How to Prevent Orphaned Case Error in Java
-
the
switch-case
in Java -
Understanding
orphaned cases
in Java -
Preventing
orphaned cases
in Java -
Best Practices for Preventing
orphaned cases
in Java - Conclusion
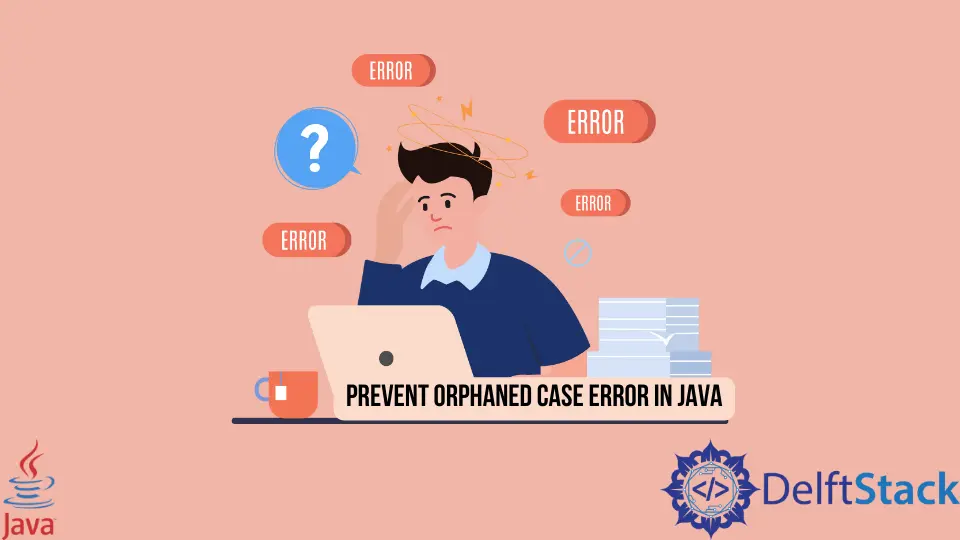
In the vast landscape of Java programming, the switch
statement stands as a powerful tool for directing program flow based on the value of an expression
. This article aims to teach about switch-case
, causes of orphaned cases
, and methods to prevent them in Java with best practices.
the switch-case
in Java
The switch-case
in Java provides a way to execute different blocks of code based on the value of an expression
. The switch
statement evaluates the expression
and matches it against various cases
.
Each case
represents a specific value or range of values that the expression
can take. When a match is found, the corresponding block of code associated with that case
is executed.
The syntax of a switch-case
in Java is as follows:
switch (expression) {
case value1:
// code block 1
break;
case value2:
// code block 2
break;
// additional cases as needed
default:
// default code block
}
In the syntax of a switch-case
in Java, the expression
being evaluated determines the flow of execution. Each case
within the switch
block represents a potential value or range of values that the expression
can assume.
The break
statement is employed to conclude the switch
statement and exit the switch
block once a matching case
is found. Additionally, the default case
, which is optional, is executed when none of the specified cases
correspond to the value of the expression
.
The switch-case
offers a cleaner alternative to using multiple nested if-else
statements, making the code more readable and easier to maintain. Additionally, the default case
can be used to handle scenarios where none of the specified cases
match the expression
’s value.
Overall, the switch-case
provides a structured approach to control flow in Java programs.
Understanding orphaned cases
in Java
In Java, an orphaned case
refers to a situation where a case
statement within a switch
block lacks corresponding logic or has been left incomplete. It’s important to solve this error because orphaned cases
can lead to unexpected behavior or bugs in your code.
Ensuring that all cases
within a switch
statement are properly handled helps maintain code reliability and prevents potential issues during execution.
Three common causes of orphaned cases
include incomplete refactoring, typographical errors, and incomplete implementation. Let’s explore each cause in detail:
Cause | Description |
---|---|
Incomplete refactoring | If you’ve refactored your code and removed the logic associated with a case but forgot to remove the case itself, it can result in an orphaned case error. |
Typographical errors | Sometimes, typos or mistakes in code editing can result in cases being orphaned. For example, accidentally deleting or commenting out the associated code. |
Incomplete implementation | During the initial coding phase, you might have added placeholder cases that you forgot to implement. These cases remain orphaned until you implement their functionality. |
orphaned cases
in Java refer to instances where a case
within a switch
statement lacks corresponding logic or has been left incomplete. This can occur due to various reasons, such as incomplete refactoring, typographical errors, or incomplete implementation.
Incomplete refactoring happens when code logic is removed but the associated case
is not. Typographical errors occur when mistakes in code editing lead to cases
being unintentionally orphaned.
Incomplete implementation arises when placeholder cases
are added during coding but not properly implemented. It’s important to address orphaned cases
as they can result in unexpected behavior or bugs in your code.
Regular code review and attention to detail can help prevent and resolve orphaned cases
effectively.
Preventing orphaned cases
in Java
In Java programming, switch
statements provide a concise way to execute different blocks of code based on the value of an expression
. However, ensuring that all cases
within a switch
statement are properly handled is crucial to prevent orphaned cases
, which can lead to bugs and unexpected behavior in your code.
In this section, we’ll explore how to use correct methods to prevent orphaned cases
in Java.
Let’s consider a scenario where we have a Java class named DayOfWeek
with a main
method. Inside the main
method, we declare an integer variable day
representing the day of the week.
We then use a switch
statement to determine the corresponding day based on the value of day
.
public class DayOfWeek {
public static void main(String[] args) {
int day = 4; // Change this value to test different cases
// Typographical error - Switch (never use upper cases when dealing with switch cases
switch (day) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
case 3:
System.out.println("Tuesday");
break;
// Incomplete refactoring - case 4 is left orphaned
// Typographical error - case 5 is mistyped as 'cas'
// case 4:
// Missing code for Wednesday
// cas 5:
// Incomplete implementation - case 5 is not implemented
case 4:
System.out.println("Wednesday");
break;
case 5:
System.out.println("Thursday");
break;
case 6:
System.out.println("Friday");
break;
case 7:
System.out.println("Saturday");
break;
default:
System.out.println("Invalid day");
}
}
}
In the provided example, the DayOfWeek
class contains a main
method where we declare an integer variable day
representing the day of the week. We initialize day
with a value of 4 for demonstration purposes.
However, upon examining the switch
statement, we notice several issues. Firstly, the switch
statement itself is written in upper case, which is not conventional.
switch statements
in Java are typically written in lowercase. This typographical error could lead to confusion but does not directly cause orphaned cases
.
Secondly, the case
for Wednesday
(day 4) is initially commented out, indicating incomplete refactoring. This results in a potential orphaned case
where the logic for Wednesday
is missing.
Thirdly, the case
for Thursday
(day 5) is mistyped as cas
instead of case
. This typographical error renders the case
unrecognized and contributes to the orphaned case
problem.
Lastly, although case 4
(Wednesday
) is uncommented, there is no associated logic, leaving it as an orphaned case
.
To prevent orphaned cases
in Java switch
statements, it’s essential to adhere to best practices and thoroughly review your code. This includes ensuring proper casing, addressing incomplete refactoring, fixing typographical errors, and implementing all necessary cases
.
By following the correct methods, you can maintain clean and reliable code, reducing the likelihood of encountering unexpected behavior or bugs.
When executing the provided code with day
set to 4
, the output will be:
This output demonstrates that the issue of incomplete implementation for Wednesday
(day 4) has been resolved, and the switch
statement now correctly handles all cases
.
Best Practices for Preventing orphaned cases
in Java
Consistent Coding Style
Ensure that your code follows a consistent coding style, including the casing of keywords like switch
and case
. Stick to lowercase for these keywords to maintain readability and avoid potential typographical errors.
Comprehensive Code Review
Regularly conduct thorough code reviews to catch any instances of incomplete refactoring, typographical errors, or incomplete implementation. Encourage team members to scrutinize switch
statements carefully for any orphaned cases
.
Use Enumerations
Consider using enumerations to represent finite sets of constants, especially when dealing with switch
statements that involve a limited number of cases
. Enumerations provide compile-time type safety and can help prevent orphaned cases
by explicitly defining all possible values.
default case
Handling
Always include a default case
in your switch
statements to handle unexpected input or cases
that are not explicitly covered. This ensures that your code behaves in a predictable manner even when encountering unforeseen scenarios.
Unit Testing
Develop comprehensive unit tests that cover all branches of your switch
statements. Write test cases to specifically target each case
and ensure that the associated logic is correctly implemented.
This helps identify any orphaned cases
and verifies that the switch
statement behaves as expected under different conditions.
Static Code Analysis
Utilize static code analysis tools or IDE plugins that can detect potential issues, such as orphaned cases
, during development. These tools can provide automated suggestions for resolution and help maintain code quality and consistency across your project.
Clear Documentation
Document your code thoroughly, including explanations for each case
within switch
statements. Clearly state the purpose and expected behavior of each case
to avoid confusion and reduce the likelihood of orphaned cases
due to misunderstanding or oversight.
Regular Code Refactoring
Regularly refactor your code to remove redundant or unnecessary cases
from switch
statements. As your codebase evolves, certain cases
may become obsolete or redundant, leading to potential orphaned cases
if not properly addressed.
Team Collaboration
Encourage collaboration and communication within your development team to foster a culture of shared responsibility and accountability. Encourage team members to raise awareness of any potential orphaned cases
they encounter and work together to address them promptly.
Conclusion
Java switch cases
provide a structured approach to control flow, allowing for the execution of different code blocks based on the value of an expression
. However, orphaned cases
can occur due to incomplete refactoring, typographical errors, or incomplete implementation, leading to unexpected behavior in code execution.
To prevent orphaned cases
, it’s essential to adhere to correct methods such as ensuring consistent coding style, conducting comprehensive code reviews, using enumerations, handling default cases
, writing unit tests, utilizing static code analysis, documenting code thoroughly, and regularly refactoring code.
By following these best practices, developers can maintain clean and reliable code, minimizing the risk of encountering orphaned cases
and ensuring the robustness of their Java applications.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack