How to Convert Long to Double in Java
-
Convert
long
todouble
Using Implicit Casting in Java -
Convert
long
todouble
Using Explicit Casting in Java -
Convert
long
todouble
Using thedoubleValue()
Method in Java -
Convert
long
todouble
Using thelongBitsToDouble()
Method in Java -
Convert
long
todouble
Using thelongBitsToDouble()
Method in Java - Conclusion
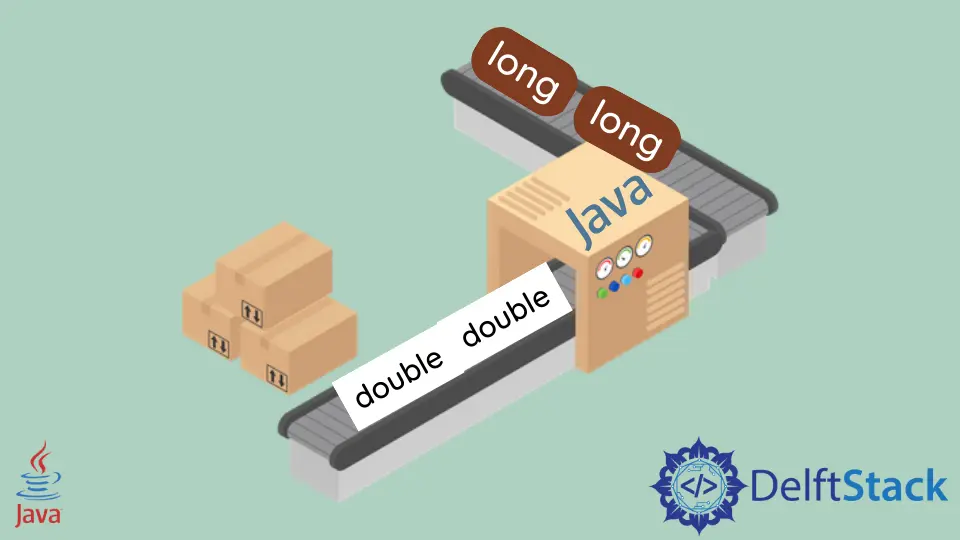
This tutorial introduces how to convert long
type to double
type in Java.
In Java, long
and double
are both used to store numerical values. The long
is used to store non-floating values, whereas the double
is used to store floating-point values, and both take the same number of bytes (16 bytes) to store data into memory.
In this article, we’ll learn to convert long
type value to double
type by using some methods such as doubleValue()
, longBitsToDouble()
, and parseDouble()
method.
Convert long
to double
Using Implicit Casting in Java
In this example, we are converting a long
type value to a double
type. Since both use the same bytes, conversion gets implemented easily, and double
values append a floating point after the long
value.
public class SimpleTesting {
public static void main(String[] args) {
long l = 97;
System.out.println("long value: " + l);
double d = l;
System.out.println("double value: " + d);
}
}
Output:
long value: 97
double value: 97.0
In this code, we declare a long
variable l
and initialize it with the value 97
. Subsequently, we use System.out.println
to display the value of the long
variable, producing the output: long value: 97
.
The program then employs implicit casting to convert the long
variable to a double
variable d
. Finally, we print the converted double
value to the console concatenated to the output, resulting in: double value: 97.0
.
The use of implicit casting in this scenario is possible because both long
and double
are numeric data types, and the Java compiler automatically handles the conversion between them.
Convert long
to double
Using Explicit Casting in Java
Although we don’t need to use explicit casting for long
to double
conversion for better code, we can use explicit casting. See the example below; we get the same result as we get in the above example.
public class SimpleTesting {
public static void main(String[] args) {
long l = 97;
System.out.println("long value: " + l);
double d = (double) l;
System.out.println("double value: " + d);
}
}
Output:
long value: 97
double value: 97.0
In this code, we initialize a long
variable l
with the value 97
. Using System.out.println
, we display the original long
value, resulting in the output long value: 97
.
Following this, we explicitly cast the long
variable to a double
using (double) l
. This explicit casting is employed to ensure a clear conversion from the integral type (long
) to a floating-point type (double
).
Finally, we print the newly obtained double
value, and the output is double value: 97.0
. The explicit casting is crucial here, as it emphasizes precision in the transformation, highlighting the conversion from a whole number to a decimal in Java.
Convert long
to double
Using the doubleValue()
Method in Java
If you have a long
object, you can simply use the doubleValue()
method of the Long
class to get a double
type value. This method does not take any argument but returns a double
after converting a long
value.
public class SimpleTesting {
public static void main(String[] args) {
Long l = new Long(97);
System.out.println("long value: " + l);
double d = l.doubleValue();
System.out.println("double value: " + d);
}
}
Output:
long value: 97
double value: 97.0
In this code, we create a Long
object l
initialized with the value 97
. Using System.out.println
, we display the Long
object’s value, resulting in the output long value: 97
.
Subsequently, we employ the doubleValue()
method on the Long
object to obtain its corresponding double
value. This method facilitates a straightforward conversion from the boxed Long
type to the primitive double
type.
Finally, we print the double
value, and the output is double value: 97.0
. This approach allows for a seamless transition from a boxed numeric type to a primitive type, demonstrating the versatility of Java’s object-oriented features in numeric conversions.
Convert long
to double
Using the longBitsToDouble()
Method in Java
We can use the longBitsToDouble()
method as well to get a double
value from a long
type. This is a static method and belongs to the Double
class.
This method actually does the binary-level conversion. So, to get results in readable form, use the doubleToRawLongBits()
method.
public class SimpleTesting {
public static void main(String[] args) {
long l = 97;
System.out.println("long value: " + l);
double d = Double.longBitsToDouble(l);
System.out.println("double value: " + d);
System.out.println(Double.doubleToRawLongBits(d));
}
}
Output:
long value: 97
double value: 4.8E-322
97
In this code, we initialize a long
variable l
with the value 97
. Using System.out.println
, we display the original long
value, resulting in the output long value: 97
.
Subsequently, we employ the longBitsToDouble()
method from the Double
class to perform a binary-level conversion, obtaining a double
value d
. We then print the converted double
value, yielding the output double value: 4.8E-322
.
Additionally, we use Double.doubleToRawLongBits(d)
to display the raw bits of the double
value, which in this case is 97
. The longBitsToDouble()
method enables us to convert the bit representation of a long
to its equivalent double
value, showcasing the binary-level precision in the conversion process.
Convert long
to double
Using the longBitsToDouble()
Method in Java
The parseDouble()
method takes a string type long
object value and returns a double
value. This is a static method of the Double
class and can only be used for string arguments.
public class SimpleTesting {
public static void main(String[] args) {
Long l = new Long(97);
System.out.println("long value: " + l);
double d = Double.parseDouble(l + "");
System.out.println("double value: " + d);
}
}
Output:
long value: 97
double value: 97.0
In this code, we create a Long
object l
initialized with the value 97
. Utilizing System.out.println
, we display the Long
object’s value, resulting in the output long value: 97
.
Subsequently, we employ the Double.parseDouble()
method to convert the string representation of the Long
object to a double
value d
. The concatenation of the Long
object with an empty string is a common technique to convert it to a string.
Finally, we print the converted double
value, and the output is double value: 97.0
. This method is useful when dealing with numeric values stored as strings, providing a convenient way to convert them into their corresponding double
representation in Java.
Conclusion
In conclusion, this tutorial delves into various methods for converting a long
type to a double
type. The implicit casting approach was demonstrated, showcasing the ease of conversion due to both types utilizing the same number of bytes.
Additionally, explicit casting was introduced as an alternative for better code clarity. The tutorial also covered the utilization of methods such as doubleValue()
, which belongs to the Long
class, offering a straightforward way to convert a Long
object to a double
type.
The use of the longBitsToDouble()
method, a binary-level conversion method in the Double
class, was explored, along with the recommendation to use doubleToRawLongBits()
for readable results. Finally, the tutorial introduced the parseDouble()
method for converting a string representation of a long
object to a double
value.
Understanding these conversion techniques empowers developers to choose the most suitable approach for their specific scenarios, ensuring accurate and efficient conversion from long
to double
in Java.