How to Return an ArrayList in Java
- Return an ArrayList From a Non-Static Function in Java
- Return an ArrayList From a Static Function in Java
- Conclusion
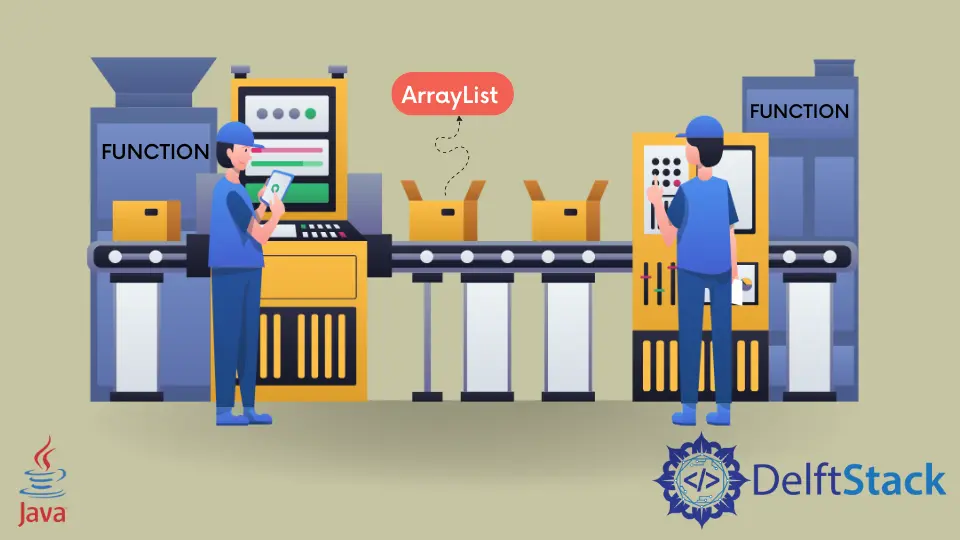
An ArrayList, part of the java.util
package, is a dynamic and resizable data structure in Java. Unlike traditional arrays, ArrayLists can be easily adjusted in size, making them highly flexible; however, due to their dynamic nature, they can occasionally exhibit slower performance.
In this tutorial, our focus is on returning an ArrayList from a function within a Java class.
Return an ArrayList From a Non-Static Function in Java
In Java, non-static functions are associated with instances (objects) of a class. These functions can access instance-specific data and provide a means to encapsulate behavior within a class.
To invoke a non-static function, we need an object of the class that contains the function.
In the following code, we will work with a non-static function that creates and returns an ArrayList of some size and try to invoke this function in another class. Since this function is non-static, we will need an object of the class to invoke it.
import java.util.ArrayList;
import java.util.List; // You need to import List from java.util
public class ClassA {
public static void main(String args[]) {
ClassB m1 = new ClassB();
List<Integer> listInClassA = m1.myNumbers(); // Use List<Integer> instead of raw List
System.out.println("The List is " + listInClassA);
}
}
public class ClassB {
public ArrayList<Integer> myNumbers() {
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(10);
numbers.add(15);
numbers.add(30);
return numbers;
}
}
Output:
The List is [10, 15, 30]
As we can see, the function myNumbers()
is not static, so we need to create an instance of ClassB
in ClassA
, which we named m1
. This allows us to have access to the ArrayList method myNumbers()
of ClassB
.
After that, we called the myNumbers()
method on the m1
object of ClassB
. Here’s what happened step by step:
-
We defined the method with a return type of
ArrayList<Integer>
, indicating that it will return an ArrayList containing integers. -
Inside the method, we created a new ArrayList called
numbers
that will hold integer values. -
We added three integer values (10, 15, and 30) to the
numbers
ArrayList using theadd()
method. -
Finally, we returned the populated
numbers
ArrayList.
Return an ArrayList From a Static Function in Java
A static function can be accessed or invoked without creating an object of the class to which it belongs. If the static method is to be called from outside its parent class, we have to specify the class where that static function was defined.
Let’s modify our code slightly while working with a static function.
import java.util.ArrayList;
import java.util.List;
public class ClassA {
public static void main(String args[]) {
List<Integer> listInClassA =
ClassB.myNumbers(); // Use ClassB.myNumbers() to call the static method
System.out.println("The List is " + listInClassA);
}
}
public class ClassB {
public static ArrayList<Integer> myNumbers() {
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(10);
numbers.add(15);
numbers.add(30);
return numbers;
}
}
Output:
The List is [10, 15, 30]
In the above example, we referred to the function from classB
in classA
without creating an object of classB
.
As we can see, returning an ArrayList from a static method in Java is a straightforward process. We can access static methods using the class name followed by the method name and then use them to perform various operations, including creating and returning collections like ArrayList.
Conclusion
In Java, it is common to encapsulate functionality within classes and return results from functions or methods. In a nutshell, returning an ArrayList from a function involves the following steps:
-
Define the ArrayList: Within the function, create an ArrayList and populate it with the desired elements.
-
Specify the Return Type: Declare the return type of the function to be
ArrayList<Type>
, whereType
represents the type of elements the ArrayList will hold. -
Return the ArrayList: Use the
return
keyword to send the populated ArrayList back to the calling code.