How to Convert Binary String to Int in Java
-
Convert a Binary
string
toint
in Java Using theInteger.parseInt()
Method -
Convert a Binary
string
toint
in Java Using theMath.pow()
Method -
Convert a Binary
string
toint
in Java Using thevalueOf()
Method of theBigInteger
Class - Conclusion
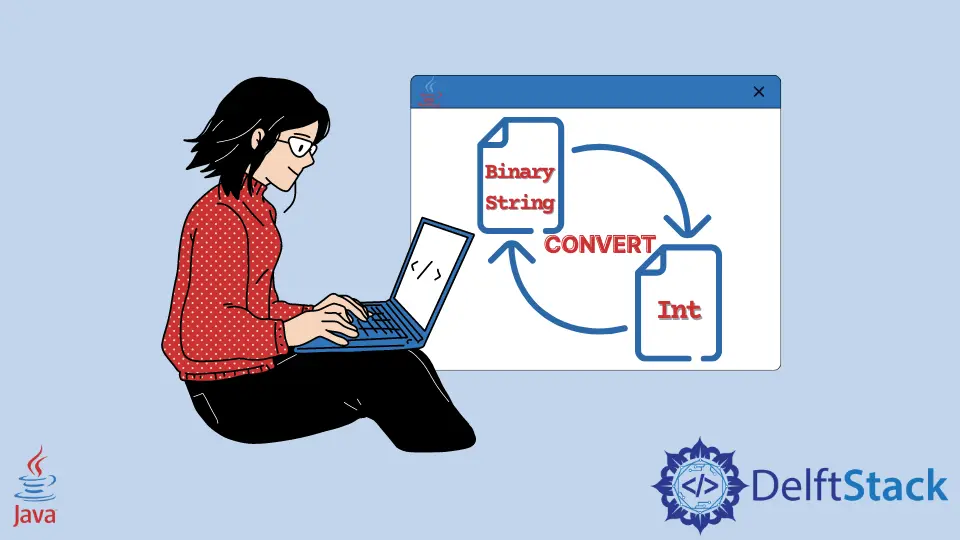
Binary, composed of the digits 0
and 1
, forms the fundamental language of computers. While machines effortlessly interpret these binary codes, deciphering them can be a challenge for humans, and this is why there are various techniques to convert binary representations into formats more readable by us.
In this tutorial, we’ll explore various methods for converting a binary string
to an int
. Our objective is to take a binary input and parse it, producing an int
that accurately represents the corresponding binary number.
Convert a Binary string
to int
in Java Using the Integer.parseInt()
Method
In Java, the Integer.parseInt()
method provides a straightforward way to convert a binary string
into an integer. This method allows us to parse binary representations by specifying the base, in this case, 2
for binary.
Basic Syntax:
int decimal = Integer.parseInt(binaryString, 2);
In the syntax, the method takes two parameters: the first parameter, binaryString
, is the string containing the binary digits that we want to parse, and the second parameter, 2
, signifies the base of the number system we are converting from.
Code:
public class BinaryStringToInt {
public static void main(String[] args) {
try {
String binaryString = "10010";
int foo = Integer.parseInt(binaryString, 2);
System.out.println(foo);
} catch (NumberFormatException e) {
System.out.println("Error: The binary string is not valid");
}
}
}
Output:
18
In this code, we aim to convert a binary string, 10010
, to its decimal equivalent using the Integer.parseInt()
method with base 2
. Within a try-catch
block, we attempt the conversion, storing the result in the variable foo
.
If the binary string is valid, we print the decimal value to the console, which in this case is 18
.
Convert a Binary string
to int
in Java Using the Math.pow()
Method
In Java, the Math.pow()
method serves as a key tool for manually converting a binary string
into an integer. This method enables us to raise the base, in this case, 2
, to the power of each binary digit’s position, facilitating the calculation of the decimal equivalent.
Code:
public class Main {
public static void main(String[] args) {
String binaryString = "101000";
double convertedDouble = 0;
for (int i = 0; i < binaryString.length(); i++) {
if (binaryString.charAt(i) == '1') {
int len = binaryString.length() - 1 - i;
convertedDouble += Math.pow(2, len);
}
}
int convertedInt = (int) convertedDouble;
System.out.println(convertedInt);
}
}
Output:
40
In this code, we are converting a binary string, 101000
, to its decimal equivalent without using built-in methods. Using a for
loop, we iterate through each character of the binary string.
If the character is '1'
, we calculate its decimal value based on its position using the Math.pow()
method. The calculated values are accumulated in the variable convertedDouble
.
Finally, we cast convertedDouble
to an integer and print the result to the console which is 40
.
Convert a Binary string
to int
in Java Using the valueOf()
Method of the BigInteger
Class
When dealing with larger binary strings that might exceed the capacity of standard data types, the valueOf()
method of the BigInteger
class becomes a valuable asset. This method allows us to effortlessly convert a binary string
into an integer, providing flexibility and precision.
Code:
import java.math.BigInteger;
public class BinaryConversion {
public static void main(String[] args) {
String binaryString = "10010";
BigInteger bigInt = new BigInteger(binaryString, 2);
int decimal = bigInt.intValue();
System.out.println(decimal);
}
}
Output:
18
In this code, we leverage the BigInteger
class to convert a binary string, 10010
, to its decimal equivalent. Using the BigInteger
constructor with the binary string and base 2
, we create a BigInteger
object named bigInt
.
To obtain the corresponding integer value, we use the intValue()
method and store the result in the variable decimal
. Finally, we print the decimal value to the console, which is 18
.
Conclusion
In conclusion, navigating the binary language of computers, composed of 0
and 1
, can be challenging for humans. To ease this, we explored methods in Java for converting binary strings to integers.
The Integer.parseInt()
method offers a straightforward solution, allowing easy conversion by specifying base 2
. For a more manual approach, leveraging the Math.pow()
method facilitates a systematic calculation of the decimal equivalent.
In cases of larger binary strings, the valueOf()
method of the BigInteger
class proves invaluable, ensuring precision even beyond standard data type capacities.
These methods offer developers flexibility and ease when working with binary data. Whether opting for simplicity, manual precision, or accommodating larger datasets, Java provides versatile tools for converting binary strings into integers.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn