Byte Buffer Class in Java
-
ByteBuffer
Class in Java -
Operational Categories of
ByteBuffer
Class in Java - Methods of Byte Buffer Class in Java
- Implementation of Byte Buffer in Java
-
Implement the
getChar
Method of Byte Buffer Class in Java
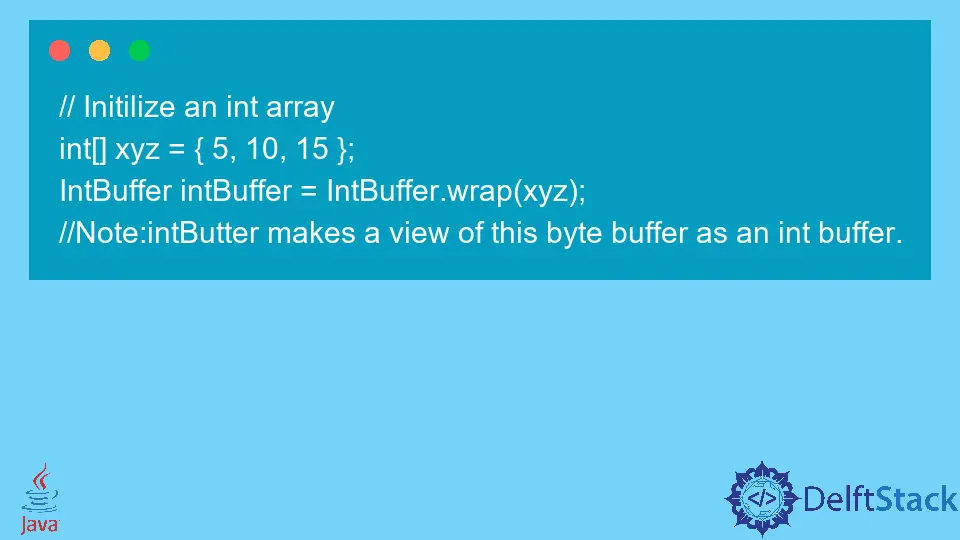
This article will help you understand how a Java Virtual Machine deals with a ByteBuffer
class. We will also signify its scope and list out its major parameters.
Finally, we will run two Java programs to demonstrate what we discussed.
ByteBuffer
Class in Java
A ByteBuffer
class is used to contain a series of integer values. These integer values are used in the I/O operations.
It is a buffer that helps transfer bytes from a source to a destination. Also, it provides abstractions such as current position, limit, capacity, and so on, besides storage like a buffer array.
Example1
and Example2
.We use the first example to demonstrate how to allocate a ByteBuffer
and use its objects. We will mainly use the getChar()
and rewind()
methods in the second example.
Please keep reading to learn more.
Operational Categories of ByteBuffer
Class in Java
- We can use
get
andput
methods (absolute and relative) to read and write single bytes. - We can also use (relative
bulk get
methods) to transfer adjoining sequence data of bytes from this buffer to an array. - We can also use methods for compacting, duplicating, and slicing a byte buffer (Optional).
- Absolute and relative
get
andput
methods for reading and writing values of other primitive types, converting them to and from byte sequences in particular byte order.
Hierarchy of ByteBuffer
Class:
java.lang.Object> java.nio.Buffer>java.nio.ByteBuffer`
A byte buffer can be direct or indirect, also referred to as non-direct. The Java Virtual Machine (JVM) will make every if we have a direct byte buffer.
Attempt to perform native I/O operations on it directly. In other words, JVM tries to avoid copying the buffer’s content to (or from) an intermediate buffer before (or after) each incantation of a native I/O operation of the operating system.
With two simple methods, we can create byte buffers:
-
allocate()
- We can allocate space for the buffer’s content.The following code block shows you how to create an empty byte buffer with a 20-byte capacity.
Syntax:
ByteBuffer xyz = ByteBuffer.allocate(20);
-
wrap()
- wrap it into an existing byte array of a buffer.Syntax:
// Initilize an int array int[] xyz = {5, 10, 15}; IntBuffer intBuffer = IntBuffer.wrap(xyz); // Note:intButter makes a view of this byte buffer as an int buffer.
Methods of Byte Buffer Class in Java
There are more methods that this class may contain. However, we will highlight only those that we think are necessary.
Secondly, we will not use each method in the table below for our implementation. We aim to familiarize you with some of the most significant methods and their use.
If you want to read more: Byte Buffer Class Oracle Docs.
S.N | Method Name | Summary |
---|---|---|
1 | put(byte a) |
It reserves a fresh byte buffer. |
2 | get() |
A relative get method. |
3 | allocate(int capacity) |
Reserves a new byte buffer. |
4 | order() |
Gives the current hash code of this buffer. |
5 | isDirect() |
Inform if or not this byte buffer is direct. |
6 | hashArray() |
Hash array tells if or not an available byte array backs this buffer. |
7 | getInt() |
For reading an int value. |
8 | compact() |
Compacts this buffer |
9 | asIntBuffer() |
This views this byte buffer as an int buffer. |
10 | allocateDirect(int capacity) |
It allocates a new direct byte buffer. |
11 | putInt(int value) |
It is a relative put method. |
12 | put(int, byte ) |
This is an absolute put method. |
13 | getChar() |
It is used to obtain a method for reading a character value. |
Implementation of Byte Buffer in Java
The following program will not show you an example for each method given in the table. But, you will cover some of the most common ones.
Alternatively, you may also refer to the official documentation.
Examples:
-
Set Capacity
ByteBuffer bfr = ByteBuffer.allocate(20);
-
Get the byte buffer’s capacity.
int capacity = bfr.capacity();
-
Set the position using absolute
put(int, byte)
.Note: This method does not affect the position.
// position=2 bfr.put(2, (byte) 2xFF);
-
Set the position to 10
bfr.position(10);
-
You can also use the relative
put(byte)
mentioned in the table.bfr.put((byte) 2xFF); // position 2
-
If you want to get the new position
int newposition = bfr.position(40);
-
You can get the remaining byte count
int remainingByteCount = bfr.remaining();
-
Setting the Limit
bfr.limit(10);
Implementation:
We will demonstrate the byte buffer class in this program. First, we will create a byte buffer and its object and allocate it a size.
Then, we will convert the int
data type to the byte by typecasting using the PuntInt()
function.
Code:
package bytebuffer.delftstack.com.util;
/*We will demonstrate the byte buffer class in this program.
*First of all, we will create a byte buffer and its object and allocate it a size.
*Then, we will convert the int data type to the byte by typecasting with the help of PuntInt()
*function */
import java.nio.*;
import java.util.*;
// main class
public class Example1 {
// main function
public static void main(String[] args) {
// capacity declaration
int allocCapac = 6;
// Creating the ByteBuffer
try {
// creating object of ByteBuffer
// and allocating size capacity
ByteBuffer createOBJ = ByteBuffer.allocate(allocCapac);
// putting the int to byte typecast value
// in ByteBuffer using putInt() method
createOBJ.put((byte) 20);
createOBJ.put((byte) 40);
createOBJ.put((byte) 60);
createOBJ.put((byte) 80);
createOBJ.put((byte) 100);
createOBJ.put((byte) 120);
createOBJ.rewind();
// We will print the byter buffer now
System.out.println("The byte buffer: " + Arrays.toString(createOBJ.array()));
}
// catch exception for error
catch (IllegalArgumentException e) {
System.out.println("IllegalArgumentException catched");
} catch (ReadOnlyBufferException e) {
System.out.println("ReadOnlyBufferException catched");
}
}
}
// class
Output:
The byte buffer: [20, 40, 60, 80, 100, 120]
Implement the getChar
Method of Byte Buffer Class in Java
We will use string instead of int
in this code block like in the previous program. First of all, we declare the capacity of the byte buffer to be 100
.
Then, we create its object, put the string instead of the int
value, and allocate it with size. After that, we will use rewind()
to rewind this buffer and in the while
loop and finally apply the getChar
function.
Please check out the following code block to learn more.
package bytebuffer.delftstack.com.util;
/*In this code block, we will use string instead of int like the previous program.
First of all, we declare the capacity of the byte buffer to `100`.
Then, we create its object, put the string instead of the int value, and allocate it with size.
After that, we will use `rewind()` to rewind this buffer and in the while loop and finally apply the
getChar function. Please check out the following code block to learn more:*/
import java.nio.ByteBuffer;
public class Example2 {
public static void main(String[] args) {
// Declaring the capacity of the ByteBuffer
int capacity = 100;
// Creating the ByteBuffer
// creating object of ByteBuffer
// and allocating size capacity
ByteBuffer bufferOBJ = ByteBuffer.allocate(capacity);
// putting the string in the bytebuffer
bufferOBJ.asCharBuffer().put("JAVA");
// rewind the Bytebuffer
bufferOBJ.rewind(); // The position is set to zero and the mark isdiscarded.
// Declaring the variable
char charr;
// print the ByteBuffer
System.out.println("This is the default byte buffer: ");
while ((charr = bufferOBJ.getChar()) != 0) System.out.print(charr + "");
// rewind the Bytebuffer
bufferOBJ.rewind();
// Reads the char at this buffer's current position
// using getChar() method
char FrstVal = bufferOBJ.getChar();
// print the char FrstVal
System.out.println("\n\n The first byte value is : " + FrstVal);
// Reads the char at this buffer's next position
// using getChar() method
char NXTval = bufferOBJ.getChar();
// print the char FrstVal
System.out.print("The next byte value is : " + NXTval);
}
}
Output:
This is the default byte buffer:
JAVA
The first byte value is : J
The next byte value is : A
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn