How to Fix Unreported Exception Must Be Caught or Declared to Be Thrown
-
Java Error
unreported exception must be caught or declared to be thrown
- Handle Exception in the Calling Function in Java
- Make the Calling Function Throw the Same Exception in Java
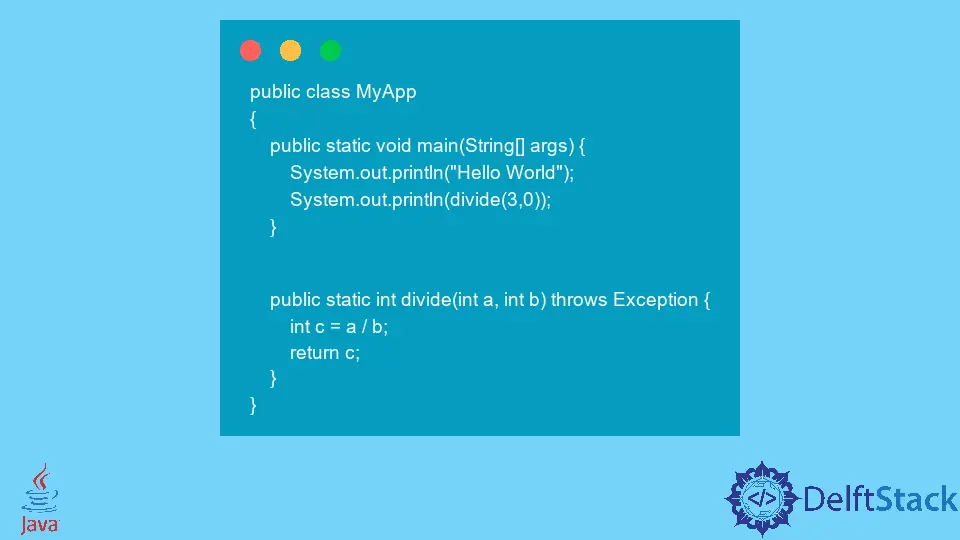
Java is an object-oriented, strongly typed and compiled language and has the concept of classes to leverage different aspects of programming like inheritance and polymorphism. It is also good support for handling exceptions due to highly nested functions.
This article will demonstrate the unreported exception must be caught or declared to be thrown
compile time error in Java.
Java Error unreported exception must be caught or declared to be thrown
Consider the following code sample.
public class MyApp {
public static void main(String[] args) {
System.out.println("Hello World");
System.out.println(divide(3, 0));
}
public static int divide(int a, int b) throws Exception {
int c = a / b;
return c;
}
}
The following error is obtained when the above code sample is compiled using javac MyApp.java
.
MyApp.java:7: error: unreported exception Exception; must be caught or declared to be thrown
System.out.println(divide(3,0));
^
1 error
So what is happening here?
The function divide(int a, int b)
throws an Exception
which is not caught or handled anywhere in the calling function, which in this case is main(String[] args)
. It can be resolved by handling the exception or making the calling function throw the same exception.
Handle Exception in the Calling Function in Java
So in the last section, this was one of the approaches to resolve the error. Let’s understand it with an example considering the code sample discussed above.
public class MyApp {
public static void main(String[] args) {
System.out.println("Hello World");
try {
System.out.println(divide(3, 0));
} catch (Exception e) {
System.out.println("Division by zero !!!");
}
}
public static int divide(int a, int b) throws Exception {
int c = a / b;
return c;
}
}
Now when we try to compile the code, it gets compiled successfully, and the following output is obtained on running the code.
Hello World
Division by zero !!!
Thus, the exception is now handled in the main
method, and the print
statement in the catch
block is executed.
Make the Calling Function Throw the Same Exception in Java
So the other approach is to make the calling function also throw the same exception. Let’s take an example of how that can be achieved.
public class MyApp {
public static void main(String[] args) throws Exception {
System.out.println("Hello World");
try {
performOperation();
} catch (Exception e) {
System.out.println("Division by zero!!");
}
}
public static void performOperation() throws Exception {
divide(3, 0);
}
public static int divide(int a, int b) throws Exception {
int c = a / b;
return c;
}
}
So we have taken the previous example and added an extra function, performOperation()
, in between. We have made the performOperation()
function also throw the same Exception
as divide(int a, int b)
; thus, it gets compiled successfully, and the same output can be obtained.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack