Or Statement in Java: Your Guide to the Logical OR Operator
-
What Is
OR
Statement in Java -
Purpose of the
OR
Statement in Java -
Types of
OR
Statements in Java -
OR
Statement in Java Rules -
OR
Statement in Java Troubleshooting -
How to Avoid Java
OR
Statement Errors -
OR
Statement in Java Conclusion
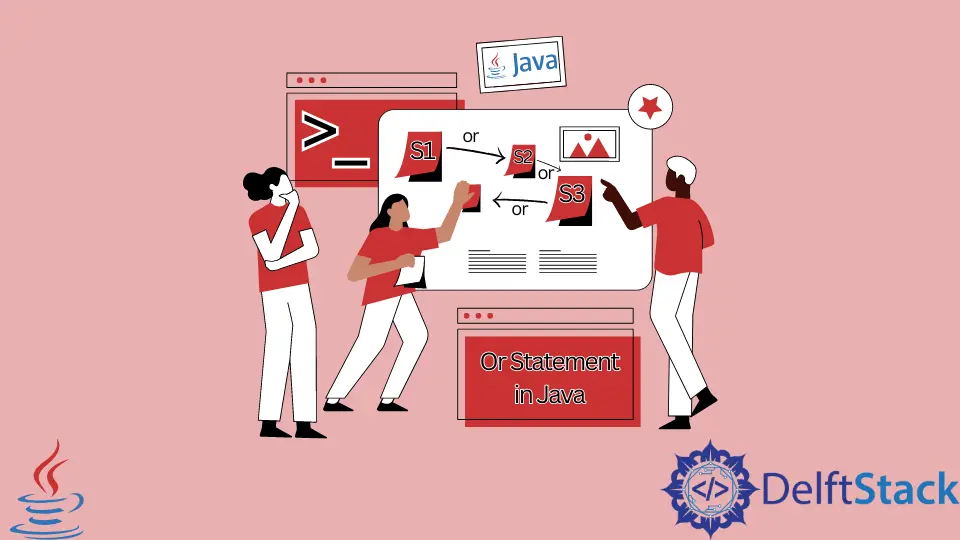
In programming, the OR
statement is a fundamental concept used to control the flow of a program by evaluating conditions and making decisions based on the results. In Java, a popular and versatile programming language, the OR
statement is implemented as a logical operator, specifically the ||
operator.
In this article, we will explore the Java OR
statement, how it works, and its practical applications.
What Is OR
Statement in Java
The OR
operator, ||
, is used to evaluate multiple Boolean expressions and return true
if at least one of them is true. If all expressions are false, it returns false
.
Here is the truth table for the OR
statement:
A | B | A OR B |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
The OR
operator is a short-circuit logical operator, which means that it evaluates the expressions from left to right and stops as soon as it finds a true value. If the left expression is true, it doesn’t evaluate the right expression, as the overall result is already determined.
Here’s the syntax of the OR
operator in Java:
expression1 || expression2
The OR
operator is commonly used in conditional statements, loops, and decision-making processes to create more dynamic and flexible code.
Purpose of the OR
Statement in Java
The purpose of the OR
statement is to evaluate to true
if at least one of the expressions it connects is true
. If both expressions are false
, then the entire OR
statement evaluates to false
.
In Java, the OR
statement is commonly used in conditional expressions, such as in if
statements or loops, to make decisions based on multiple conditions.
It allows developers to create flexible and dynamic control flow in their programs by specifying that a particular block of code should be executed if at least one of the specified conditions holds true.
Types of OR
Statements in Java
Using the OR
Statement in Conditional Statements in Java
Conditional statements in Java allow you to execute different blocks of code depending on whether certain conditions are met. The OR
operator (||
) plays a significant role in this process, as it allows you to evaluate multiple conditions and make decisions based on the results.
Code Example Using the OR
Statement in Conditional Statements:
import java.util.Scanner;
public class AuthenticationExample {
public static void main(String[] args) {
// Assume predefined username and password
String correctUsername = "admin";
String correctPassword = "secure123";
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your username: ");
String username = scanner.nextLine();
System.out.print("Enter your password: ");
String password = scanner.nextLine();
// Conditional statement using OR statement for authentication
if (username.equals(correctUsername) || password.equals(correctPassword)) {
System.out.println("Authentication successful. Welcome, " + username + "!");
} else {
System.out.println("Authentication failed. Invalid username or password.");
}
scanner.close();
}
}
Output:
In this example, the user is prompted to enter a username and a password.
The OR
statement in the conditional statement checks if either the entered username matches the correct username (username.equals(correctUsername)
) or if the entered password matches the correct password (password.equals(correctPassword)
).
If either condition is true, it prints Authentication successful. Welcome, [username]!
. Otherwise, it prints Authentication failed. Invalid username or password.
.
This simulates a basic form of authentication where either the correct username or password is sufficient for access. In a real-world scenario, secure authentication mechanisms should be used.
Using the OR
Statement as Loop Control in Java
The OR
operator, denoted as ||
, is not just a tool for conditional statements; it can also be used for controlling loops in Java.
This time, we will see how the OR
operator can be effectively employed to manage loops and regulate their execution based on multiple conditions.
Code Example Using the OR
Statement as Loop Control in Java:
public class OrOperatorLoopControl {
public static void main(String[] args) {
boolean condition1 = false;
boolean condition2 = true;
int iteration = 0;
while (!condition1 || !condition2) {
iteration++;
System.out.println(
"Iteration " + iteration + ": Loop continues until at least one condition is true");
if (iteration == 3) {
condition1 = true; // Modify condition1 after 3 iterations
}
if (iteration == 5) {
condition2 = false; // Modify condition2 after 5 iterations
}
}
System.out.println("Loop exited after " + iteration + " iterations.");
}
}
Output:
In this example, we have the following key elements: the Boolean variables (condition
and condition2
) and the iteration
variable.
The Boolean variables, condition1
and condition2
, are initially set to false
and true
, respectively. The iteration
variable is used to keep track of the loop’s iterations.
The while
loop is the central component controlling the program’s flow. It evaluates the combined conditions using the OR
operator (||
).
The loop continues until either condition1
or condition2
becomes true. In this case, condition1
becomes true after three iterations, causing the loop to exit.
The final output indicates that the loop exited after three iterations.
Using the OR
Statement for Input Validation in Java
In Java programming, the OR
operator (||
) serves a crucial role in input validation, allowing developers to ensure that at least one condition is met when verifying user-provided data.
Now, we’ll explore how the OR
operator can be used for input validation, providing a detailed explanation of the code example provided and the expected output.
Code Example Using the OR
Statement for Input Validation:
import java.util.Scanner;
public class InputValidationExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your age: ");
int age = scanner.nextInt();
System.out.print("Do you have a driver's license? (yes/no): ");
String hasLicense = scanner.next().toLowerCase();
// Input validation using OR statement
if (age >= 18 || hasLicense.equals("yes")) {
System.out.println("You are eligible to drive. Drive safely!");
} else {
System.out.println("You are not eligible to drive yet.");
}
scanner.close();
}
}
Output:
In this example, the Java code prompts the user to enter their age. It then asks whether the user has a driver’s license (accepting yes
or no
as input).
The input validation using the OR
statement checks whether the user is either 18 years or older (age >= 18
) or has a driver’s license (hasLicense.equals("yes")
).
If either condition is true, it prints a message indicating that the user is eligible to drive. Otherwise, it informs the user that they are not eligible to drive yet.
In summary, the program checks if the user is eligible to drive based on age or the possession of a driver’s license and provides a corresponding message.
OR
Statement in Java Rules
The Java OR
statement, represented by the ||
operator, follows certain rules in terms of evaluation and behavior. Here are the key rules for the OR
statement:
- Evaluation:
- The
OR
(||
) operator evaluates the expression totrue
if at least one of the operands istrue
. - If the left operand is
true
, the right operand is not evaluated, as the overall result will betrue
regardless of the right operand.
- The
-
Short-Circuiting:
- Java uses short-circuit evaluation for the
OR
operator. If the left operand istrue
, the entire expression istrue
, and the right operand is not evaluated. - This is important when the right operand involves complex or potentially risky operations that should be avoided if unnecessary.
- Java uses short-circuit evaluation for the
-
Data Types:
- The
OR
operator can be used with boolean expressions, and the operands must be of boolean type.
- The
-
Operands Evaluation Order:
- The
OR
statement evaluates the left operand first and then the right operand if necessary. - If the left operand is
true
, the right operand is not evaluated, following the short-circuiting rule.
- The
Understanding these rules helps ensure efficient and correct use of the OR
statement, particularly when dealing with complex expressions or avoiding unnecessary computations.
OR
Statement in Java Troubleshooting
When using the OR
statement, several issues may arise, and troubleshooting becomes essential to identify and resolve these issues. Here are some possible issues and tips for troubleshooting:
-
Short-Circuiting Unexpected Behavior:
- Issue: Short-circuiting might lead to unexpected behavior if the right operand has side effects.
- Troubleshooting: Be aware of short-circuiting, especially when the right operand involves method calls or modifies variables. Ensure that the order of evaluation aligns with the intended logic.
-
Incorrect Operand Types:
- Issue: Using the
||
operator with non-boolean operands can lead to compilation errors. - Troubleshooting: Ensure that both operands are boolean expressions. If necessary, explicitly convert other types to boolean using appropriate conditions.
- Issue: Using the
-
Logical Flaws in Conditions:
- Issue: Logical errors in conditions may cause unexpected results.
- Troubleshooting: Review the conditions and their logical operators to ensure they match the intended logic. Use parentheses to clarify the order of operations if needed.
When troubleshooting issues with the OR
statement, it’s crucial to carefully review the code, consider the order of evaluation, and use appropriate parentheses to ensure the intended logic is preserved. Testing with different inputs and scenarios can also help uncover and resolve potential issues.
How to Avoid Java OR
Statement Errors
To avoid errors and ensure proper usage of the OR
statement, follow these best practices:
- Use Boolean Operands: Ensure that both operands of the
||
operator are boolean expressions. This helps prevent compilation errors. - Understand Short-Circuiting: Be aware of the short-circuiting behavior of the
||
operator. If the left operand istrue
, the right operand is not evaluated. Plan your conditions accordingly. - Parenthesize Complex Conditions: Use parentheses to explicitly indicate the order of evaluation, especially when dealing with complex conditions. This helps prevent logical errors.
By following these practices, you can minimize the chances of errors and improve the reliability of code that involves the OR
statement.
OR
Statement in Java Conclusion
In conclusion, understanding and effectively utilizing the OR
statement is essential for creating dynamic and flexible programs in Java.
We’ve explored the fundamentals of the OR
statement, its truth table, and practical applications in conditional statements, loop control, and input validation. The rules and troubleshooting tips provided offer guidance to navigate potential challenges associated with the OR
statement.
To ensure error-free code, follow best practices, such as using boolean operands, understanding short-circuiting, and testing with various inputs. By incorporating these practices into your coding habits and reviewing code during collaborative processes, you can enhance the robustness and clarity of your Java programs.
For those looking to deepen their understanding, the next learning steps could involve exploring advanced topics in Java programming or delving into other logical operators for comprehensive mastery.