Java One Line if Statement
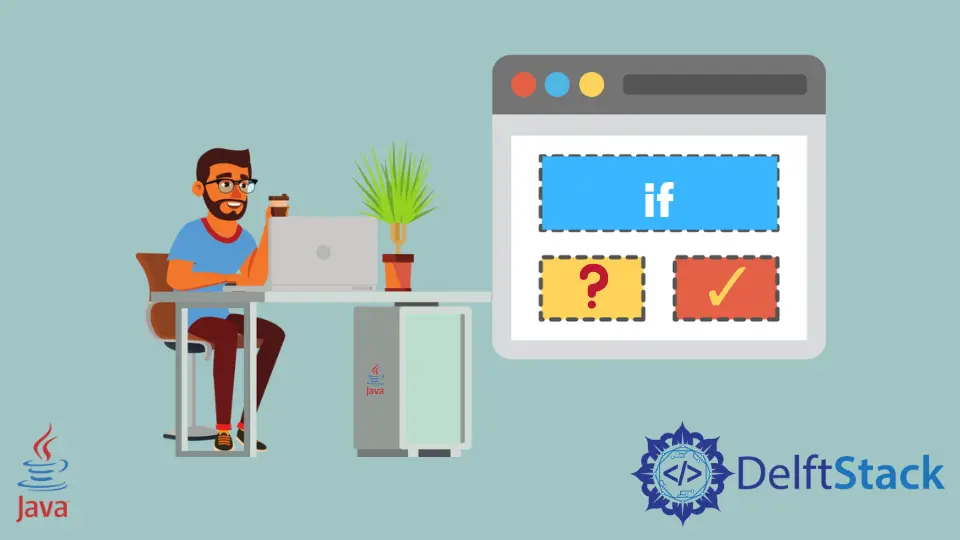
The if-else
statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else
block, which may not always be ideal, especially for concise and readable code.
Fortunately, Java provides a shorthand form called the ternary operator, which allows us to write a one-line if-else
statement.
Ternary Operator in Java
The ternary operator in Java, denoted as ? :
, provides a compact way to represent an if-else
statement. Its syntax is as follows:
condition ? expression1 : expression2;
Here, condition
is evaluated first. If condition
is true, expression1
is executed; otherwise, expression2
is executed.
Example 1: Assigning a Value Based on a Condition
public class TernaryOperatorExample {
public static void main(String[] args) {
int marks = 67;
String distinction = marks > 70 ? "Yes" : "No";
System.out.println("Has made a distinction: " + distinction);
}
}
This code uses the ternary operator to determine if a student has made a distinction based on their exam marks.
If marks
is greater than 70
, the string Yes
is assigned to the distinction
variable and the output will be:
Has made a distinction: Yes
If marks
is less than or equal to 70
, the string No
is assigned to the distinction
variable and the output will be:
Has made a distinction: No
Example 2: Printing a Message Conditionally
public class Main {
public static void main(String[] args) {
boolean isRaining = true;
System.out.println(isRaining ? "Bring an umbrella" : "No need for an umbrella");
}
}
This code defines a Boolean variable isRaining
with the value true
and uses a ternary operator to print a message based on the value of isRaining
.
If isRaining
is true
, it prints Bring an umbrella
; otherwise, it prints No need for an umbrella
.
Example 3: Returning a Value from a Method
public class Main {
public static void main(String[] args) {
int a = 10;
int b = 20;
int max = (a > b) ? a : b;
System.out.println("The maximum value is: " + max);
}
}
Output:
The maximum value is: 20
In this code, we’re assigning the maximum of a
and b
to the variable max
using a ternary operator. The maximum value is then printed to the console.
You can try it yourself by replacing the values of a
and b
with your desired values to find the maximum between them.
One Line if-else
Statement Using filter
in Java 8
Java 8 introduced streams and the filter
method, which operates similarly to an if-else
statement. It allows us to filter elements based on a condition.
Here’s its syntax:
Stream<T> filter(Predicate<? super T> predicate);
Predicate
is a functional interface that takes an argument and returns a Boolean. It’s often used with lambda expressions to define the condition for filtering.
Here’s a demonstration of a one-line if-else
-like usage using the filter
method in Java 8 streams.
Example 1:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class OneLineFilterUsage {
public static void main(String[] args) {
List<String> words = Arrays.asList("apple", "banana", "cherry", "date");
List<String> filteredWords = words.stream()
.map(word -> word.startsWith("b") ? word : "Not available")
.collect(Collectors.toList());
System.out.println("Filtered words: " + filteredWords);
}
}
Output:
Filtered words: [Not available, banana, Not available, Not available]
As we can see, this example demonstrates a one-line usage of an if-else
-like structure using the filter
method. It first defines a list of words and then applies a stream to this list.
Within the map
function, a lambda expression is used to check if each word starts with the letter b
. If a word meets this condition, it remains unchanged; otherwise, it is replaced with the string Not available
.
Finally, the resulting stream is collected back into a list using collect(Collectors.toList())
. The output shows the effect of this one-line if-else
-like usage, modifying the words based on the specified condition.
Let’s see another example.
Example 2:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Java8Streams {
public static void main(String[] args) {
List<String> stringList = Arrays.asList("1", "2");
stringList.stream().filter(string -> string.equals("1")).forEach(System.out::println);
}
}
Output:
1
This example code also showcases the use of Java 8 streams to filter and print elements from a list based on a condition. The code begins by importing necessary packages and defining a class named Java8Streams
.
Inside the main
method, a list of strings, stringList
, is created with elements 1
and 2
. The stream is then created from this list using the stream()
method.
Next, the filter
method is applied to this stream, utilizing a lambda expression as the predicate. The lambda expression checks if the string is equal to 1
.
If this condition is true, the string is allowed to pass through the filter; otherwise, it is discarded.
Finally, the forEach
method is used to iterate over the filtered stream and print each element that passed the filter. It uses a method reference System.out::println
to achieve this.
Conclusion
In summary, one-line if statements in Java offer a compact and efficient way to handle simple conditional logic. By understanding the syntax and appropriate usage, you can write concise code while maintaining readability and clarity.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn