How to Convert Object to Int in Java
-
Convert
Object
toint
in Java by Using Integer Wrapper Class -
Convert
Object
toint
in Java by UsingNumber
andintValue()
Function -
Convert
Object
toint
in Java by UsingInteger
andintValue()
Function -
Convert
Object
toint
in Java by UsingInteger.parseInt()
Function - Conclusion
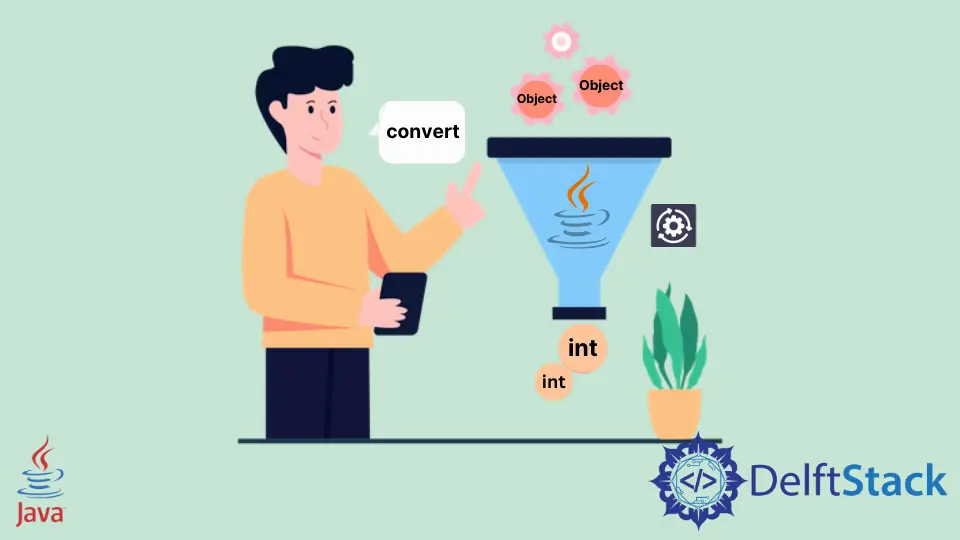
We can say the Object
class is the parent class of all classes in Java by default. In Java, we can use the int
keyword, a primitive data type, to declare variables and return integer-type values with methods.
In this article, we explored different Java methods to convert an object to an int
, as there are various scenarios where such conversions can be useful. One common use case is performing computations that can only be executed on integer values.
Additionally, these conversions can be handy when you need to return an integer value or manipulate numeric data. Suppose you are given an Object
of the Object
class, and you need to convert it to an int
.
In this article, we discussed four distinct approaches: using the Integer
wrapper class, leveraging the Number
and intValue()
function, employing the Integer
class with the intValue()
function, and the use of Integer.parseInt()
.
Convert Object
to int
in Java by Using Integer Wrapper Class
In Java, we can use the Integer
wrapper class to convert an object to an int
. This is particularly useful when we have an Object
and need to extract an integer value from it.
In the following syntax, the public static int objectToInt(Object obj)
defines a static method named objectToInt
. It accepts an Object
as a parameter and returns an int
.
Within the method, we perform the conversion by explicitly casting obj
to an Integer
, and then we assign it to an int
variable, x
.
Finally, the method returns the resulting int
value using the return
statement.
Syntax:
public static int objectToInt(Object obj) {
int x = (Integer) obj;
return x;
}
Parameter:
Object obj
: The parameter indicates that theobjectToInt
accepts anObject
. We perform explicit casting to convertobj
into anInteger
, assigning it to anint
variable,x
, and then return theint
value from the method.
Let’s look at the code below to understand how it works.
public class Main {
public static int objectToInt(Object obj) {
int x = (Integer) obj;
return x;
}
public static void main(String args[]) {
Object obj = new Object();
int value = 123;
obj = value;
int res = objectToInt(obj);
System.out.println("The converted int is " + res);
}
}
In the example, we have a Main
class that contains two methods: objectToInt
and main
.
The objectToInt
method takes an Object
as a parameter and returns an int
. We use explicit casting to convert the Object
to an int
by casting it to an Integer
and then returning the result.
We create an Object
variable obj
within the main
method and initialize it with the value 123
. We then call the objectToInt
method to convert obj
to an int
and store the result in the variable res
.
Finally, we print "The converted int is "
followed by the value stored in res
.
Output:
The converted int is 123
The output indicates that the code successfully converted an Object
containing the integer value 123
into an int
.
Convert Object
to int
in Java by Using Number
and intValue()
Function
In Java, we have an alternative method to convert an Object
to an int
by utilizing the Number
class and its intValue()
function. This method is very handy when dealing with generic objects that may contain numeric values.
In the following syntax, we define a method, objectToInt
, which takes an Object
as its parameter. This method is declared as public static
and returns an int
.
In the conversion process, we explicitly cast the obj
Object
to a Number
and then call the intValue()
function on it. This step is crucial as it extracts the int
value, making it the central part of the conversion.
Finally, we return the resulting int
value, completing the conversion process.
Syntax:
public static int objectToInt(Object obj) {
int x = ((Number) obj).intValue();
return x;
}
Parameter:
Object obj
: It specifies that theobjectToInt
method accepts anObject
as input. Within the method, we convert thisObject
into aNumber
and then extract anint
using theintValue()
function, and the method returns thisint
.
Let’s look at the code below to understand how it works.
public class Main {
public static int objectToInt(Object obj) {
int x = ((Number) obj).intValue();
return x;
}
public static void main(String args[]) {
Object obj = new Object();
int value = 123;
obj = value;
int res = objectToInt(obj);
System.out.println("The converted int is " + res);
}
}
In the example, we define a Main
class containing a method called objectToInt
. This method accepts an Object
as an input and returns an int
.
Within the objectToInt
method, we cast the Object
to a Number
and extract the integer value using intValue()
. We then assign and return this integer value.
In the main
method, we initialize an Object
(obj
) and assign an int
value of 123
to it. We call the objectToInt
method to convert obj
to an int
, and the result is printed to the console.
Output:
The converted int is 123
The output indicates that the code successfully converted an Object
containing the integer value 123
into an int
.
Convert Object
to int
in Java by Using Integer
and intValue()
Function
We can modify the previous method and convert an object to int
in Java by utilizing the Integer
class along with its intValue()
function. This method becomes particularly valuable when dealing with generic objects that may contain numeric data.
In the syntax, we’ve defined a method called objectToInt
. This method is specified as public static
, accepts an Object
as a parameter, and returns an int
.
We specifically cast the Object
as an Integer
to convert it to an int
. This casting step reflects our assumption that the Object
contains an Integer
.
Next, we call the intValue()
method on the Integer
object to retrieve the underlying int
value. To finish the conversion, we return the method’s received int
value.
Syntax:
public static int objectToInt(Object obj) {
int x = ((Integer) obj).intValue();
return x;
}
Let’s look at the code below to understand how it works.
public class Main {
public static int objectToInt(Object obj) {
int x = ((Integer) obj).intValue();
return x;
}
public static void main(String args[]) {
Object obj = new Object();
int value = 123;
obj = value;
int res = objectToInt(obj);
System.out.println("The converted int is " + res);
}
}
In the example, we have a Main
class with two vital methods: objectToInt
and main
.
The objectToInt
method is at the core of our conversion process. It’s a public static function designed to convert an Object
into an int
.
Within the objectToInt
method, we explicitly cast the Object
named obj
into an Integer
, expressing our anticipation of an Integer
value. Following this, we efficiently extract the underlying integer value using the intValue()
method.
In our main
method, we create an initially empty instance of the Object
class. Simultaneously, we declare an int
variable named value
and initialize it with 123
.
Next, we call the objectToInt
method, passing obj
as an argument. The method extracts the integer value from obj
, returning it and storing it in another int
variable named res
.
Finally, we print the result to the console.
Output:
The converted int is 123
This output confirms the success of the conversion process, displaying the resulting int
value, which, in this case, is 123
.
Convert Object
to int
in Java by Using Integer.parseInt()
Function
We can also use the Integer.parseInt()
method to convert an object to int
in Java.
In the syntax below, within the try
block, we specify obj
as the Object
we intend to convert. We should replace the ellipsis ...
with your specific Object
.
Then, we proceed by converting obj
into a String
and then performing the conversion to an int
with Integer.parseInt()
. If this conversion process fails, resulting in a NumberFormatException
, we manage it within the catch
block.
Next, inside the catch
block, we can specify how to address this situation, like throwing a different exception or defining alternative behavior for cases where the Object
doesn’t represent a valid integer, ensuring code robustness.
Syntax:
int result;
try {
Object obj = ...; // Replace with your Object containing the value
String str = obj.toString();
result = Integer.parseInt(str);
} catch (NumberFormatException e) {
// Handle the case where the Object does not represent a valid integer
// You can throw an exception or define an alternative behavior.
}
Parameter:
Object obj
: This is theObject
that you want to convert to anint
. Replace it with your actualObject
containing the value you want to convert.
Let’s look at the code below to understand how it works.
public class Main {
public static int objectToInt(Object obj) {
int x = Integer.parseInt(obj.toString());
return x;
}
public static void main(String args[]) {
Object obj = new Object();
int value = 123;
obj = value;
int res = objectToInt(obj);
System.out.println("The converted int is " + res);
}
}
In the code, we have a method called objectToInt
that takes an Object
as input, converts it to a String
, and then parses it to an int
. In the main
method, we construct an Object
named obj
initially containing a generic object.
We then assign the integer value 123
to obj
. We call the objectToInt
method with obj
as an argument, and the resulting integer is stored in res
.
The program prints "The converted int is"
followed by the value of res
.
Output:
The converted int is 123
This output confirms the success of the conversion process, displaying the resulting int
value, which, in this case, is 123
.
Conclusion
In this article, the discussed methods provide flexibility for converting an Object
to an int
. These conversion methods are valuable tools that enhance the versatility of working with objects and numeric data in Java, allowing you to effectively bridge the gap between generic objects and integer values.
The first approach, using the Integer
wrapper class, is particularly straightforward and recommended for its simplicity. However, you can choose the most suitable approach according to the particular needs of your Java application.