream;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
// Main class
public class Main {
// main method
public static void main(String[] args) {
// path of a text file
File filePath = new File("Files/TestFile.txt");
try {
// obtain input bytes from a file
FileInputStream fileInputStream = new FileInputStream(filePath);
// adds the functionality to another input stream
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream);
// lets an app read primitive Java data types from the specified input stream
DataInputStream dataInputStream = new DataInputStream(bufferedInputStream);
if (dataInputStream.available() != 0) {
// Get a line.
String line = dataInputStream.readLine();
// Place words to an array which are split by a "space".
String[] stringParts = line.split(" ");
// Initialize the word's maximum length.
int maximumLength = 1;
// iterate over each stingPart, the next one is addressed as "stringX"
for (String stringX : stringParts) {
// If a document contains the word longer than.
if (maximumLength < stringX.length())
// Set the new value for the maximum length.
maximumLength = stringX.length();
} // end for-loop
// +1 because array index starts from "0".
int[] counter = new int[maximumLength + 1];
for (String str : stringParts) {
// Add one to the number of words that length has
counter[str.length()]++;
}
// We are using this kind of loop because we require the "length".
for (int i = 1; i < counter.length; i++) {
System.out.println(i + " letter words: " + counter[i]);
} // end for-loop
} // end if statement
} // end try
catch (IOException ex) {
ex.printStackTrace();
} // end catch
} // end main method
} // end Main class
이 코드에서는 .txt
파일에 액세스하고 해당 파일을 한 줄씩 읽고 단일 공백
을 기준으로 분할된 단어를 배열에 배치합니다. 그런 다음 각 단어의 문자 수를 세고 프로그램 출력에 모두 표시합니다.
이 프로그램은 출력을 생성하지만 String line = dataInputStream.readLine();
줄에서 더 이상 사용되지 않는 API를 사용하거나 재정의하고 있음을 강조합니다. 다음을 참조하십시오.
이 경고는 DataInputStream
클래스의 readLine()
메소드를 사용하여 생성됩니다. 문서에 따르면 이 메서드는 바이트를 문자로 제대로 변환하지 않기 때문에 JDK 1.1
부터 사용되지 않습니다.
그러나 이 메서드는 더 이상 사용되지 않으며 경우에 따라 예상대로 수행될 수 있습니다. 그러나 우리는 그것이 더 이상 그 역할을 수행할 것이라고 보장할 수 없습니다.
따라서 유사하지만 일관된 방법을 사용하는 것이 좋습니다.
JDK 1.1
부터 텍스트 줄을 읽는 데 선호되는 방법은 BufferedReader
클래스의 readLine()
함수입니다. 처음부터 모든 코드를 변경할 필요는 없지만 DataInputStream
을 BufferedReader
클래스로 변환하기만 하면 됩니다.
다음 코드 줄을 바꿉니다.
DataInputStream dataInputStream = new DataInputStream(in);
이 코드 줄을 사용하여:
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(in));
이제 완전한 작업 프로그램은 다음과 같습니다.
// import libraries
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
// Main class
public class Main {
// main method
public static void main(String[] args) {
// path of a text file
File filePath = new File("Files/TestFile.txt");
try {
// obtain input bytes from a file
FileInputStream fileInputStream = new FileInputStream(filePath);
// adds the functionality to another input stream
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream);
// lets an app read primitive Java data types from the specified input stream
// DataInputStream dataInputStream = new DataInputStream(bufferedInputStream);
BufferedReader bufferedReader =
new BufferedReader(new InputStreamReader(bufferedInputStream));
String line = "";
// get a line and check if it is not null
if ((line = bufferedReader.readLine()) != null) {
// Place words to an array which are split by a "space".
String[] stringParts = line.split(" ");
// Initialize the word's maximum length.
int maximumLength = 1;
// iterate over each stingPart, the next one is addressed as "stringX"
for (String stringX : stringParts) {
// If a document contains the word longer than.
if (maximumLength < stringX.length())
// Set the new value for the maximum length.
maximumLength = stringX.length();
} // end for-loop
// +1 because array index starts from "0".
int[] counter = new int[maximumLength + 1];
for (String str : stringParts) {
// Add one to the number of words that length has
counter[str.length()]++;
}
// We are using this kind of loop because we require the "length".
for (int i = 1; i < counter.length; i++) {
System.out.println(i + " letter words: " + counter[i]);
} // end for-loop
} // end if statement
} // end try
catch (IOException ex) {
ex.printStackTrace();
} // end catch
} // end main method
} // end Main class
또한 다음과 유사한 내용도 표시되는 경우.
Recompile with -Xlint: deprecation for details
괜찮아요; 더 이상 사용되지 않는 항목을 사용하는 위치에 대한 자세한 정보를 얻기 위해 컴파일하는 동안 사용할 옵션을 알려줍니다.